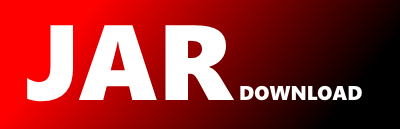
org.fusesource.jansi.internal.CLibrary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of log4j-core Show documentation
Show all versions of log4j-core Show documentation
The Apache Log4j Implementation
/*
* Copyright (C) 2009-2017 the original author(s).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.fusesource.jansi.internal;
/**
* Interface to access some low level POSIX functions, loaded by
* HawtJNI Runtime
* as jansi
library.
*
* @author Hiram Chirino
* @see JansiLoader
*/
@SuppressWarnings("unused")
public class CLibrary {
static {
JansiLoader.initialize();
init();
}
private static native void init();
public static int STDOUT_FILENO = 1;
public static int STDERR_FILENO = 2;
public static boolean HAVE_ISATTY;
public static boolean HAVE_TTYNAME;
public static int TCSANOW;
public static int TCSADRAIN;
public static int TCSAFLUSH;
public static long TIOCGETA;
public static long TIOCSETA;
public static long TIOCGETD;
public static long TIOCSETD;
public static long TIOCGWINSZ;
public static long TIOCSWINSZ;
/**
* test whether a file descriptor refers to a terminal
*
* @param fd file descriptor
* @return isatty() returns 1 if fd is an open file descriptor referring to a
* terminal; otherwise 0 is returned, and errno is set to indicate the
* error
* @see ISATTY(3) man-page
* @see ISATTY(3P) man-page
*/
public static native int isatty(int fd);
public static native String ttyname(int filedes);
/**
* The openpty() function finds an available pseudoterminal and returns
* file descriptors for the master and slave in amaster and aslave.
*
* @param amaster master return value
* @param aslave slave return value
* @param name filename return value
* @param termios optional pty attributes
* @param winsize optional size
* @return 0 on success
* @see OPENPTY(3) man-page
*/
public static native int openpty(
int[] amaster,
int[] aslave,
byte[] name,
Termios termios,
WinSize winsize);
public static native int tcgetattr(
int filedes,
Termios termios);
public static native int tcsetattr(
int filedes,
int optional_actions,
Termios termios);
/**
* Control a STREAMS device.
*
* @see IOCTL(3P) man-page
*/
public static native int ioctl(
int filedes,
long request,
int[] params);
public static native int ioctl(
int filedes,
long request,
WinSize params);
/**
* Window sizes.
*
* @see IOCTL_TTY(2) man-page
*/
public static class WinSize {
static {
JansiLoader.initialize();
init();
}
private static native void init();
public static int SIZEOF;
public short ws_row;
public short ws_col;
public short ws_xpixel;
public short ws_ypixel;
public WinSize() {
}
public WinSize(short ws_row, short ws_col) {
this.ws_row = ws_row;
this.ws_col = ws_col;
}
}
/**
* termios structure for termios functions, describing a general terminal interface that is
* provided to control asynchronous communications ports
*
* @see TERMIOS(3) man-page
*/
public static class Termios {
static {
JansiLoader.initialize();
init();
}
private static native void init();
public static int SIZEOF;
public long c_iflag;
public long c_oflag;
public long c_cflag;
public long c_lflag;
public byte[] c_cc = new byte[32];
public long c_ispeed;
public long c_ospeed;
}
public static native int setenv(String name, String value);
public static native int chdir(String path);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy