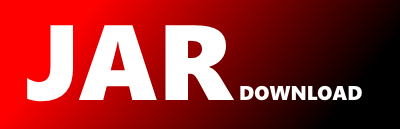
com.hashicorp.nomad.javasdk.NomadPredicates Maven / Gradle / Ivy
package com.hashicorp.nomad.javasdk;
import com.hashicorp.nomad.apimodel.Evaluation;
import com.hashicorp.nomad.apimodel.Job;
import com.hashicorp.nomad.apimodel.NodeListStub;
import javax.annotation.Nonnull;
import java.util.List;
/**
* Predicates for use with {@link QueryOptions} to poll until a condition is met.
*/
public abstract class NomadPredicates {
/**
* Returns a predicate that checks whether the server had a known leader when responding.
*
* @param the response value type of the request this predicate will be used with
*/
public static Predicate> hadKnownLeader() {
return new Predicate>() {
@Override
public boolean apply(ServerQueryResponse response) {
return response.hadKnownLeader();
}
};
}
/**
* Transforms a predicate on a value of type T into a predicate on a {@code ServerQueryResponse}.
*
* @param valuePredicate the predicate for the response value
* @param the response value type of the request this predicate will be used with
*/
public static Predicate> responseValue(final Predicate valuePredicate) {
return new Predicate>() {
@Override
public boolean apply(ServerQueryResponse value) {
return valuePredicate.apply(value.getValue());
}
};
}
/**
* Returns a predicate that checks if a list is empty.
*
* @param the element type of the list type this predicate will be used with
*/
public static Predicate> nonEmpty() {
return new Predicate>() {
@Override
public boolean apply(@Nonnull List collection) {
return !collection.isEmpty();
}
};
}
/**
* Returns a predicate that checks if an evaluation has the given status.
*
* @param status the expected status
*/
public static Predicate evaluationHasStatus(final String status) {
return new Predicate() {
@Override
public boolean apply(@Nonnull Evaluation evaluation) {
return status.equals(evaluation.getStatus());
}
};
}
/**
* Returns a predicate that checks if an evaluation has completed.
*/
public static Predicate evaluationHasCompleted() {
return evaluationHasStatus("complete");
}
/**
* Returns a predicate that checks if a job has the given status.
*
* @param status the expected status
*/
public static Predicate jobHasStatus(final String status) {
return new Predicate() {
@Override
public boolean apply(Job job) {
return status.equals(job.getStatus());
}
};
}
/**
* Returns a predicate that checks if a job has completed.
*/
public static Predicate jobHasCompleted() {
return jobHasStatus("complete");
}
/**
* Returns a predicate that checks if the given client node is ready.
*
* @param name the client's node name
*/
public static Predicate> clientNodeIsReady(final String name) {
return new Predicate>() {
@Override
public boolean apply(List nodes) {
for (NodeListStub node : nodes)
if (name.equals(node.getName()))
return "ready".equals(node.getStatus());
return false;
}
};
}
/**
* Returns a predicate that is true when both of the given predicates is true.
*
* @param a one of the predicates in the conjunction
* @param b one of the predicates in the conjunction
* @param the type this predicate will be used with
*/
public static Predicate both(final Predicate super T> a, final Predicate super T> b) {
return new Predicate() {
@Override
public boolean apply(T value) {
return a.apply(value) && b.apply(value);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy