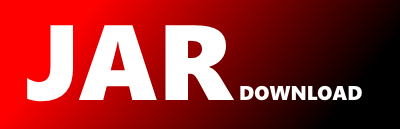
com.mycomm.YesHttp.core.Request Maven / Gradle / Ivy
/*
* Copyright 2018 jw362j.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.mycomm.YesHttp.core;
import java.net.URLConnection;
import java.util.Map;
/**
*
* @author jw362j
*/
public abstract class Request {
public static final String DEFAULT_PARAMS_ENCODING = "UTF-8";
/**
* Supported request methods.
*/
public interface Method {
short DEPRECATED_GET_OR_POST = -1;
short GET = 0;
short POST = 1;
short PUT = 2;
short DELETE = 3;
short HEAD = 4;
short OPTIONS = 5;
short TRACE = 6;
short PATCH = 7;
}
public interface YesLog {
public void LogMe(String msg);
}
public interface HttpObserver {
public void Observe(URLConnection connection);
}
public interface Protocol {
short HTTP = 0;
short HTTPS = 1;
short HTTPS_IGNORE_CERT = 2;
}
private final short mMethod;
/**
* URL of this request.
*/
private final String mUrl;
private final Response.Listener response;
private final Response.ErrorListener errorListener;
private final HttpObserver httpObserver;
private final YesLog log;
private final short protocol;
public Request(String mUrl, Response.Listener response) {
this((short)0, mUrl, response, null, null, (short)0);
}
public Request(String mUrl, Response.Listener response, Response.ErrorListener errorListener) {
this((short)0, mUrl, response, errorListener, null, (short)0);
}
public Request(short mMethod, String mUrl, Response.Listener response, Response.ErrorListener errorListener, YesLog log, short protocol) {
this(mMethod, mUrl, response, null, errorListener, log, protocol);
}
public Request(short mMethod, String mUrl, Response.Listener response, HttpObserver observer, Response.ErrorListener errorListener, YesLog log, short protocol) {
this.mMethod = mMethod;
this.mUrl = mUrl;
this.response = response;
this.errorListener = errorListener;
this.log = log;
this.protocol = protocol;
this.httpObserver = observer;
}
public YesLog getLog() {
return log;
}
public abstract Map getParams();
public abstract int getReadTimeout();
public abstract int getConnectTimeout();
public abstract Map getHeaders();
public abstract boolean useGzip();
public String getmUrl() {
return mUrl;
}
public short getmMethod() {
return mMethod;
}
public short getProtocol() {
return protocol;
}
public Response.Listener getResponse() {
return response;
}
public Response.ErrorListener getErrorListener() {
return errorListener;
}
public HttpObserver getHttpObserver() {
return httpObserver;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy