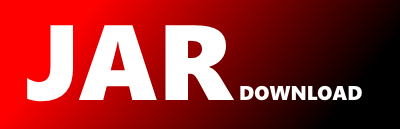
com.mycomm.YesHttp.core.JsonRequest Maven / Gradle / Ivy
/*
* Copyright 2018 jw362j.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.mycomm.YesHttp.core;
import java.io.IOException;
import java.io.OutputStream;
import java.net.URLConnection;
import java.net.URLEncoder;
import java.util.Set;
import java.util.zip.GZIPOutputStream;
/**
*
* @author jw362j
*/
public abstract class JsonRequest extends BaseRequest {
private GZIPOutputStream gZIPOutputStream;
private final HttpObserver observer = new HttpObserver() {
public void Observe(URLConnection connection) {
if (!HttpMethod.POST.equals( getmMethod() ) && !HttpMethod.PUT.equals( getmMethod() ) ) {
getLog().d("not post data not put in JsonRequest =====");
return;
}
if (connection == null) {
return;
}
connection.setRequestProperty("Content-Type", "application/json");
}
};
public JsonRequest(String mUrl, Response.Listener response, Response.ErrorListener errorListener, short protocol) {
super(HttpMethod.PUT, mUrl, response, errorListener, null,protocol);
}
public JsonRequest(String mUrl, Response.Listener response, Response.ErrorListener errorListener, YesLog log, short protocol) {
super(HttpMethod.POST, mUrl, response, errorListener, log, protocol);
}
public JsonRequest(String mUrl, Response.Listener response, HttpObserver observer, Response.ErrorListener errorListener, YesLog log, short protocol) {
super(HttpMethod.POST, mUrl, response, observer, errorListener, log, protocol);
}
@Override
public HttpObserver getHttpObserver() {
return observer;
}
public void write(OutputStream outputStream) {
try {
if (!HttpMethod.POST.equals( getmMethod() ) && !HttpMethod.PUT.equals( getmMethod() ) ) {
getLog().d("not post data not put in JsonRequest =====xx");
return;
}
String targetJsonData = JsonBodyBuilder();
if (targetJsonData != null && targetJsonData.length() > 0) {
getLog().d("the post data in JsonRequest is:" + targetJsonData);
if (useGzip()) {
gZIPOutputStream = new GZIPOutputStream(outputStream);
gZIPOutputStream.write(targetJsonData.getBytes(DEFAULT_PARAMS_ENCODING));
gZIPOutputStream.flush();
gZIPOutputStream.close();
} else {
outputStream.write(targetJsonData.getBytes(DEFAULT_PARAMS_ENCODING));
}
return;
}
getParams(params);
if (params == null ||params.isEmpty()) {
getLog().d("Params are null in Method.POST about JsonRequest,post body output give up ...");
getErrorListener().onErrorResponse(new YesHttpError("JsonBodyBuilder() is returnning null value or getParams()returning the null parameters!"));
return;
}
StringBuilder post_data_defaultJson = new StringBuilder();
post_data_defaultJson.append("{");
Set keys = params.keySet();
for (String key : keys) {
if(params.get(key) != null)post_data_defaultJson.append("\"").append(URLEncoder.encode(key,DEFAULT_PARAMS_ENCODING)).append("\"").append(":").append("\"").append(URLEncoder.encode(params.get(key),DEFAULT_PARAMS_ENCODING)).append("\"").append(",");
}
post_data_defaultJson.deleteCharAt(post_data_defaultJson.length() - 1);
post_data_defaultJson.append("}");
targetJsonData = post_data_defaultJson.toString();
getLog().d("the post data in JsonRequestA is:" + targetJsonData);
if (useGzip()) {
gZIPOutputStream = new GZIPOutputStream(outputStream);
gZIPOutputStream.write(targetJsonData.getBytes(DEFAULT_PARAMS_ENCODING));
gZIPOutputStream.flush();
gZIPOutputStream.close();
} else {
outputStream.write(targetJsonData.getBytes(DEFAULT_PARAMS_ENCODING));
}
} catch (IOException ex) {
getLog().e("Exception in StringRequest write:" + ex.getMessage());
}
}
public abstract String JsonBodyBuilder();//have to build the json format request body ,if none-null is returned here,then would ignore getParams() method
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy