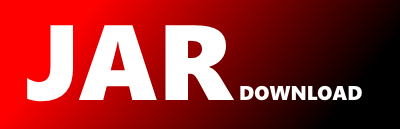
com.hannesdorfmann.httpkit.request.HttpKeyValueEntityRequest Maven / Gradle / Ivy
package com.hannesdorfmann.httpkit.request;
import java.net.URLEncoder;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
/**
*
* This {@link HttpRequest} is used to send http body entity in text from. This
* is normally used for HTTP POST, PUT. It simply contains a key - value map for
* transmitting data as HTTP POST or PUT parameter with the corresponding value
* to the web server. However you should not use this base class but use
* the concrete classes like {@link HttpPostKeyValueRequest} or
* {@link HttpPutKeyValueRequest}, because they may also add more http method
* specific http headers
*
*
*
* Only text values are transmitted as URLEncoded strings. For Transmitting
* binary data, or multipart you must use another {@link HttpRequest}
* implementation
*
*
* @author Hannes Dorfmann
*
*/
public abstract class HttpKeyValueEntityRequest extends AbstractHttpRequest {
/**
* The encoding that is used in combination with
* {@link URLEncoder#encode(String, String)}
*/
private String encodingCharset = "utf-8";
private final Map paramsMap;
/**
* Creates a new object
*
* @param httpMethod
* Should be {@link HttpRequest#HTTP_METHOD_POST} etc.
*/
public HttpKeyValueEntityRequest(String url, String httpMethod) {
setHttpMethod(httpMethod);
setUrl(url);
paramsMap = new LinkedHashMap();
// Setting up the headers
Map headers = getHttpHeaders();
if (headers == null)
headers = new HashMap();
headers.put("Accept-Charset", encodingCharset);
}
public String getCharsetEncoding() {
return encodingCharset;
}
public void setCharsetEnconding(String encoding) {
this.encodingCharset = encoding;
}
public String putParam(String key, String value) {
if (key == null)
throw new NullPointerException(
"Could not put a parameter, because the parameter name (key) is null for "
+ super.getUrl());
if (value == null)
throw new NullPointerException(
"Could not put the parameter with the name " + key
+ " , because the value was null for "
+ super.getUrl());
return paramsMap.put(key, value);
}
public String putParam(String key, int value) {
if (key == null)
throw new NullPointerException(
"Could not put a parameter, because the parameter name (key) is null for "
+ super.getUrl());
return paramsMap.put(key, Integer.toString(value));
}
public String putParam(String key, boolean value) {
if (key == null)
throw new NullPointerException(
"Could not put a parameter, because the parameter name (key) is null for "
+ super.getUrl());
return paramsMap.put(key, Boolean.toString(value));
}
public String putParam(String key, long value) {
if (key == null)
throw new NullPointerException(
"Could not put a parameter, because the parameter name (key) is null for "
+ super.getUrl());
return paramsMap.put(key, Long.toString(value));
}
public String putParam(String key, double value) {
if (key == null)
throw new NullPointerException(
"Could not put a parameter, because the parameter name (key) is null for "
+ super.getUrl());
return paramsMap.put(key, Double.toString(value));
}
public String putParam(String key, float value) {
if (key == null)
throw new NullPointerException(
"Could not put a parameter, because the parameter name (key) is null for "
+ super.getUrl());
return paramsMap.put(key, Float.toString(value));
}
public String putParam(String key, short value) {
if (key == null)
throw new NullPointerException(
"Could not put a parameter, because the parameter name (key) is null for "
+ super.getUrl());
return paramsMap.put(key, Short.toString(value));
}
public String putParam(String key, char value) {
if (key == null)
throw new NullPointerException(
"Could not put a parameter, because the parameter name (key) is null for "
+ super.getUrl());
return paramsMap.put(key, Character.toString(value));
}
/**
* Returns the parameters name and value in a string that can be sent with
* the http request to transmit the parameters
*
* @return
*/
protected String getParamsString() {
StringBuffer buf = new StringBuffer();
int i = 0;
for (Entry e : paramsMap.entrySet()) {
if (i != 0)
buf.append("&");
buf.append(e.getKey() + "=" + e.getValue());
i++;
}
return buf.toString();
}
/**
* Get the count of how many
*
* @return
*/
public int getKeyValuesCount() {
return paramsMap.size();
}
/**
* Get the entry set of the key value map
*
* @return
*/
public Set> getKeyValues() {
return paramsMap.entrySet();
}
@Override
public String toString() {
return super.toString() + " " + getHttpMethod() + " " + getUrl() + " "
+ paramsMap.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy