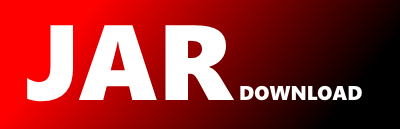
com.hashicorp.cdktf.DataTerraformRemoteStateCos Maven / Gradle / Ivy
package com.hashicorp.cdktf;
/**
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.79.0 (build b22f628)", date = "2023-07-26T11:31:27.502Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Jsii(module = com.hashicorp.cdktf.$Module.class, fqn = "cdktf.DataTerraformRemoteStateCos")
public class DataTerraformRemoteStateCos extends com.hashicorp.cdktf.TerraformRemoteState {
protected DataTerraformRemoteStateCos(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected DataTerraformRemoteStateCos(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
* @param config This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public DataTerraformRemoteStateCos(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull com.hashicorp.cdktf.DataTerraformRemoteStateCosConfig config) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(config, "config is required") });
}
/**
* (experimental) A fluent builder for {@link com.hashicorp.cdktf.DataTerraformRemoteStateCos}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private final com.hashicorp.cdktf.DataTerraformRemoteStateCosConfig.Builder config;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.config = new com.hashicorp.cdktf.DataTerraformRemoteStateCosConfig.Builder();
}
/**
* @return {@code this}
* @param defaults This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder defaults(final java.util.Map defaults) {
this.config.defaults(defaults);
return this;
}
/**
* @return {@code this}
* @param workspace This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder workspace(final java.lang.String workspace) {
this.config.workspace(workspace);
return this;
}
/**
* (experimental) (Required) The name of the COS bucket.
*
* You shall manually create it first.
*
* @return {@code this}
* @param bucket (Required) The name of the COS bucket. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder bucket(final java.lang.String bucket) {
this.config.bucket(bucket);
return this;
}
/**
* (experimental) (Optional) Object ACL to be applied to the state file, allows private and public-read.
*
* Defaults to private.
*
* @return {@code this}
* @param acl (Optional) Object ACL to be applied to the state file, allows private and public-read. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder acl(final java.lang.String acl) {
this.config.acl(acl);
return this;
}
/**
* (experimental) (Optional) Whether to enable server side encryption of the state file.
*
* If it is true, COS will use 'AES256' encryption algorithm to encrypt state file.
*
* @return {@code this}
* @param encrypt (Optional) Whether to enable server side encryption of the state file. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder encrypt(final java.lang.Boolean encrypt) {
this.config.encrypt(encrypt);
return this;
}
/**
* (experimental) (Optional) The path for saving the state file in bucket.
*
* Defaults to terraform.tfstate.
*
* @return {@code this}
* @param key (Optional) The path for saving the state file in bucket. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder key(final java.lang.String key) {
this.config.key(key);
return this;
}
/**
* (experimental) (Optional) The directory for saving the state file in bucket.
*
* Default to "env:".
*
* @return {@code this}
* @param prefix (Optional) The directory for saving the state file in bucket. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder prefix(final java.lang.String prefix) {
this.config.prefix(prefix);
return this;
}
/**
* (experimental) (Optional) The region of the COS bucket.
*
* It supports environment variables TENCENTCLOUD_REGION.
*
* @return {@code this}
* @param region (Optional) The region of the COS bucket. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder region(final java.lang.String region) {
this.config.region(region);
return this;
}
/**
* (experimental) (Optional) Secret id of Tencent Cloud.
*
* It supports environment variables TENCENTCLOUD_SECRET_ID.
*
* @return {@code this}
* @param secretId (Optional) Secret id of Tencent Cloud. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder secretId(final java.lang.String secretId) {
this.config.secretId(secretId);
return this;
}
/**
* (experimental) (Optional) Secret key of Tencent Cloud.
*
* It supports environment variables TENCENTCLOUD_SECRET_KEY.
*
* @return {@code this}
* @param secretKey (Optional) Secret key of Tencent Cloud. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder secretKey(final java.lang.String secretKey) {
this.config.secretKey(secretKey);
return this;
}
/**
* @returns a newly built instance of {@link com.hashicorp.cdktf.DataTerraformRemoteStateCos}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public com.hashicorp.cdktf.DataTerraformRemoteStateCos build() {
return new com.hashicorp.cdktf.DataTerraformRemoteStateCos(
this.scope,
this.id,
this.config.build()
);
}
}
}