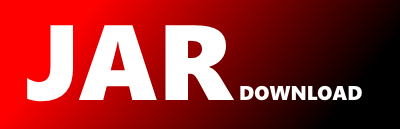
com.haoxuer.discover.notice.data.dao.impl.UserNotificationDaoImpl Maven / Gradle / Ivy
package com.haoxuer.discover.notice.data.dao.impl;
import com.haoxuer.discover.config.data.entity.User;
import com.haoxuer.discover.data.core.CriteriaDaoImpl;
import com.haoxuer.discover.notice.data.dao.UserNotificationDao;
import com.haoxuer.discover.notice.data.dao.UserNotificationMemberDao;
import com.haoxuer.discover.notice.data.entity.UserNotification;
import com.haoxuer.discover.notice.data.entity.UserNotificationMember;
import com.haoxuer.discover.notice.data.enums.NotificationCategory;
import com.haoxuer.discover.notice.data.enums.NotificationState;
import org.hibernate.SessionFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Repository;
/**
* Created by imake on 2018年01月02日11:12:13.
*/
@Repository
public class UserNotificationDaoImpl extends CriteriaDaoImpl implements UserNotificationDao {
@Autowired
private UserNotificationMemberDao notificationMemberDao;
@Override
public UserNotification findById(Long id) {
if (id == null) {
return null;
}
return get(id);
}
@Override
public UserNotification save(UserNotification bean) {
getSession().save(bean);
return bean;
}
@Override
public UserNotification deleteById(Long id) {
UserNotification entity = super.get(id);
if (entity != null) {
getSession().delete(entity);
}
return entity;
}
@Override
public UserNotification send(UserNotification bean, Long... users) {
bean.setCategory(NotificationCategory.single);
save(bean);
if (users != null) {
for (Long user : users) {
UserNotificationMember member = new UserNotificationMember();
member.setNotification(bean);
member.setState(NotificationState.unread);
member.setUser(User.fromId(user));
notificationMemberDao.save(member);
}
}
return bean;
}
@Override
protected Class getEntityClass() {
return UserNotification.class;
}
@Autowired
public void setSuperSessionFactory(SessionFactory sessionFactory) {
super.setSessionFactory(sessionFactory);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy