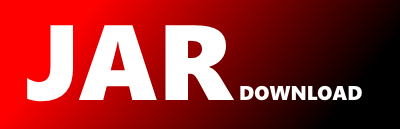
com.hannesdorfmann.httpkit.util.CacheUtils Maven / Gradle / Ivy
package com.hannesdorfmann.httpkit.util;
import android.annotation.SuppressLint;
import android.app.ActivityManager;
import android.content.Context;
import android.content.pm.PackageInfo;
import android.content.pm.PackageManager;
import android.os.Build;
import java.io.File;
import static android.content.Context.ACTIVITY_SERVICE;
import static android.content.pm.ApplicationInfo.FLAG_LARGE_HEAP;
/**
* @author Hannes Dorfmann
*/
public class CacheUtils {
/**
* The maximum of a Memory cache
*/
private static final int MAX_MEM_CACHE_SIZE = 40 * 1024 * 1024; // 40MB
/**
* Get the memory class.
*
* Return the approximate per-application memory class of the current
* device. This gives you an idea of how hard a memory limit you should
* impose on your application to let the overall system work best. The
* returned value is in megabytes; the baseline Android memory class is 16
* (which happens to be the Java heap limit of those devices); some device
* with more memory may return 24 or even higher numbers.
*
*
* @param c
* @return
*/
public static int getMemoryClass(Context c) {
ActivityManager activityManager = (ActivityManager) c
.getApplicationContext().getSystemService(
Context.ACTIVITY_SERVICE);
return activityManager.getMemoryClass();
}
/**
* Get the current free ram in Mega Bytes
*
* @return
*/
public static long getCurrentRam(Context c) {
ActivityManager.MemoryInfo mi = new ActivityManager.MemoryInfo();
ActivityManager activityManager = (ActivityManager) c
.getApplicationContext().getSystemService(
Context.ACTIVITY_SERVICE);
activityManager.getMemoryInfo(mi);
long availableMegs = mi.availMem / 1048576L;
return availableMegs;
}
// /**
// * Calculates a good size for a dedicated Bitmap memory cache. It returns
// * the size of the cache in bytes.
// *
// * @param c
// * A context
// * @return The size in bytes
// */
// public static int getBitmapCacheSize(Context c) {
//
// int memoryClass = getMemoryClass(c);
//
// // MemoryClass 16 MB
// if (memoryClass <= 16)
// return 2 * 1024 * 1024;
//
// // Memory class 24 MB
// if (memoryClass <= 24)
// return 4 * 1024 * 1024;
//
// // Memory class 32
// if (memoryClass <= 32)
// return 6 * 1024 * 1024;
//
// // Memory class 64
// if (memoryClass <= 64)
// return 12 * 1024 * 1024;
//
// // Memory class 128
// if (memoryClass <= 128)
// return 24 * 1024 * 1024;
//
// // More than Memory class 128
// return 48 * 1024 * 1024;
//
// }
/**
* Calculates the memory cache
*
* @param context
* @return
*/
@SuppressLint("NewApi")
public static int getBitmapMemoryCacheSize(Context context) {
ActivityManager am = (ActivityManager) context
.getSystemService(ACTIVITY_SERVICE);
boolean largeHeap = (context.getApplicationInfo().flags & FLAG_LARGE_HEAP) != 0;
int memoryClass = am.getMemoryClass();
if (largeHeap && Build.VERSION.SDK_INT >= Build.VERSION_CODES.HONEYCOMB) {
memoryClass = am.getLargeMemoryClass();
}
// Target 15% of the available RAM.
int size = 1024 * 1024 * memoryClass / 7;
// Bound to max size for mem cache.
return Math.min(size, MAX_MEM_CACHE_SIZE);
}
/**
* Get the current version code number of the app
*
* @return
*/
public static int getApplicationVersionCode(Context c) {
try {
PackageInfo pinfo = c.getPackageManager().getPackageInfo(
c.getPackageName(), 0);
int versionNumber = pinfo.versionCode;
return versionNumber;
} catch (PackageManager.NameNotFoundException e) {
// This exception should never be thrown
e.printStackTrace();
return 0;
}
}
/**
* Get the path to the disk (as {@link java.io.File}), where the disk cache can store his cache entries
*
* @param c
* {@link android.content.Context}
* @return
*/
public static File getDiskCacheDir(Context c) {
File dir = c.getExternalCacheDir();
if (dir == null)
dir = c.getCacheDir();
return dir;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy