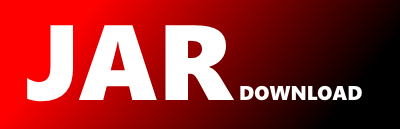
net.glxn.qrgen.core.scheme.GeoInfo Maven / Gradle / Ivy
package net.glxn.qrgen.core.scheme;
import java.util.ArrayList;
import java.util.List;
/**
* Encodes a geographic information, format is:
* geo:40.71872,-73.98905,100
*
*/
public class GeoInfo extends Schema {
public static final String GEO = "geo";
private List points;
/**
* Default constructor to construct new geo info object.
*/
public GeoInfo() {
super();
this.points = new ArrayList();
}
public List getPoints() {
return points;
}
public void setPoints(List points) {
this.points = points;
}
@Override
public Schema parseSchema(String code) {
if (code == null || !code.trim().toLowerCase().startsWith(GEO)) {
throw new IllegalArgumentException("this is not a geo info code: " + code);
}
String[] points = code.trim().toLowerCase().replaceAll(GEO + ":", "").split(",");
if (points != null && points.length > 0) {
for (String point : points) {
this.points.add(point);
}
}
return this;
}
@Override
public String generateString() {
StringBuilder builder = new StringBuilder();
if (points != null) {
int s = points.size();
for (int i = 0; i < s; i++) {
builder.append(points.get(i));
if (i < s - 1) {
builder.append(",");
}
}
}
return GEO + ":" + builder.toString();
}
@Override
public String toString() {
return generateString();
}
public static GeoInfo parse(final String geoInfoCode) {
GeoInfo geoInfo = new GeoInfo();
geoInfo.parseSchema(geoInfoCode);
return geoInfo;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy