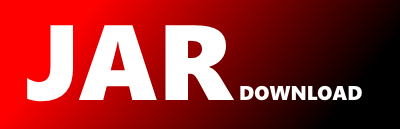
com.hashicorp.nomad.javasdk.QueryOptions Maven / Gradle / Ivy
package com.hashicorp.nomad.javasdk;
import javax.annotation.Nullable;
import java.math.BigInteger;
/**
* Options that control how an operation that queries a Nomad server is performed.
*
* @param the response value type of the request these options will be used with
*/
public class QueryOptions {
@Nullable private String region;
@Nullable private String namespace;
@Nullable private BigInteger index;
@Nullable private WaitStrategy waitStrategy;
private boolean allowStale;
@Nullable private Predicate> repeatedPollPredicate;
@Nullable private String authToken;
/**
* Gets the region to which requests should be forwarded.
*
* @return the region the request should be routed to,
* or null if the API client's default region configuration should be used.
* @see Cross-Region Requests
*/
@Nullable
public String getRegion() {
return region;
}
/**
* Sets the region to which the request should be forwarded.
*
* @param region the region the request should be routed to,
* or null if the API client's default region configuration should be used.
* @return this QueryOptions instance.
* @see Cross-Region Requests
*/
public QueryOptions setRegion(@Nullable String region) {
this.region = region;
return this;
}
/**
* Gets the namespace for this request.
*
* When null, falls back to the NomadApiClient's namespace.
*/
@Nullable
public String getNamespace() {
return namespace;
}
/**
* Sets the namespace for this request.
*
* When null, falls back to the NomadApiClient's namespace.
*
* @param namespace the namespace to use
*/
public QueryOptions setNamespace(@Nullable String namespace) {
this.namespace = namespace;
return this;
}
/**
* Gets the long-polling query index to use.
*
* @return the index to use
* @see Blocking Queries
*/
public BigInteger getIndex() {
return index;
}
/**
* Sets the long-polling query index.
*
* The index is usually from a previous previous response's {@link ServerResponse#getIndex()} method.
* This will cause the request to block until there is a potential change in the response.
*
* @param index the index to use
* @return this QueryOptions instance.
* @see Blocking Queries
*/
public QueryOptions setIndex(BigInteger index) {
this.index = index;
return this;
}
/**
* Gets the wait strategy to use.
*
* @return the wait strategy to use, or null if a simple non-blocking request should be performed.
* @see Blocking Queries
*/
@Nullable
public WaitStrategy getWaitStrategy() {
return waitStrategy;
}
/**
* Sets the wait strategy to use.
*
* The wait strategy is used to determine the maximum amount of time to wait for a long-poll to complete.
*
* @param waitStrategy the wait strategy to use, or null if a simple non-blocking request should be performed.
* @return this QueryOptions instance.
* @see Blocking Queries
*/
public QueryOptions setWaitStrategy(WaitStrategy waitStrategy) {
this.waitStrategy = waitStrategy;
return this;
}
/**
* Gets whether to allow stale responses.
*
* @return true if state responses are acceptable, false if they are not.
* @see Consistency Modes
*/
public boolean isAllowStale() {
return allowStale;
}
/**
* Sets whether to allow stale responses.
*
* When true, non-leader nodes will answer from their own state, which might be slightly behind the leader.
* You can check the response's {@link ServerQueryResponse#getMillisSinceLastContact()} to gauge the staleness of
* the result.
*
* @param allowStale true if state responses are acceptable, false if they are not.
* @return this QueryOptions instance.
* @see Consistency Modes
*/
public QueryOptions setAllowStale(boolean allowStale) {
this.allowStale = allowStale;
return this;
}
/**
* Gets a response predicate that we will try to satisfy
* by repeatedly polling the server until the predicate is true or the WaitStrategy times out.
*
* @return the predicate to statisfy, or null if we should accept whatever is first returned
*/
@Nullable
public Predicate> getRepeatedPollPredicate() {
return repeatedPollPredicate;
}
/**
* Sets a response predicate that we will try to satisfy
* by repeatedly polling the server until the predicate is true or the WaitStrategy times out.
*
* @param repeatedPollPredicate the predicate to statisfy, or null if we should accept whatever is first returned
* @return this QueryOptions instance.
*/
public QueryOptions setRepeatedPollPredicate(@Nullable Predicate> repeatedPollPredicate) {
this.repeatedPollPredicate = repeatedPollPredicate;
return this;
}
/**
* Returns query options that cause us to poll hoping for a response that is newer than a previous reponse.
*
* @param previousResponse a response whose index should be used in the query options
* @param the response value type of the request these options will be used with
* @return QueryOptions with the index set to the index of the previous response
* @see Blocking Queries
*/
public static QueryOptions newerThan(ServerQueryResponse previousResponse) {
return new QueryOptions().setIndex(previousResponse == null ? null : previousResponse.getIndex());
}
/**
* Returns query options that will poll repeatedly until a condition is met.
*
* @param predicate the condition to statisfy
* @param waitStrategy the wait strategy to use
* @param the response value type of the request these options will be used with
* @return QueryOptions with the given predicate and wait strategy set
*/
public static QueryOptions pollRepeatedlyUntil(Predicate> predicate,
WaitStrategy waitStrategy) {
return new QueryOptions()
.setRepeatedPollPredicate(predicate)
.setWaitStrategy(waitStrategy);
}
/**
* Gets the secret ID of the ACL token to use for this request.
*
* When null, falls back to the NomadApiClient's auth token.
*/
@Nullable
public String getAuthToken() {
return authToken;
}
/**
* Sets the secret ID of the ACL token to use for this request.
*
* When null, falls back to the NomadApiClient's auth token.
*
* @param authToken the secret ID
*/
public QueryOptions setAuthToken(@Nullable String authToken) {
this.authToken = authToken;
return this;
}
}