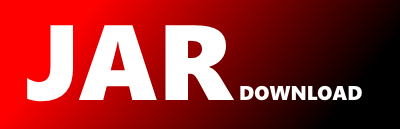
com.hashicorp.nomad.apimodel.JobSummary Maven / Gradle / Ivy
package com.hashicorp.nomad.apimodel;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.hashicorp.nomad.javasdk.ApiObject;
import com.hashicorp.nomad.javasdk.NomadJson;
import java.io.IOException;
import java.math.BigInteger;
import java.util.List;
import java.util.Map;
/**
* This is a generated JavaBean representing a request or response structure.
*
* @see Nomad HTTP API documentation associated with the endpoint you are using.
*/
public final class JobSummary extends ApiObject {
private String jobId;
private String namespace;
private Map summary;
private JobChildrenSummary children;
private BigInteger createIndex;
private BigInteger modifyIndex;
@JsonProperty("JobID")
public String getJobId() {
return jobId;
}
public JobSummary setJobId(String jobId) {
this.jobId = jobId;
return this;
}
@JsonProperty("Namespace")
public String getNamespace() {
return namespace;
}
public JobSummary setNamespace(String namespace) {
this.namespace = namespace;
return this;
}
@JsonProperty("Summary")
public Map getSummary() {
return summary;
}
public JobSummary setSummary(Map summary) {
this.summary = summary;
return this;
}
public JobSummary addSummary(String key, TaskGroupSummary value) {
if (this.summary == null)
this.summary = new java.util.HashMap<>();
this.summary.put(key, value);
return this;
}
@JsonProperty("Children")
public JobChildrenSummary getChildren() {
return children;
}
public JobSummary setChildren(JobChildrenSummary children) {
this.children = children;
return this;
}
@JsonProperty("CreateIndex")
public BigInteger getCreateIndex() {
return createIndex;
}
public JobSummary setCreateIndex(BigInteger createIndex) {
this.createIndex = createIndex;
return this;
}
@JsonProperty("ModifyIndex")
public BigInteger getModifyIndex() {
return modifyIndex;
}
public JobSummary setModifyIndex(BigInteger modifyIndex) {
this.modifyIndex = modifyIndex;
return this;
}
@Override
public String toString() {
return NomadJson.serialize(this);
}
public static JobSummary fromJson(String json) throws IOException {
return NomadJson.deserialize(json, JobSummary.class);
}
public static List fromJsonArray(String json) throws IOException {
return NomadJson.deserializeList(json, JobSummary.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy