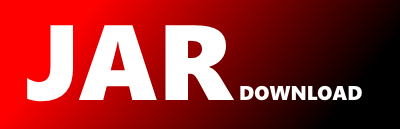
com.hadoopz.MyDroidLib.util.MyLogUtil Maven / Gradle / Ivy
/*
* Copyright 2017 jw362j.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.hadoopz.MyDroidLib.util;
import android.os.Environment;
import com.mycomm.MyConveyor.core.MyConveyor;
import com.mycomm.MyConveyor.core.TaskRequest;
import com.mycomm.MyConveyor.core.TaskType;
import com.mycomm.MyConveyor.core.Tasker;
import java.io.File;
import java.io.FileOutputStream;
import java.util.Date;
/**
*
* @author jw362j
*/
public class MyLogUtil {
private static final String log_tag_default = "MyLogUtilTag";
private static int log_flag = -100;
private static boolean log_runtime_switcher;
private static final String log_flag_runtime = "d33600715c5e79c155e268fbf99556tt.txt";
private static StringBuilder logBuffer;
private static final int logBufferMax = 1024 * 4;
private static final long logFileMaxSize = 1024 * 1024 * 2;
public static void ForceFlushLog() {
LogMe(log_tag_default, "flush the log buffer....", true);
}
public static void LogMe(String msg) {
LogMe(log_tag_default, msg);
}
public static void LogMe(String tag, String msg) {
LogMe(tag, msg, false);
}
private static void LogMe(String tag, final String msg, final boolean forceFlush) {
executeLogTask(new Tasker() {
public void onTask() {
if (log_flag == -100) {
log_flag = 1;
if (isFlagExist()) {
log_runtime_switcher = true;
} else {
return;
}
}
if (!log_runtime_switcher) {
return;
}
if (msg == null) {
return;
}
if (logBuffer == null) {
logBuffer = new StringBuilder();
}
logBuffer.append("Now is:").append(new Date()).append(":").append(msg).append("\n");
if (logBuffer.length() > logBufferMax || forceFlush) {
logOnFile(logBuffer.toString() + "\n");
}
}
});
}
private static boolean isFlagExist() {
boolean isCardExist = Environment.MEDIA_MOUNTED.equals(Environment.getExternalStorageState());
if (!isCardExist) {
return false;
}
File f = new File(Environment.getExternalStorageDirectory().getAbsolutePath() + File.separator + log_flag_runtime);
if (f.exists()) {
return true;
}
return false;
}
private static void logOnFile(final String msg) {
boolean needToRename = false;
boolean isCardExist = android.os.Environment.MEDIA_MOUNTED.equals(Environment.getExternalStorageState());
if (isCardExist) {
String sdFilePath = Environment.getExternalStorageDirectory().getAbsolutePath() + File.separator + "mylog" + File.separator;
try {
String fileName = sdFilePath + "log_holder" + ".log";
File filePath = new File(sdFilePath);
if (!filePath.exists()) {
filePath.mkdirs();
}
File file = new File(fileName);
if (!file.exists()) {
file.createNewFile();
} else if (file.length() > logFileMaxSize) {
needToRename = true;
}
FileOutputStream fos;
fos = new FileOutputStream(file, true);
fos.write(msg.getBytes());
fos.close();
if (needToRename) {
file.renameTo(new File(sdFilePath + System.currentTimeMillis() + ".log"));//.delete();
}
} catch (Exception e) {
System.err.println("error:" + e.getMessage());
}
logBuffer.delete(0, logBuffer.length());
} else {
System.out.println("sdcard is not ready!");
}
}
private static void executeLogTask(final Tasker tasker) {
MyConveyor.getInstance().execute(new TaskRequest() {
public TaskType getTaskType() {
return TaskType.TASK_LOG;
}
public Tasker getTask() {
return tasker;
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy