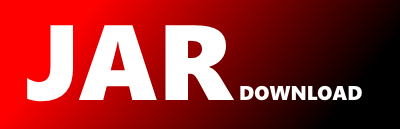
com.hanzhigu.so.utils.ValueUtils Maven / Gradle / Ivy
package com.hanzhigu.so.utils;
import java.lang.reflect.Array;
import java.math.BigDecimal;
import java.util.Collection;
import java.util.Map;
public class ValueUtils {
public static BigDecimal getBigDecimal(BigDecimal num, int scale) {
BigDecimal intNum = new BigDecimal(num.intValue());
if(intNum.compareTo(num) == 0) {
return intNum;
}
return num.setScale(scale,BigDecimal.ROUND_HALF_UP);
}
public static boolean isBoolean(Class> classType) {
if (classType == null) {
return false;
}
return (Boolean.TYPE.equals(classType)) || (Boolean.class.equals(classType));
}
public static boolean isNotEmpty(Object value) {
return !isEmpty(value);
}
public static boolean isEmpty(Object value) {
if (value == null) {
return true;
}
if ((value instanceof String)) {
String text = (String) value;
return (text.isEmpty()) || (text.trim().isEmpty());
}
if (value.getClass().isArray()) {
return Array.getLength(value) == 0;
}
if ((value instanceof Collection)) {
return ((Collection) value).isEmpty();
}
if ((value instanceof Map)) {
return ((Map) value).isEmpty();
}
return false;
}
public static boolean isNumber(Class> classType) {
if (classType == null) {
return false;
}
return (Number.class.isAssignableFrom(classType)) || (Double.TYPE.equals(classType))
|| (Short.TYPE.equals(classType)) || (Integer.TYPE.equals(classType)) || (Float.TYPE.equals(classType));
}
public static boolean isZero(double value) {
return (value == 0.0D) || (Math.abs(value) < 1.E-005D);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy