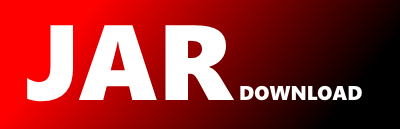
net.glxn.qrgen.core.scheme.Wifi Maven / Gradle / Ivy
package net.glxn.qrgen.core.scheme;
import static net.glxn.qrgen.core.scheme.SchemeUtil.getParameters;
import java.util.Map;
/**
* Encodes a Wifi connection, format is:
* WIFI:T:AUTHENTICATION;S:SSID;P:PSK;H:HIDDEN;
*/
public class Wifi extends Schema {
public static final String WIFI_PROTOCOL_HEADER = "WIFI:";
public static final String AUTHENTICATION = "T";
public static final String SSID = "S";
public static final String PSK = "P";
public static final String HIDDEN = "H";
private String authentication;
private String ssid;
private String psk;
private boolean hidden = false;
public Wifi() {
super();
}
/**
* @return the authentication
*/
public String getAuthentication() {
return authentication;
}
/**
* @param authentication
* the authentication to set
*/
public void setAuthentication(Authentication authentication) {
setAuthentication(authentication.toString());
}
/**
* @param authentication
* the authentication to set
*/
public void setAuthentication(String authentication) {
this.authentication = authentication;
}
/**
* @param authentication
* the authentication to set
*/
public Wifi withAuthentication(Authentication authentication) {
setAuthentication(authentication);
return this;
}
/**
* @return the ssid
*/
public String getSsid() {
return ssid;
}
/**
* @param ssid
* the ssid to set
*/
public void setSsid(String ssid) {
this.ssid = ssid;
}
/**
* @param ssid
* the ssid to set
*/
public Wifi withSsid(String ssid) {
setSsid(ssid);
return this;
}
/**
* @return the psk
*/
public String getPsk() {
return psk;
}
/**
* @param psk
* the psk to set
*/
public void setPsk(String psk) {
this.psk = psk;
}
/**
* @param psk
* the psk to set
*/
public Wifi withPsk(String psk) {
setPsk(psk);
return this;
}
/**
* @return the hidden
*/
public boolean isHidden() {
return hidden;
}
/**
* @param value
* the hidden to set
*/
public void setHidden(final String value) {
setHidden(Boolean.valueOf(value));
}
/**
* @param hidden
* the hidden to set
*/
public void setHidden(boolean hidden) {
this.hidden = hidden;
}
/**
* @param hidden
* the hidden to set
*/
public Wifi withHidden(boolean hidden) {
setHidden(hidden);
return this;
}
public enum Authentication {
WEP, WPA, nopass;
}
@Override
public Schema parseSchema(String code) {
if (code == null || !code.startsWith(WIFI_PROTOCOL_HEADER)) {
throw new IllegalArgumentException("this is not a valid WIFI code: " + code);
}
Map parameters = getParameters(code.substring(WIFI_PROTOCOL_HEADER.length()), "(?
© 2015 - 2025 Weber Informatics LLC | Privacy Policy