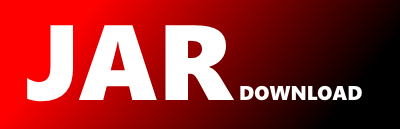
com.hannesdorfmann.httpkit.parser.LoganSquareParserWriter Maven / Gradle / Ivy
package com.hannesdorfmann.httpkit.parser;
import com.bluelinelabs.logansquare.LoganSquare;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.PrintWriter;
/**
* Uses Logan Square parser writer
* @author Hannes Dorfmann
*/
public class LoganSquareParserWriter implements ParserWriter
© 2015 - 2025 Weber Informatics LLC | Privacy Policy