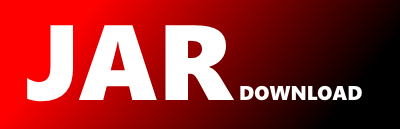
com.hfg.citation.Citation Maven / Gradle / Ivy
package com.hfg.citation;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import com.hfg.util.StringBuilderPlus;
//------------------------------------------------------------------------------
/**
Citation data object.
@author J. Alex Taylor, hairyfatguy.com
*/
//------------------------------------------------------------------------------
// com.hfg XML/HTML Coding Library
//
// This library is free software; you can redistribute it and/or
// modify it under the terms of the GNU Lesser General Public
// License as published by the Free Software Foundation; either
// version 2.1 of the License, or (at your option) any later version.
//
// This library is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
// Lesser General Public License for more details.
//
// You should have received a copy of the GNU Lesser General Public
// License along with this library; if not, write to the Free Software
// Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
//
// J. Alex Taylor, President, Founder, CEO, COO, CFO, OOPS hairyfatguy.com
// [email protected]
//------------------------------------------------------------------------------
public class Citation
{
private CitationType mType;
private String mAbstract;
private Date mAccessDate;
private List mAuthors;
private String mCallNumber;
private String mArticleNumber;
private String mDOI;
private String mISBN;
private String mISSN;
private String mIssue;
private Journal mJournal;
private MediaType mMediaType;
private String mNote;
private String mPages;
private String mPlaceOfPublication;
private String mPublisher;
private String mRepository;
private String mSource;
private String mTitle;
private String mURI;
private String mURL;
private String mVersion;
private String mVolume;
private String mWebsiteTitle;
private Integer mYear;
private String mLanguage;
private String mInstitution;
private PatentData mPatentData;
private String mRawContent;
//###########################################################################
// CONSTRUCTORS
//###########################################################################
//---------------------------------------------------------------------------
public Citation()
{
init();
}
//---------------------------------------------------------------------------
public Citation(String inValue)
{
this();
setRawContent(inValue);
}
//---------------------------------------------------------------------------
private void init()
{
setType(CitationType.general);
}
//###########################################################################
// PUBLIC METHODS
//###########################################################################
//---------------------------------------------------------------------------
@Override
public String toString()
{
String output;
if (mRawContent != null)
{
output = mRawContent;
}
else
{
output = new APA().generateAsString(this);
}
return output;
}
//---------------------------------------------------------------------------
public Citation setRawContent(String inValue)
{
mRawContent = inValue;
return this;
}
//---------------------------------------------------------------------------
public Citation appendRawContent(String inValue)
{
mRawContent = (mRawContent != null ? mRawContent + " " : "") + inValue;
return this;
}
//---------------------------------------------------------------------------
public Citation setType(CitationType inValue)
{
mType = inValue;
return this;
}
//---------------------------------------------------------------------------
public CitationType getType()
{
return mType;
}
//---------------------------------------------------------------------------
public Citation setAbstract(String inValue)
{
mAbstract = inValue;
return this;
}
//---------------------------------------------------------------------------
public String getAbstract()
{
return mAbstract;
}
//---------------------------------------------------------------------------
public Citation setAccessDate(Date inValue)
{
mAccessDate = inValue;
return this;
}
//---------------------------------------------------------------------------
public Date getAccessDate()
{
return mAccessDate;
}
//---------------------------------------------------------------------------
public Citation addAuthor(Author inValue)
{
if (null == mAuthors)
{
mAuthors = new ArrayList<>(5);
}
mAuthors.add(inValue);
return this;
}
//---------------------------------------------------------------------------
public List getAuthors()
{
return mAuthors;
}
//---------------------------------------------------------------------------
/**
Specify the library call number for the citation source.
@param inValue the library call number
@return this Citation object to facilitate method chaining.
*/
public Citation setCallNumber(String inValue)
{
mCallNumber = inValue;
return this;
}
//---------------------------------------------------------------------------
public String getCallNumber()
{
return mCallNumber;
}
//---------------------------------------------------------------------------
/**
Specify the article number for the citation source. Often used by online-only publishers.
@param inValue the article number
@return this Citation object to facilitate method chaining.
*/
public Citation setArticleNumber(String inValue)
{
mArticleNumber = inValue;
return this;
}
//---------------------------------------------------------------------------
public String getArticleNumber()
{
return mArticleNumber;
}
//---------------------------------------------------------------------------
public Citation setYear(Integer inValue)
{
mYear = inValue;
return this;
}
//---------------------------------------------------------------------------
public Integer getYear()
{
return mYear;
}
//---------------------------------------------------------------------------
public Citation setJournal(Journal inValue)
{
mJournal = inValue;
if (inValue != null)
{
setType(CitationType.journal);
}
return this;
}
//---------------------------------------------------------------------------
public Journal getJournal()
{
return mJournal;
}
//---------------------------------------------------------------------------
public Citation setIssue(String inValue)
{
mIssue = inValue;
return this;
}
//---------------------------------------------------------------------------
public String getIssue()
{
return mIssue;
}
//---------------------------------------------------------------------------
/**
Specify the media type for the citation source material.
@param inValue the media type for the citation source material
@return this Citation object to facilitate method chaining.
*/
public Citation setMediaType(MediaType inValue)
{
mMediaType = inValue;
return this;
}
//---------------------------------------------------------------------------
public MediaType getMediaType()
{
return mMediaType;
}
//---------------------------------------------------------------------------
/**
Specify additional citation details, commentary, or summary.
@param inValue the note contents
@return this Citation object to facilitate method chaining.
*/
public Citation setNote(String inValue)
{
mNote = inValue;
return this;
}
//---------------------------------------------------------------------------
public String getNote()
{
return mNote;
}
//---------------------------------------------------------------------------
public Citation setPages(String inValue)
{
mPages = inValue;
return this;
}
//---------------------------------------------------------------------------
public String getPages()
{
return mPages;
}
//---------------------------------------------------------------------------
public Citation setPublisher(String inValue)
{
mPublisher = inValue;
return this;
}
//---------------------------------------------------------------------------
public String getPublisher()
{
return mPublisher;
}
//---------------------------------------------------------------------------
public Citation setPlaceOfPublication(String inValue)
{
mPlaceOfPublication = inValue;
return this;
}
//---------------------------------------------------------------------------
public String getPlaceOfPublication()
{
return mPlaceOfPublication;
}
//---------------------------------------------------------------------------
/**
Specifies the digital object identifier (DOI) for the citation.
The DOI is a unique alphanumeric string assigned by a registration agency
(the International DOI Foundation) to identify content and provide a persistent
link to its location on the Internet.
@param inValue the DOI string
@return this Citation object to facilitate method chaining.
*/
public Citation setDOI(String inValue)
{
mDOI = inValue;
return this;
}
//---------------------------------------------------------------------------
public String getDOI()
{
return mDOI;
}
//---------------------------------------------------------------------------
/**
Specifies the international standard book number (ISBN) for the citation.
@param inValue the ISBN string
@return this Citation object to facilitate method chaining.
*/
public Citation setISBN(String inValue)
{
mISBN = inValue;
return this;
}
//---------------------------------------------------------------------------
public String getISBN()
{
return mISBN;
}
//---------------------------------------------------------------------------
/**
Specifies the international standard serial number (ISSN) for the citation.
From the issn.org website: "An ISSN is an 8-digit code used to identify
newspapers, journals, magazines and periodicals of all kinds and on all
media–print and electronic."
@param inValue the ISSN string
@return this Citation object to facilitate method chaining.
*/
public Citation setISSN(String inValue)
{
mISSN = inValue;
return this;
}
//---------------------------------------------------------------------------
public String getISSN()
{
return mISSN;
}
//---------------------------------------------------------------------------
/**
Specifies the repository in which the citation source was accessed.
@param inValue the name of the repository
@return this Citation object to facilitate method chaining.
*/
public Citation setRepository(String inValue)
{
mRepository = inValue;
return this;
}
//---------------------------------------------------------------------------
public String getRepository()
{
return mRepository;
}
//---------------------------------------------------------------------------
/**
Specifies the origin of the citation material.
@param inValue the citation source
@return this Citation object to facilitate method chaining.
*/
public Citation setSource(String inValue)
{
mSource = inValue;
return this;
}
//---------------------------------------------------------------------------
public String getSource()
{
return mSource;
}
//---------------------------------------------------------------------------
public Citation setTitle(String inValue)
{
mTitle = inValue;
return this;
}
//---------------------------------------------------------------------------
public String getTitle()
{
return mTitle;
}
//---------------------------------------------------------------------------
public Citation setURI(String inValue)
{
mURI = inValue;
return this;
}
//---------------------------------------------------------------------------
public String getURI()
{
return mURI;
}
//---------------------------------------------------------------------------
public Citation setURL(String inValue)
{
mURL = inValue;
return this;
}
//---------------------------------------------------------------------------
public String getURL()
{
return mURL;
}
//---------------------------------------------------------------------------
public Citation setVersion(String inValue)
{
mVersion = inValue;
return this;
}
//---------------------------------------------------------------------------
public String getVersion()
{
return mVersion;
}
//---------------------------------------------------------------------------
public Citation setVolume(String inValue)
{
mVolume = inValue;
return this;
}
//---------------------------------------------------------------------------
public String getVolume()
{
return mVolume;
}
//---------------------------------------------------------------------------
public Citation setWebsiteTitle(String inValue)
{
mWebsiteTitle = inValue;
return this;
}
//---------------------------------------------------------------------------
public String getWebsiteTitle()
{
return mWebsiteTitle;
}
//---------------------------------------------------------------------------
public Citation setLanguage(String inValue)
{
mLanguage = inValue;
return this;
}
//---------------------------------------------------------------------------
public String getLanguage()
{
return mLanguage;
}
//---------------------------------------------------------------------------
public Citation setInstitution(String inValue)
{
mInstitution = inValue;
return this;
}
//---------------------------------------------------------------------------
public String getInstitution()
{
return mInstitution;
}
//---------------------------------------------------------------------------
public Citation setPatentData(PatentData inValue)
{
mPatentData = inValue;
return this;
}
//---------------------------------------------------------------------------
public PatentData getPatentData()
{
return mPatentData;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy