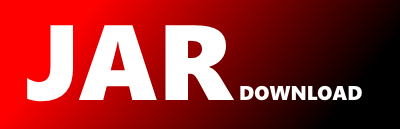
com.gwtplatform.dispatch.rest.delegates.client.AbstractResourceDelegate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dispatch-rest-delegates Show documentation
Show all versions of dispatch-rest-delegates Show documentation
Allow Rest-Dispatch resources to return their raw result type directly.
The newest version!
/**
* Copyright 2014 ArcBees Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.gwtplatform.dispatch.rest.delegates.client;
import com.google.gwt.user.client.rpc.AsyncCallback;
import com.gwtplatform.dispatch.client.DelegatingDispatchRequest;
import com.gwtplatform.dispatch.rest.client.RestDispatch;
import com.gwtplatform.dispatch.rest.shared.RestAction;
import com.gwtplatform.dispatch.shared.DispatchRequest;
/**
* Common code used by generated implementations of {@link ResourceDelegate}.
*
* @param The resource used by this delegate.
*/
public abstract class AbstractResourceDelegate implements ResourceDelegate, Cloneable {
private static final AsyncCallback
© 2015 - 2025 Weber Informatics LLC | Privacy Policy