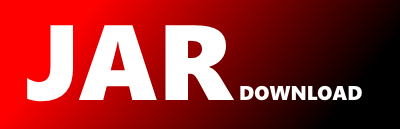
com.hashicorp.cdktf.HttpBackendConfig Maven / Gradle / Ivy
Show all versions of cdktf Show documentation
package com.hashicorp.cdktf;
/**
* (experimental) Stores the state using a simple REST client.
*
* State will be fetched via GET, updated via POST, and purged with DELETE.
* The method used for updating is configurable.
*
* This backend optionally supports state locking.
* When locking support is enabled it will use LOCK and UNLOCK requests providing the lock info in the body.
* The endpoint should return a 423: Locked or 409: Conflict with the holding lock info when
* it's already taken, 200: OK for success. Any other status will be considered an error.
* The ID of the holding lock info will be added as a query parameter to state updates requests.
*
* Read more about this backend in the Terraform docs:
* https://developer.hashicorp.com/terraform/language/settings/backends/http
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.79.0 (build b22f628)", date = "2023-07-26T11:31:27.554Z")
@software.amazon.jsii.Jsii(module = com.hashicorp.cdktf.$Module.class, fqn = "cdktf.HttpBackendConfig")
@software.amazon.jsii.Jsii.Proxy(HttpBackendConfig.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public interface HttpBackendConfig extends software.amazon.jsii.JsiiSerializable {
/**
* (experimental) (Required) The address of the REST endpoint.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@org.jetbrains.annotations.NotNull java.lang.String getAddress();
/**
* (experimental) (Optional) The address of the lock REST endpoint.
*
* Defaults to disabled.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getLockAddress() {
return null;
}
/**
* (experimental) (Optional) The HTTP method to use when locking.
*
* Defaults to LOCK.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getLockMethod() {
return null;
}
/**
* (experimental) (Optional) The password for HTTP basic authentication.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getPassword() {
return null;
}
/**
* (experimental) (Optional) The number of HTTP request retries.
*
* Defaults to 2.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.Number getRetryMax() {
return null;
}
/**
* (experimental) (Optional) The maximum time in seconds to wait between HTTP request attempts.
*
* Defaults to 30.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.Number getRetryWaitMax() {
return null;
}
/**
* (experimental) (Optional) The minimum time in seconds to wait between HTTP request attempts.
*
* Defaults to 1.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.Number getRetryWaitMin() {
return null;
}
/**
* (experimental) (Optional) Whether to skip TLS verification.
*
* Defaults to false.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getSkipCertVerification() {
return null;
}
/**
* (experimental) (Optional) The address of the unlock REST endpoint.
*
* Defaults to disabled.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getUnlockAddress() {
return null;
}
/**
* (experimental) (Optional) The HTTP method to use when unlocking.
*
* Defaults to UNLOCK.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getUnlockMethod() {
return null;
}
/**
* (experimental) (Optional) HTTP method to use when updating state.
*
* Defaults to POST.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getUpdateMethod() {
return null;
}
/**
* (experimental) (Optional) The username for HTTP basic authentication.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getUsername() {
return null;
}
/**
* @return a {@link Builder} of {@link HttpBackendConfig}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link HttpBackendConfig}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String address;
java.lang.String lockAddress;
java.lang.String lockMethod;
java.lang.String password;
java.lang.Number retryMax;
java.lang.Number retryWaitMax;
java.lang.Number retryWaitMin;
java.lang.Boolean skipCertVerification;
java.lang.String unlockAddress;
java.lang.String unlockMethod;
java.lang.String updateMethod;
java.lang.String username;
/**
* Sets the value of {@link HttpBackendConfig#getAddress}
* @param address (Required) The address of the REST endpoint. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder address(java.lang.String address) {
this.address = address;
return this;
}
/**
* Sets the value of {@link HttpBackendConfig#getLockAddress}
* @param lockAddress (Optional) The address of the lock REST endpoint.
* Defaults to disabled.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder lockAddress(java.lang.String lockAddress) {
this.lockAddress = lockAddress;
return this;
}
/**
* Sets the value of {@link HttpBackendConfig#getLockMethod}
* @param lockMethod (Optional) The HTTP method to use when locking.
* Defaults to LOCK.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder lockMethod(java.lang.String lockMethod) {
this.lockMethod = lockMethod;
return this;
}
/**
* Sets the value of {@link HttpBackendConfig#getPassword}
* @param password (Optional) The password for HTTP basic authentication.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder password(java.lang.String password) {
this.password = password;
return this;
}
/**
* Sets the value of {@link HttpBackendConfig#getRetryMax}
* @param retryMax (Optional) The number of HTTP request retries.
* Defaults to 2.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder retryMax(java.lang.Number retryMax) {
this.retryMax = retryMax;
return this;
}
/**
* Sets the value of {@link HttpBackendConfig#getRetryWaitMax}
* @param retryWaitMax (Optional) The maximum time in seconds to wait between HTTP request attempts.
* Defaults to 30.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder retryWaitMax(java.lang.Number retryWaitMax) {
this.retryWaitMax = retryWaitMax;
return this;
}
/**
* Sets the value of {@link HttpBackendConfig#getRetryWaitMin}
* @param retryWaitMin (Optional) The minimum time in seconds to wait between HTTP request attempts.
* Defaults to 1.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder retryWaitMin(java.lang.Number retryWaitMin) {
this.retryWaitMin = retryWaitMin;
return this;
}
/**
* Sets the value of {@link HttpBackendConfig#getSkipCertVerification}
* @param skipCertVerification (Optional) Whether to skip TLS verification.
* Defaults to false.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder skipCertVerification(java.lang.Boolean skipCertVerification) {
this.skipCertVerification = skipCertVerification;
return this;
}
/**
* Sets the value of {@link HttpBackendConfig#getUnlockAddress}
* @param unlockAddress (Optional) The address of the unlock REST endpoint.
* Defaults to disabled.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder unlockAddress(java.lang.String unlockAddress) {
this.unlockAddress = unlockAddress;
return this;
}
/**
* Sets the value of {@link HttpBackendConfig#getUnlockMethod}
* @param unlockMethod (Optional) The HTTP method to use when unlocking.
* Defaults to UNLOCK.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder unlockMethod(java.lang.String unlockMethod) {
this.unlockMethod = unlockMethod;
return this;
}
/**
* Sets the value of {@link HttpBackendConfig#getUpdateMethod}
* @param updateMethod (Optional) HTTP method to use when updating state.
* Defaults to POST.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder updateMethod(java.lang.String updateMethod) {
this.updateMethod = updateMethod;
return this;
}
/**
* Sets the value of {@link HttpBackendConfig#getUsername}
* @param username (Optional) The username for HTTP basic authentication.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder username(java.lang.String username) {
this.username = username;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link HttpBackendConfig}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public HttpBackendConfig build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link HttpBackendConfig}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements HttpBackendConfig {
private final java.lang.String address;
private final java.lang.String lockAddress;
private final java.lang.String lockMethod;
private final java.lang.String password;
private final java.lang.Number retryMax;
private final java.lang.Number retryWaitMax;
private final java.lang.Number retryWaitMin;
private final java.lang.Boolean skipCertVerification;
private final java.lang.String unlockAddress;
private final java.lang.String unlockMethod;
private final java.lang.String updateMethod;
private final java.lang.String username;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.address = software.amazon.jsii.Kernel.get(this, "address", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.lockAddress = software.amazon.jsii.Kernel.get(this, "lockAddress", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.lockMethod = software.amazon.jsii.Kernel.get(this, "lockMethod", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.password = software.amazon.jsii.Kernel.get(this, "password", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.retryMax = software.amazon.jsii.Kernel.get(this, "retryMax", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.retryWaitMax = software.amazon.jsii.Kernel.get(this, "retryWaitMax", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.retryWaitMin = software.amazon.jsii.Kernel.get(this, "retryWaitMin", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.skipCertVerification = software.amazon.jsii.Kernel.get(this, "skipCertVerification", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.unlockAddress = software.amazon.jsii.Kernel.get(this, "unlockAddress", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.unlockMethod = software.amazon.jsii.Kernel.get(this, "unlockMethod", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.updateMethod = software.amazon.jsii.Kernel.get(this, "updateMethod", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.username = software.amazon.jsii.Kernel.get(this, "username", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.address = java.util.Objects.requireNonNull(builder.address, "address is required");
this.lockAddress = builder.lockAddress;
this.lockMethod = builder.lockMethod;
this.password = builder.password;
this.retryMax = builder.retryMax;
this.retryWaitMax = builder.retryWaitMax;
this.retryWaitMin = builder.retryWaitMin;
this.skipCertVerification = builder.skipCertVerification;
this.unlockAddress = builder.unlockAddress;
this.unlockMethod = builder.unlockMethod;
this.updateMethod = builder.updateMethod;
this.username = builder.username;
}
@Override
public final java.lang.String getAddress() {
return this.address;
}
@Override
public final java.lang.String getLockAddress() {
return this.lockAddress;
}
@Override
public final java.lang.String getLockMethod() {
return this.lockMethod;
}
@Override
public final java.lang.String getPassword() {
return this.password;
}
@Override
public final java.lang.Number getRetryMax() {
return this.retryMax;
}
@Override
public final java.lang.Number getRetryWaitMax() {
return this.retryWaitMax;
}
@Override
public final java.lang.Number getRetryWaitMin() {
return this.retryWaitMin;
}
@Override
public final java.lang.Boolean getSkipCertVerification() {
return this.skipCertVerification;
}
@Override
public final java.lang.String getUnlockAddress() {
return this.unlockAddress;
}
@Override
public final java.lang.String getUnlockMethod() {
return this.unlockMethod;
}
@Override
public final java.lang.String getUpdateMethod() {
return this.updateMethod;
}
@Override
public final java.lang.String getUsername() {
return this.username;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("address", om.valueToTree(this.getAddress()));
if (this.getLockAddress() != null) {
data.set("lockAddress", om.valueToTree(this.getLockAddress()));
}
if (this.getLockMethod() != null) {
data.set("lockMethod", om.valueToTree(this.getLockMethod()));
}
if (this.getPassword() != null) {
data.set("password", om.valueToTree(this.getPassword()));
}
if (this.getRetryMax() != null) {
data.set("retryMax", om.valueToTree(this.getRetryMax()));
}
if (this.getRetryWaitMax() != null) {
data.set("retryWaitMax", om.valueToTree(this.getRetryWaitMax()));
}
if (this.getRetryWaitMin() != null) {
data.set("retryWaitMin", om.valueToTree(this.getRetryWaitMin()));
}
if (this.getSkipCertVerification() != null) {
data.set("skipCertVerification", om.valueToTree(this.getSkipCertVerification()));
}
if (this.getUnlockAddress() != null) {
data.set("unlockAddress", om.valueToTree(this.getUnlockAddress()));
}
if (this.getUnlockMethod() != null) {
data.set("unlockMethod", om.valueToTree(this.getUnlockMethod()));
}
if (this.getUpdateMethod() != null) {
data.set("updateMethod", om.valueToTree(this.getUpdateMethod()));
}
if (this.getUsername() != null) {
data.set("username", om.valueToTree(this.getUsername()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("cdktf.HttpBackendConfig"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
HttpBackendConfig.Jsii$Proxy that = (HttpBackendConfig.Jsii$Proxy) o;
if (!address.equals(that.address)) return false;
if (this.lockAddress != null ? !this.lockAddress.equals(that.lockAddress) : that.lockAddress != null) return false;
if (this.lockMethod != null ? !this.lockMethod.equals(that.lockMethod) : that.lockMethod != null) return false;
if (this.password != null ? !this.password.equals(that.password) : that.password != null) return false;
if (this.retryMax != null ? !this.retryMax.equals(that.retryMax) : that.retryMax != null) return false;
if (this.retryWaitMax != null ? !this.retryWaitMax.equals(that.retryWaitMax) : that.retryWaitMax != null) return false;
if (this.retryWaitMin != null ? !this.retryWaitMin.equals(that.retryWaitMin) : that.retryWaitMin != null) return false;
if (this.skipCertVerification != null ? !this.skipCertVerification.equals(that.skipCertVerification) : that.skipCertVerification != null) return false;
if (this.unlockAddress != null ? !this.unlockAddress.equals(that.unlockAddress) : that.unlockAddress != null) return false;
if (this.unlockMethod != null ? !this.unlockMethod.equals(that.unlockMethod) : that.unlockMethod != null) return false;
if (this.updateMethod != null ? !this.updateMethod.equals(that.updateMethod) : that.updateMethod != null) return false;
return this.username != null ? this.username.equals(that.username) : that.username == null;
}
@Override
public final int hashCode() {
int result = this.address.hashCode();
result = 31 * result + (this.lockAddress != null ? this.lockAddress.hashCode() : 0);
result = 31 * result + (this.lockMethod != null ? this.lockMethod.hashCode() : 0);
result = 31 * result + (this.password != null ? this.password.hashCode() : 0);
result = 31 * result + (this.retryMax != null ? this.retryMax.hashCode() : 0);
result = 31 * result + (this.retryWaitMax != null ? this.retryWaitMax.hashCode() : 0);
result = 31 * result + (this.retryWaitMin != null ? this.retryWaitMin.hashCode() : 0);
result = 31 * result + (this.skipCertVerification != null ? this.skipCertVerification.hashCode() : 0);
result = 31 * result + (this.unlockAddress != null ? this.unlockAddress.hashCode() : 0);
result = 31 * result + (this.unlockMethod != null ? this.unlockMethod.hashCode() : 0);
result = 31 * result + (this.updateMethod != null ? this.updateMethod.hashCode() : 0);
result = 31 * result + (this.username != null ? this.username.hashCode() : 0);
return result;
}
}
}