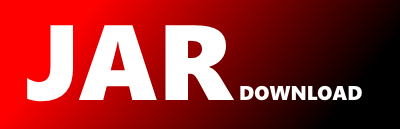
com.harium.database.dao.OrmLiteBaseDAOImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ormlite Show documentation
Show all versions of ormlite Show documentation
OrmLite module to Harium Database
package com.harium.database.dao;
import com.harium.database.DatabaseError;
import com.harium.database.model.BaseDAO;
import com.j256.ormlite.dao.Dao;
import com.j256.ormlite.dao.DaoManager;
import com.j256.ormlite.support.ConnectionSource;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
public class OrmLiteBaseDAOImpl implements BaseDAO {
private Class klass;
protected Dao dao;
public OrmLiteBaseDAOImpl(Class klass) {
this.klass = klass;
}
public void init(ConnectionSource connectionSource) {
try {
dao = DaoManager.createDao(connectionSource, klass);
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public List queryForEq(String column, Object o) throws SQLException {
return dao.queryForEq(column, o);
}
public T queryForId(int id) {
try {
return dao.queryForId(id);
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
public List queryAll() {
try {
return dao.queryForAll();
} catch (SQLException e) {
e.printStackTrace();
}
return new ArrayList();
}
public int create(T item) {
try {
return dao.create(item);
} catch (SQLException e) {
e.printStackTrace();
return DatabaseError.ON_CREATE;
}
}
public int update(T item) {
try {
return dao.update(item);
} catch (SQLException e) {
e.printStackTrace();
return DatabaseError.ON_UPDATE;
}
}
public int createOrUpdate(T item) {
Dao.CreateOrUpdateStatus status = null;
try {
status = dao.createOrUpdate(item);
return status.getNumLinesChanged();
} catch (SQLException e) {
e.printStackTrace();
if (status.isCreated()) {
return DatabaseError.ON_UPDATE;
}
return DatabaseError.ON_CREATE;
}
}
public int delete(T model) {
try {
return dao.delete(model);
} catch (SQLException e) {
e.printStackTrace();
return DatabaseError.ON_DELETE;
}
}
public long count() {
try {
return dao.countOf();
} catch (SQLException e) {
e.printStackTrace();
}
return 0;
}
public Class getKlass() {
return klass;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy