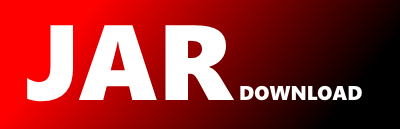
com.hfg.javascript.JsArray Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com_hfg Show documentation
Show all versions of com_hfg Show documentation
com.hfg xml, html, svg, and bioinformatics utility library
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy