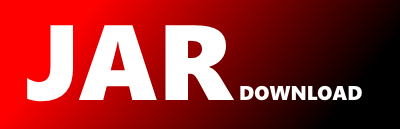
com.hadoopz.MyDroidLib.image.SDCardImageLoader Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2019 jw362j.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.hadoopz.MyDroidLib.image;
import android.content.Context;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import com.mycomm.IProtocol.log.UniversalLogHolder;
import com.mycomm.MyConveyor.core.MyConveyor;
import com.mycomm.MyConveyor.core.TaskRequest;
import com.mycomm.MyConveyor.core.TaskType;
import com.mycomm.MyConveyor.core.Tasker;
import com.mycomm.YesHttp.core.FileDownLoadResponse;
import com.mycomm.YesHttp.core.HttpMethod;
import com.mycomm.YesHttp.core.Request;
import com.mycomm.YesHttp.core.Response;
import com.mycomm.YesHttp.core.StringRequest;
import com.mycomm.YesHttp.core.YesHttpEngine;
import com.mycomm.itool.SystemUtil;
import java.io.File;
/**
*
* @author jw362j
*/
public class SDCardImageLoader implements ImgLoader {
private final Context mContext;
private final String SDCARD_DIR_CACHE;
private static ImgLoader imgLoader;
private static final Request.YesLog yeslog = new Request.YesLog() {
public void w(String text) {
UniversalLogHolder.w(getClass().getSimpleName(), text);
}
public void e(String text) {
UniversalLogHolder.e(getClass().getSimpleName(), text);
}
public void d(String text) {
UniversalLogHolder.d(getClass().getSimpleName(), text);
}
public void i(String text) {
UniversalLogHolder.i(getClass().getSimpleName(), text);
}
public void v(String text) {
UniversalLogHolder.v(getClass().getSimpleName(), text);
}
};
private SDCardImageLoader(Context context) {
this.mContext = context;
String baseFilePath = mContext.getExternalFilesDir("imgCache").getAbsolutePath();
if (!baseFilePath.endsWith(File.separator)) {
baseFilePath += File.separator;
}
SDCARD_DIR_CACHE = baseFilePath;
File x = new File(SDCARD_DIR_CACHE);
if (!x.exists()) {
x.mkdir();
}
UniversalLogHolder.d(getClass().getSimpleName(), "the img cache dir is:" + baseFilePath);
}
public static ImgLoader getImgLoader(Context context) {
if (imgLoader == null) {
imgLoader = new SDCardImageLoader(context);
}
return imgLoader;
}
public void loadImage(String imageUrl, LoadImgListener imgListener) {
loadImage(imageUrl, imgListener, false);
}
public void loadImage(final String imageUrl, final LoadImgListener imgListener, final boolean forceLoad) {
if (SystemUtil.isTxtEmpty(imageUrl)) {
if (imgListener != null) {
imgListener.onFailed(new IllegalArgumentException("please make sure you pass the correct image url!"));
}
return;
}
runHeavyTask(new Tasker() {
public void onTask() {
if (forceLoad) {
executeDownload(imageUrl, imgListener, ImageFrom.NETWORK_URL);
return;
}
File theTarget = new File(SDCARD_DIR_CACHE + SystemUtil.getMD5(imageUrl.getBytes()));
if (theTarget.exists()) {
UniversalLogHolder.d(getClass().getSimpleName(), "the file exists...");
if (imgListener != null) {
String fileAbsPath = SDCARD_DIR_CACHE + SystemUtil.getMD5(imageUrl.getBytes());
Bitmap bitmap = BitmapFactory.decodeFile(fileAbsPath);
imgListener.onSuccess(bitmap, ImageFrom.SDCARD);
if (!bitmap.isRecycled()) {
bitmap.recycle();
}
}
return;
}
executeDownload(imageUrl, imgListener, ImageFrom.NETWORK_URL);
}
});
}
private void runHeavyTask(final Tasker tasker) {
MyConveyor.getInstance().execute(new TaskRequest() {
public TaskType getTaskType() {
return TaskType.TASK_IMG_DOWNLOAD;
}
public Tasker getTask() {
return tasker;
}
});
}
private void executeDownload(final String imageUrl, final LoadImgListener imgListener, final ImageFrom imageFrom) {
Request request = new StringRequest(HttpMethod.GET, imageUrl,
new FileDownLoadResponse(SDCARD_DIR_CACHE, yeslog, new Response.DownLoadUpLoadListener() {
public void onProgressing(float rate) {
UniversalLogHolder.d(getClass().getSimpleName(), "the download rate :" + rate);
}
}, null) {
@Override
public void responseMe(String newFileNameSimple) {
UniversalLogHolder.d(getClass().getSimpleName(), "the file name of newFileNameSimple:" + newFileNameSimple);
if (imgListener != null) {
imgListener.onSuccess(BitmapFactory.decodeFile(SDCARD_DIR_CACHE + newFileNameSimple), imageFrom);
}
}
}, null, yeslog, Request.Protocol.HTTP);
YesHttpEngine.getYesHttpEngine().send(request);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy