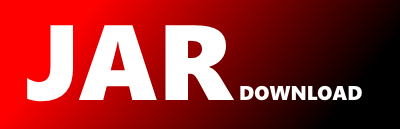
com.hannesdorfmann.httpkit.parser.ParserWriter Maven / Gradle / Ivy
package com.hannesdorfmann.httpkit.parser;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.List;
import java.util.Map;
/**
* A {@link ParserWriter} can "parse" a {@link InputStream} into a real object
* and vice versa (write an Object to the original data format, like json, xml
* or evene binary image data etc.)
*
* @param The type of the real object that will be parsed
* @author Hannes Dorfmann
*/
public interface ParserWriter {
/**
* Indicates that the collection type should not be used while parsing, and
* return a single class instance instead of a collection like {@link List},
* {@link Map}, etc ...
*/
public static final int COLLECTION_TYPE_NONE = -1;
/**
* Indicates, that the result of the parsing process should be returned as
* List. the default List implementation is ArrayList
*/
public static final int COLLECTION_TYPE_LIST = 1;
/**
* Take a {@link InputStream} and parse it to a "real" object
*
* @param in The {@link InputStream}
* @param targetClass The Target class the data should be parsed in. Normally only used if json or xml should be parsed.
* @param collectionType Here you specify which kind o
* @param mimeType The mimeType, because for example a image parser can parse JPG
* and PNG and GIF in the same procedure
* @param charset The charset that is used for string text encoding. For binary
* data this parameter is useless and can be ingnored
*/
public T parse(InputStream in, Class> targetClass, int collectionType,
String mimeType, String charset) throws Exception;
/**
* This method writes the passed oject to an outputstream
*
* @param out
* @param value
* @param mimeType the mime type that should be written
* @param charset The charset that is used for string text encoding. For binary
* data this parameter is useless and can be ingnored
* @throws Exception
*/
public void write(OutputStream out, T value, String mimeType, String charset)
throws Exception;
/**
* Write the data as raw bytes
* @param out
* @param value
* @param mimeType
* @param charset
* @throws IOException
*/
public void writeRaw(OutputStream out, byte[] value, String mimeType, String charset) throws IOException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy