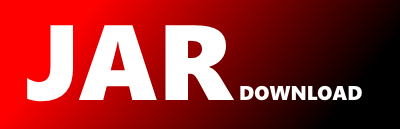
org.hibernate.query.criteria.HibernateCriteriaBuilder Maven / Gradle / Ivy
/*
* Hibernate, Relational Persistence for Idiomatic Java
*
* License: GNU Lesser General Public License (LGPL), version 2.1 or later.
* See the lgpl.txt file in the root directory or .
*/
package org.hibernate.query.criteria;
import java.util.Map;
import jakarta.persistence.criteria.CriteriaBuilder;
import jakarta.persistence.criteria.Expression;
import jakarta.persistence.criteria.Predicate;
/**
* Hibernate extensions to the JPA CriteriaBuilder. Currently there are no extensions; these are coming in 6.0
*
* @author Steve Ebersole
*/
public interface HibernateCriteriaBuilder extends CriteriaBuilder {
/**
* Create a predicate that tests whether a Map is empty.
*
* NOTE : Due to type-erasure we cannot name this the same as
* {@link CriteriaBuilder#isEmpty}
*
*
* @param mapExpression The expression resolving to a Map which we
* want to check for emptiness
*
* @return is-empty predicate
*/
> Predicate isMapEmpty(Expression mapExpression);
/**
* Create a predicate that tests whether a Map is
* not empty.
*
* NOTE : Due to type-erasure we cannot name this the same as
* {@link CriteriaBuilder#isNotEmpty}
*
* @param mapExpression The expression resolving to a Map which we
* want to check for non-emptiness
*
* @return is-not-empty predicate
*/
> Predicate isMapNotEmpty(Expression mapExpression);
/**
* Create an expression that tests the size of a map.
*
* NOTE : Due to type-erasure we cannot name this the same as
* {@link CriteriaBuilder#size}
*
* @param mapExpression The expression resolving to a Map for which we
* want to know the size
*
* @return size expression
*/
> Expression mapSize(Expression mapExpression);
/**
* Create an expression that tests the size of a map.
*
* @param map The Map for which we want to know the size
*
* @return size expression
*/
> Expression mapSize(M map);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy