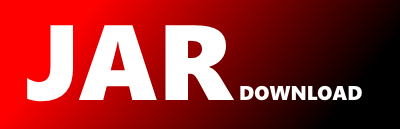
com.hashicorp.nomad.apimodel.Node Maven / Gradle / Ivy
package com.hashicorp.nomad.apimodel;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.hashicorp.nomad.javasdk.ApiObject;
import com.hashicorp.nomad.javasdk.NomadJson;
import java.io.IOException;
import java.math.BigInteger;
import java.util.List;
import java.util.Map;
/**
* This is a generated JavaBean representing a request or response structure.
*
* @see Nomad HTTP API documentation associated with the endpoint you are using.
*/
public final class Node extends ApiObject {
private String id;
private String datacenter;
private String name;
private String httpAddr;
private boolean tlsEnabled;
private Map attributes;
private Resources resources;
private Resources reserved;
private Map links;
private Map meta;
private String nodeClass;
private boolean drain;
private String status;
private String statusDescription;
private long statusUpdatedAt;
private BigInteger createIndex;
private BigInteger modifyIndex;
@JsonProperty("ID")
public String getId() {
return id;
}
public Node setId(String id) {
this.id = id;
return this;
}
@JsonProperty("Datacenter")
public String getDatacenter() {
return datacenter;
}
public Node setDatacenter(String datacenter) {
this.datacenter = datacenter;
return this;
}
@JsonProperty("Name")
public String getName() {
return name;
}
public Node setName(String name) {
this.name = name;
return this;
}
@JsonProperty("HTTPAddr")
public String getHttpAddr() {
return httpAddr;
}
public Node setHttpAddr(String httpAddr) {
this.httpAddr = httpAddr;
return this;
}
@JsonProperty("TLSEnabled")
public boolean getTlsEnabled() {
return tlsEnabled;
}
public Node setTlsEnabled(boolean tlsEnabled) {
this.tlsEnabled = tlsEnabled;
return this;
}
@JsonProperty("Attributes")
public Map getAttributes() {
return attributes;
}
public Node setAttributes(Map attributes) {
this.attributes = attributes;
return this;
}
public Node addAttributes(String key, String value) {
if (this.attributes == null)
this.attributes = new java.util.HashMap<>();
this.attributes.put(key, value);
return this;
}
@JsonProperty("Resources")
public Resources getResources() {
return resources;
}
public Node setResources(Resources resources) {
this.resources = resources;
return this;
}
@JsonProperty("Reserved")
public Resources getReserved() {
return reserved;
}
public Node setReserved(Resources reserved) {
this.reserved = reserved;
return this;
}
@JsonProperty("Links")
public Map getLinks() {
return links;
}
public Node setLinks(Map links) {
this.links = links;
return this;
}
public Node addLinks(String key, String value) {
if (this.links == null)
this.links = new java.util.HashMap<>();
this.links.put(key, value);
return this;
}
@JsonProperty("Meta")
public Map getMeta() {
return meta;
}
public Node setMeta(Map meta) {
this.meta = meta;
return this;
}
public Node addMeta(String key, String value) {
if (this.meta == null)
this.meta = new java.util.HashMap<>();
this.meta.put(key, value);
return this;
}
@JsonProperty("NodeClass")
public String getNodeClass() {
return nodeClass;
}
public Node setNodeClass(String nodeClass) {
this.nodeClass = nodeClass;
return this;
}
@JsonProperty("Drain")
public boolean getDrain() {
return drain;
}
public Node setDrain(boolean drain) {
this.drain = drain;
return this;
}
@JsonProperty("Status")
public String getStatus() {
return status;
}
public Node setStatus(String status) {
this.status = status;
return this;
}
@JsonProperty("StatusDescription")
public String getStatusDescription() {
return statusDescription;
}
public Node setStatusDescription(String statusDescription) {
this.statusDescription = statusDescription;
return this;
}
@JsonProperty("StatusUpdatedAt")
public long getStatusUpdatedAt() {
return statusUpdatedAt;
}
public Node setStatusUpdatedAt(long statusUpdatedAt) {
this.statusUpdatedAt = statusUpdatedAt;
return this;
}
@JsonProperty("CreateIndex")
public BigInteger getCreateIndex() {
return createIndex;
}
public Node setCreateIndex(BigInteger createIndex) {
this.createIndex = createIndex;
return this;
}
@JsonProperty("ModifyIndex")
public BigInteger getModifyIndex() {
return modifyIndex;
}
public Node setModifyIndex(BigInteger modifyIndex) {
this.modifyIndex = modifyIndex;
return this;
}
@Override
public String toString() {
return NomadJson.serialize(this);
}
public static Node fromJson(String json) throws IOException {
return NomadJson.deserialize(json, Node.class);
}
public static List fromJsonArray(String json) throws IOException {
return NomadJson.deserializeList(json, Node.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy