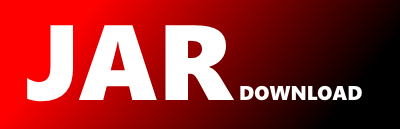
com.hack23.cia.service.user.impl.UserServiceImpl Maven / Gradle / Ivy
The newest version!
package com.hack23.cia.service.user.impl;
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Propagation;
import org.springframework.transaction.annotation.Transactional;
import com.hack23.cia.model.user.api.User;
import com.hack23.cia.service.user.api.NoSuchUser;
import com.hack23.cia.service.user.api.NoSuchUserException;
import com.hack23.cia.service.user.api.UserService;
@Transactional
@Service(value = "UserService")
public class UserServiceImpl implements UserService {
/** The entity manager. */
@PersistenceContext(name = "com.hack23.cia.model.user.api")
private EntityManager entityManager;
@Transactional(propagation = Propagation.REQUIRED, readOnly = false)
public void deleteUserById(final Integer userId) throws NoSuchUserException {
final User user = this.entityManager.find(User.class, userId);
this.entityManager.remove(user);
}
@Transactional(propagation = Propagation.SUPPORTS, readOnly = true)
public User getUserById(final Integer userId) throws NoSuchUserException {
final User user = this.entityManager.find(User.class, userId);
if (user == null) {
NoSuchUser noSuchUser = new NoSuchUser();
noSuchUser.setUserId(userId);
throw new NoSuchUserException(
"Did not find any matching user for id [" + userId + "].",
noSuchUser);
} else {
return user;
}
}
@Transactional(propagation = Propagation.REQUIRED, readOnly = false)
public Integer updateUser(User user) {
final User mergedUser = this.entityManager.merge(user);
return mergedUser.getModelObjectId();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy