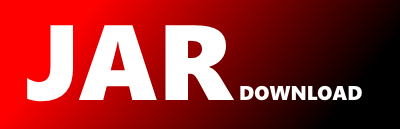
com.hashicorp.nomad.javasdk.AgentApi Maven / Gradle / Ivy
package com.hashicorp.nomad.javasdk;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.hashicorp.nomad.apimodel.AgentHealthResponse;
import com.hashicorp.nomad.apimodel.AgentSelf;
import com.hashicorp.nomad.apimodel.ServerMembers;
import org.apache.http.client.utils.URIBuilder;
import javax.annotation.Nonnull;
import java.io.IOException;
import java.util.Arrays;
import java.util.List;
/**
* API for Nomad agent and cluster management,
* exposing the functionality of the {@code /v1/agent/…} endpoints of the
* Nomad HTTP API.
*/
public class AgentApi extends ApiBase {
AgentApi(final NomadApiClient apiClient) {
super(apiClient);
}
/**
* Performs a basic healthcheck.
*
* @throws IOException if there is an HTTP or lower-level problem
* @throws NomadException if the response signals an error or cannot be deserialized
* @see {@code GET /v1/agent/health}
*/
@Nonnull
public final NomadResponse health() throws IOException, NomadException {
return executePlain(get("/v1/agent/health"), NomadJson.parserFor(AgentHealthResponse.class));
}
/**
* Queries for information about the agent we are connected to.
*
* @throws IOException if there is an HTTP or lower-level problem
* @throws NomadException if the response signals an error or cannot be deserialized
* @see {@code GET /v1/agent/self}
*/
@Nonnull
public final NomadResponse self() throws IOException, NomadException {
return executePlain(get("/v1/agent/self"), NomadJson.parserFor(AgentSelf.class));
}
/**
* Queries for the known peers in the gossip pool.
*
* @throws IOException if there is an HTTP or lower-level problem
* @throws NomadException if the response signals an error or cannot be deserialized
* @see {@code GET /v1/agent/members}
*/
@Nonnull
public final NomadResponse members() throws IOException, NomadException {
return executePlain(get("/v1/agent/members"), NomadJson.parserFor(ServerMembers.class));
}
/**
* Forces a member of the gossip pool from the "failed" state into the "left" state.
*
* @param nodeName the name of the node to force out of the pool
* @throws IOException if there is an HTTP or lower-level problem
* @throws NomadException if the response signals an error or cannot be deserialized
* @see {@code PUT /v1/agent/force-leave}
*/
@Nonnull
public final NomadResponse forceLeave(String nodeName) throws IOException, NomadException {
return executePlain(put(uri("/v1/agent/force-leave").addParameter("node", nodeName), null), null);
}
/**
* Queries an agent in client mode for its list of known servers.
*
* @throws IOException if there is an HTTP or lower-level problem
* @throws NomadException if the response signals an error or cannot be deserialized
* @see {@code GET /v1/agent/servers}
*/
@Nonnull
public final NomadResponse> servers() throws IOException, NomadException {
return executePlain(get("/v1/agent/servers"), NomadJson.parserForListOf(String.class));
}
/**
* Updates the list of known servers to the given addresses, replacing all previous addresses.
*
* @param addresses the server addresses
* @throws IOException if there is an HTTP or lower-level problem
* @throws NomadException if the response signals an error or cannot be deserialized
* @see {@code PUT /v1/agent/servers}
*/
@Nonnull
public final NomadResponse setServers(String... addresses) throws IOException, NomadException {
return setServers(Arrays.asList(addresses));
}
/**
* Updates the list of known servers to the given addresses, replacing all previous addresses.
*
* @param addresses the server addresses
* @throws IOException if there is an HTTP or lower-level problem
* @throws NomadException if the response signals an error or cannot be deserialized
* @see {@code PUT /v1/agent/servers}
*/
@Nonnull
public final NomadResponse setServers(Iterable addresses) throws IOException, NomadException {
final URIBuilder uri = uri("/v1/agent/servers");
for (String address : addresses)
uri.addParameter("address", address);
return executePlain(put(uri, null), null);
}
/**
* Causes the agent to join a cluster by joining the gossip pool at one of the given addresses.
*
* @param addresses the addresses to try joining
* @throws IOException if there is an HTTP or lower-level problem
* @throws NomadException if the response signals an error or cannot be deserialized
* @see {@code PUT /v1/agent/join}
*/
@Nonnull
public final NomadResponse join(String... addresses) throws IOException, NomadException {
return join(Arrays.asList(addresses));
}
/**
* Causes the agent to join a cluster by joining the gossip pool at one of the given addresses.
*
* @param addresses the addresses to try joining
* @throws IOException if there is an HTTP or lower-level problem
* @throws NomadException if the response signals an error or cannot be deserialized
* @see {@code PUT /v1/agent/join}
*/
@Nonnull
public final NomadResponse join(Iterable addresses) throws IOException, NomadException {
final URIBuilder uri = uri("/v1/agent/join");
for (String address : addresses)
uri.addParameter("address", address);
return executePlain(put(uri, null), new JoinResponseValueExtractor());
}
/**
* Private type representing raw responses from the /v1/agent/join endpoint.
*/
private static final class JoinResponse {
@JsonProperty("error")
private String error;
@JsonProperty("num_joined")
private int numberJoined;
}
/**
* A value extractor that converts a @{link JoinResponse} into an Integer,
* or throwing an exception if it signals an error.
*/
private static class JoinResponseValueExtractor implements ValueExtractor {
private final JsonParser parser = NomadJson.parserFor(JoinResponse.class);
@Override
public Integer extractValue(String json) throws ResponseParsingException, ErrorFoundInResponseEntityException {
JoinResponse joinResponse = parser.extractValue(json);
if (joinResponse.error != null && !joinResponse.error.isEmpty())
throw new ErrorFoundInResponseEntityException(joinResponse.error);
return joinResponse.numberJoined;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy