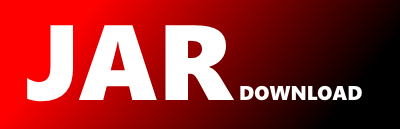
org.hibernate.engine.spi.PersistenceContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hibernate-core Show documentation
Show all versions of hibernate-core Show documentation
JPMS Module-Info's for a few of the Jakarta Libraries just until they add them in themselves
/*
* Hibernate, Relational Persistence for Idiomatic Java
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later.
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate.engine.spi;
import java.io.Serializable;
import java.util.Collection;
import java.util.HashSet;
import java.util.Iterator;
import java.util.Map;
import java.util.function.BiConsumer;
import java.util.function.Supplier;
import org.hibernate.HibernateException;
import org.hibernate.LockMode;
import org.hibernate.MappingException;
import org.hibernate.collection.spi.PersistentCollection;
import org.hibernate.engine.loading.internal.LoadContexts;
import org.hibernate.internal.util.MarkerObject;
import org.hibernate.persister.collection.CollectionPersister;
import org.hibernate.persister.entity.EntityPersister;
/**
* Represents the state of "stuff" Hibernate is tracking, including (not exhaustive):
*
* - entities
* - collections
* - snapshots
* - proxies
*
*
* Often referred to as the "first level cache".
*
* @author Gavin King
* @author Steve Ebersole
*/
@SuppressWarnings( {"JavaDoc"})
public interface PersistenceContext {
/**
* Marker object used to indicate (via reference checking) that no row was returned.
*/
Object NO_ROW = new MarkerObject( "NO_ROW" );
@SuppressWarnings( {"UnusedDeclaration"})
boolean isStateless();
/**
* Get the session to which this persistence context is bound.
*
* @return The session.
*/
SharedSessionContractImplementor getSession();
/**
* Retrieve this persistence context's managed load context.
*
* @return The load context
*/
LoadContexts getLoadContexts();
/**
* Add a collection which has no owner loaded
*
* @param key The collection key under which to add the collection
* @param collection The collection to add
*/
void addUnownedCollection(CollectionKey key, PersistentCollection collection);
/**
* Take ownership of a previously unowned collection, if one. This method returns {@code null} if no such
* collection was previously added () or was previously removed.
*
* This should indicate the owner is being loaded and we are ready to "link" them.
*
* @param key The collection key for which to locate a collection collection
*
* @return The unowned collection, or {@code null}
*/
PersistentCollection useUnownedCollection(CollectionKey key);
/**
* Get the {@link BatchFetchQueue}, instantiating one if necessary.
*
* @return The batch fetch queue in effect for this persistence context
*/
BatchFetchQueue getBatchFetchQueue();
/**
* Clear the state of the persistence context
*/
void clear();
/**
* @return false if we know for certain that all the entities are read-only
*/
@SuppressWarnings( {"UnusedDeclaration"})
boolean hasNonReadOnlyEntities();
/**
* Set the status of an entry
*
* @param entry The entry for which to set the status
* @param status The new status
*/
void setEntryStatus(EntityEntry entry, Status status);
/**
* Called after transactions end
*/
void afterTransactionCompletion();
/**
* Get the current state of the entity as known to the underlying database, or null if there is no
* corresponding row
*
* @param id The identifier of the entity for which to grab a snapshot
* @param persister The persister of the entity.
*
* @return The entity's (non-cached) snapshot
*
* @see #getCachedDatabaseSnapshot
*/
Object[] getDatabaseSnapshot(Serializable id, EntityPersister persister);
/**
* Retrieve the cached database snapshot for the requested entity key.
*
* This differs from {@link #getDatabaseSnapshot} in two important respects:
* - no snapshot is obtained from the database if not already cached
* - an entry of {@link #NO_ROW} here is interpreted as an exception
*
* @param key The entity key for which to retrieve the cached snapshot
* @return The cached snapshot
* @throws IllegalStateException if the cached snapshot was == {@link #NO_ROW}.
*/
Object[] getCachedDatabaseSnapshot(EntityKey key);
/**
* Get the values of the natural id fields as known to the underlying database, or null if the entity has no
* natural id or there is no corresponding row.
*
* @param id The identifier of the entity for which to grab a snapshot
* @param persister The persister of the entity.
*
* @return The current (non-cached) snapshot of the entity's natural id state.
*/
Object[] getNaturalIdSnapshot(Serializable id, EntityPersister persister);
/**
* Add a canonical mapping from entity key to entity instance
*
* @param key The key under which to add an entity
* @param entity The entity instance to add
*/
void addEntity(EntityKey key, Object entity);
/**
* Get the entity instance associated with the given key
*
* @param key The key under which to look for an entity
*
* @return The matching entity, or {@code null}
*/
Object getEntity(EntityKey key);
/**
* Is there an entity with the given key in the persistence context
*
* @param key The key under which to look for an entity
*
* @return {@code true} indicates an entity was found; otherwise {@code false}
*/
boolean containsEntity(EntityKey key);
/**
* Remove an entity. Also clears up all other state associated with the entity aside from the {@link EntityEntry}
*
* @param key The key whose matching entity should be removed
*
* @return The matching entity
*/
Object removeEntity(EntityKey key);
/**
* Add an entity to the cache by unique key
*
* @param euk The unique (non-primary) key under which to add an entity
* @param entity The entity instance
*/
void addEntity(EntityUniqueKey euk, Object entity);
/**
* Get an entity cached by unique key
*
* @param euk The unique (non-primary) key under which to look for an entity
*
* @return The located entity
*/
Object getEntity(EntityUniqueKey euk);
/**
* Retrieve the {@link EntityEntry} representation of the given entity.
*
* @param entity The entity instance for which to locate the corresponding entry
* @return The entry
*/
EntityEntry getEntry(Object entity);
/**
* Remove an entity entry from the session cache
*
* @param entity The entity instance for which to remove the corresponding entry
* @return The matching entry
*/
EntityEntry removeEntry(Object entity);
/**
* Is there an {@link EntityEntry} registration for this entity instance?
*
* @param entity The entity instance for which to check for an entry
*
* @return {@code true} indicates a matching entry was found.
*/
boolean isEntryFor(Object entity);
/**
* Get the collection entry for a persistent collection
*
* @param coll The persistent collection instance for which to locate the collection entry
*
* @return The matching collection entry
*/
CollectionEntry getCollectionEntry(PersistentCollection coll);
/**
* Adds an entity to the internal caches.
*/
EntityEntry addEntity(
final Object entity,
final Status status,
final Object[] loadedState,
final EntityKey entityKey,
final Object version,
final LockMode lockMode,
final boolean existsInDatabase,
final EntityPersister persister,
final boolean disableVersionIncrement);
/**
* Generates an appropriate EntityEntry instance and adds it
* to the event source's internal caches.
*/
EntityEntry addEntry(
final Object entity,
final Status status,
final Object[] loadedState,
final Object rowId,
final Serializable id,
final Object version,
final LockMode lockMode,
final boolean existsInDatabase,
final EntityPersister persister,
final boolean disableVersionIncrement);
/**
* Is the given collection associated with this persistence context?
*/
boolean containsCollection(PersistentCollection collection);
/**
* Is the given proxy associated with this persistence context?
*/
boolean containsProxy(Object proxy);
/**
* Takes the given object and, if it represents a proxy, reassociates it with this event source.
*
* @param value The possible proxy to be reassociated.
* @return Whether the passed value represented an actual proxy which got initialized.
* @throws MappingException
*/
boolean reassociateIfUninitializedProxy(Object value) throws MappingException;
/**
* If a deleted entity instance is re-saved, and it has a proxy, we need to
* reset the identifier of the proxy
*/
void reassociateProxy(Object value, Serializable id) throws MappingException;
/**
* Get the entity instance underlying the given proxy, throwing
* an exception if the proxy is uninitialized. If the given object
* is not a proxy, simply return the argument.
*/
Object unproxy(Object maybeProxy) throws HibernateException;
/**
* Possibly unproxy the given reference and reassociate it with the current session.
*
* @param maybeProxy The reference to be unproxied if it currently represents a proxy.
* @return The unproxied instance.
* @throws HibernateException
*/
Object unproxyAndReassociate(Object maybeProxy) throws HibernateException;
/**
* Attempts to check whether the given key represents an entity already loaded within the
* current session.
*
* @param object The entity reference against which to perform the uniqueness check.
*
* @throws HibernateException
*/
void checkUniqueness(EntityKey key, Object object) throws HibernateException;
/**
* If the existing proxy is insufficiently "narrow" (derived), instantiate a new proxy
* and overwrite the registration of the old one. This breaks == and occurs only for
* "class" proxies rather than "interface" proxies. Also init the proxy to point to
* the given target implementation if necessary.
*
* @param proxy The proxy instance to be narrowed.
* @param persister The persister for the proxied entity.
* @param key The internal cache key for the proxied entity.
* @param object (optional) the actual proxied entity instance.
* @return An appropriately narrowed instance.
* @throws HibernateException
*/
Object narrowProxy(Object proxy, EntityPersister persister, EntityKey key, Object object)
throws HibernateException;
/**
* Return the existing proxy associated with the given EntityKey, or the
* third argument (the entity associated with the key) if no proxy exists. Init
* the proxy to the target implementation, if necessary.
*/
Object proxyFor(EntityPersister persister, EntityKey key, Object impl)
throws HibernateException;
/**
* Return the existing proxy associated with the given EntityKey, or the
* argument (the entity associated with the key) if no proxy exists.
* (slower than the form above)
*/
Object proxyFor(Object impl) throws HibernateException;
/**
* Cross between {@link #addEntity(EntityKey, Object)} and {@link #addProxy(EntityKey, Object)}
* for use with enhancement-as-proxy
*/
void addEnhancedProxy(EntityKey key, PersistentAttributeInterceptable entity);
/**
* Get the entity that owns this persistent collection
*/
Object getCollectionOwner(Serializable key, CollectionPersister collectionPersister)
throws MappingException;
/**
* Get the entity that owned this persistent collection when it was loaded
*
* @param collection The persistent collection
* @return the owner if its entity ID is available from the collection's loaded key
* and the owner entity is in the persistence context; otherwise, returns null
*/
Object getLoadedCollectionOwnerOrNull(PersistentCollection collection);
/**
* Get the ID for the entity that owned this persistent collection when it was loaded
*
* @param collection The persistent collection
* @return the owner ID if available from the collection's loaded key; otherwise, returns null
*/
Serializable getLoadedCollectionOwnerIdOrNull(PersistentCollection collection);
/**
* add a collection we just loaded up (still needs initializing)
*/
void addUninitializedCollection(CollectionPersister persister,
PersistentCollection collection, Serializable id);
/**
* add a detached uninitialized collection
*/
void addUninitializedDetachedCollection(CollectionPersister persister,
PersistentCollection collection);
/**
* Add a new collection (ie. a newly created one, just instantiated by the
* application, with no database state or snapshot)
* @param collection The collection to be associated with the persistence context
*/
void addNewCollection(CollectionPersister persister, PersistentCollection collection)
throws HibernateException;
/**
* add an (initialized) collection that was created by another session and passed
* into update() (ie. one with a snapshot and existing state on the database)
*/
void addInitializedDetachedCollection(CollectionPersister collectionPersister,
PersistentCollection collection) throws HibernateException;
/**
* add a collection we just pulled out of the cache (does not need initializing)
*/
CollectionEntry addInitializedCollection(CollectionPersister persister,
PersistentCollection collection, Serializable id) throws HibernateException;
/**
* Get the collection instance associated with the CollectionKey
*/
PersistentCollection getCollection(CollectionKey collectionKey);
/**
* Register a collection for non-lazy loading at the end of the
* two-phase load
*/
void addNonLazyCollection(PersistentCollection collection);
/**
* Force initialization of all non-lazy collections encountered during
* the current two-phase load (actually, this is a no-op, unless this
* is the "outermost" load)
*/
void initializeNonLazyCollections() throws HibernateException;
/**
* Get the PersistentCollection object for an array
*/
PersistentCollection getCollectionHolder(Object array);
/**
* Register a PersistentCollection object for an array.
* Associates a holder with an array - MUST be called after loading
* array, since the array instance is not created until endLoad().
*/
void addCollectionHolder(PersistentCollection holder);
/**
* Remove the mapping of collection to holder during eviction
* of the owning entity
*/
PersistentCollection removeCollectionHolder(Object array);
/**
* Get the snapshot of the pre-flush collection state
*/
Serializable getSnapshot(PersistentCollection coll);
/**
* Get the collection entry for a collection passed to filter,
* which might be a collection wrapper, an array, or an unwrapped
* collection. Return null if there is no entry.
*/
CollectionEntry getCollectionEntryOrNull(Object collection);
/**
* Get an existing proxy by key
*/
Object getProxy(EntityKey key);
/**
* Add a proxy to the session cache
*/
void addProxy(EntityKey key, Object proxy);
/**
* Remove a proxy from the session cache.
*
* Additionally, ensure that any load optimization references
* such as batch or subselect loading get cleaned up as well.
*
* @param key The key of the entity proxy to be removed
* @return The proxy reference.
*/
Object removeProxy(EntityKey key);
/**
* Retrieve the set of EntityKeys representing nullifiable references
* @deprecated Use {@link #containsNullifiableEntityKey(Supplier)} or {@link #registerNullifiableEntityKey(EntityKey)} or {@link #isNullifiableEntityKeysEmpty()}
*/
@Deprecated
HashSet getNullifiableEntityKeys();
/**
* Get the mapping from key value to entity instance
* @deprecated this will be removed: it provides too wide access, making it hard to optimise the internals
* for specific access needs. Consider using #iterateEntities instead.
*/
@Deprecated
Map getEntitiesByKey();
/**
* Provides access to the entity/EntityEntry combos associated with the persistence context in a manner that
* is safe from reentrant access. Specifically, it is safe from additions/removals while iterating.
*/
Map.Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy