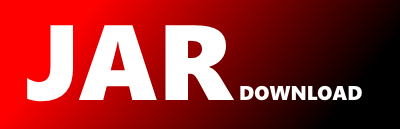
com.hfg.graphics.GraphicsUtil Maven / Gradle / Ivy
package com.hfg.graphics;
import java.awt.*;
import java.awt.geom.Path2D;
import java.awt.geom.Point2D;
import java.awt.geom.Rectangle2D;
//------------------------------------------------------------------------------
/**
* Utility methods for use with Graphics2D.
*
* @author J. Alex Taylor, hairyfatguy.com
*
*/
//------------------------------------------------------------------------------
// com.hfg XML/HTML Coding Library
//
// This library is free software; you can redistribute it and/or
// modify it under the terms of the GNU Lesser General Public
// License as published by the Free Software Foundation; either
// version 2.1 of the License, or (at your option) any later version.
//
// This library is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
// Lesser General Public License for more details.
//
// You should have received a copy of the GNU Lesser General Public
// License along with this library; if not, write to the Free Software
// Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
//
// J. Alex Taylor, President, Founder, CEO, COO, CFO, OOPS hairyfatguy.com
// [email protected]
//------------------------------------------------------------------------------
public class GraphicsUtil
{
//--------------------------------------------------------------------------
public static Path2D rotate(Rectangle2D inRect, float inAngleInDegrees, Point2D inCenterOfRotation)
{
Point2D.Double p1 = new Point2D.Double(inRect.getMinX(), inRect.getMinY());
Point2D.Double p2 = new Point2D.Double(inRect.getMaxX(), inRect.getMinY());
Point2D.Double p3 = new Point2D.Double(inRect.getMinX(), inRect.getMaxY());
Point2D.Double p4 = new Point2D.Double(inRect.getMaxX(), inRect.getMaxY());
Double cx = inCenterOfRotation.getX();
Double cy = inCenterOfRotation.getY();
double angleInRadians = Math.toRadians(inAngleInDegrees);
p1.setLocation((p1.getX()-cx) * Math.cos(angleInRadians) - (p1.getY()-cy) * Math.sin(angleInRadians)+cx,
(p1.getX()-cx) * Math.sin(angleInRadians) + (p1.getY()-cy) * Math.cos(angleInRadians)+cy);
p2.setLocation((p2.getX()-cx) * Math.cos(angleInRadians) - (p2.getY()-cy) * Math.sin(angleInRadians)+cx,
(p2.getX()-cx) * Math.sin(angleInRadians) + (p2.getY()-cy) * Math.cos(angleInRadians)+cy);
p3.setLocation((p3.getX()-cx) * Math.cos(angleInRadians) - (p3.getY()-cy) * Math.sin(angleInRadians)+cx,
(p3.getX()-cx) * Math.sin(angleInRadians) + (p3.getY()-cy) * Math.cos(angleInRadians)+cy);
p4.setLocation((p4.getX()-cx) * Math.cos(angleInRadians) - (p4.getY()-cy) * Math.sin(angleInRadians)+cx,
(p4.getX()-cx) * Math.sin(angleInRadians) + (p4.getY()-cy) * Math.cos(angleInRadians)+cy);
double[] x = {p1.getX(), p2.getX(), p3.getX(), p4.getX()};
double[] y = {p1.getY(), p2.getY(), p3.getY(), p4.getY()};
Path2D path = new Path2D.Double(Path2D.WIND_NON_ZERO, 2);
path.moveTo(p1.getX(), p1.getY());
path.lineTo(p2.getX(), p2.getY());
path.lineTo(p3.getX(), p3.getY());
path.lineTo(p4.getX(), p4.getY());
path.closePath();
return path;
}
//--------------------------------------------------------------------------
public static Polygon rotate(Rectangle inRect, float inAngleInDegrees, Point2D inCenterOfRotation)
{
Point2D.Double p1 = new Point2D.Double(inRect.getMinX(), inRect.getMinY());
Point2D.Double p2 = new Point2D.Double(inRect.getMaxX(), inRect.getMinY());
Point2D.Double p3 = new Point2D.Double(inRect.getMinX(), inRect.getMaxY());
Point2D.Double p4 = new Point2D.Double(inRect.getMaxX(), inRect.getMaxY());
Double cx = inCenterOfRotation.getX();
Double cy = inCenterOfRotation.getY();
double angleInRadians = Math.toRadians(inAngleInDegrees);
p1.setLocation((p1.getX()-cx) * Math.cos(angleInRadians) - (p1.getY()-cy) * Math.sin(angleInRadians)+cx,
(p1.getX()-cx) * Math.sin(angleInRadians) + (p1.getY()-cy) * Math.cos(angleInRadians)+cy);
p2.setLocation((p2.getX()-cx) * Math.cos(angleInRadians) - (p2.getY()-cy) * Math.sin(angleInRadians)+cx,
(p2.getX()-cx) * Math.sin(angleInRadians) + (p2.getY()-cy) * Math.cos(angleInRadians)+cy);
p3.setLocation((p3.getX()-cx) * Math.cos(angleInRadians) - (p3.getY()-cy) * Math.sin(angleInRadians)+cx,
(p3.getX()-cx) * Math.sin(angleInRadians) + (p3.getY()-cy) * Math.cos(angleInRadians)+cy);
p4.setLocation((p4.getX()-cx) * Math.cos(angleInRadians) - (p4.getY()-cy) * Math.sin(angleInRadians)+cx,
(p4.getX()-cx) * Math.sin(angleInRadians) + (p4.getY()-cy) * Math.cos(angleInRadians)+cy);
int[] x = {(int) p1.getX(), (int) p2.getX(), (int)p3.getX(), (int) p4.getX()};
int[] y = {(int) p1.getY(), (int) p2.getY(), (int)p3.getY(), (int) p4.getY()};
return new Polygon(x, y, x.length);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy