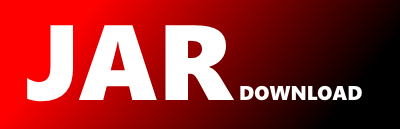
com.haoxuer.discover.common.freemarker.ObjectDirectiveModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of discover-common-hibernate Show documentation
Show all versions of discover-common-hibernate Show documentation
discover-hibernate_common is a lib for hibernate
package com.haoxuer.discover.common.freemarker;
import com.haoxuer.discover.common.utils.DirectiveUtils;
import freemarker.core.Environment;
import freemarker.template.*;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
public abstract class ObjectDirectiveModel implements TemplateDirectiveModel {
private Map map;
public ObjectDirectiveModel() {
}
public void execute(Environment env, Map params, TemplateModel[] templateModels, TemplateDirectiveBody body) throws TemplateException, IOException {
this.map = params;
Map paramWrap = new HashMap(params);
if (this.data() != null) {
paramWrap.put("viewModel", ObjectWrapper.DEFAULT_WRAPPER.wrap(this.data()));
}
Map origMap = DirectiveUtils.addParamsToVariable(env, paramWrap);
if (body != null) {
body.render(env.getOut());
}
DirectiveUtils.removeParamsFromVariable(env, paramWrap, origMap);
}
public abstract Object data();
private Map getParams() {
return this.map;
}
public Integer getInt(String name) {
Integer size = null;
try {
size = DirectiveUtils.getInt(name, this.map);
} catch (Exception var4) {
}
return size;
}
public Integer getInt(String name, Integer num) {
Integer size = null;
try {
size = DirectiveUtils.getInt(name, this.map);
if (size == null) {
size = num;
}
} catch (Exception var5) {
}
return size;
}
public Long getLong(String name, Long num) {
Long size = null;
try {
size = DirectiveUtils.getLong(name, this.map);
if (size == null) {
size = num;
}
} catch (Exception var5) {
}
return size;
}
public String getString(String name, String defaul) {
String result = null;
try {
result = DirectiveUtils.getString(name, this.map);
} catch (Exception var5) {
}
if (defaul == null) {
defaul = "";
}
if (result == null) {
result = defaul;
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy