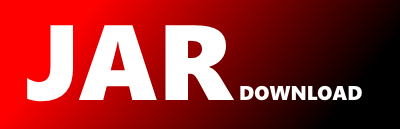
com.hashicorp.cdktf.GcsBackendConfig Maven / Gradle / Ivy
Show all versions of cdktf Show documentation
package com.hashicorp.cdktf;
/**
* (experimental) Stores the state as an object in a configurable prefix in a pre-existing bucket on Google Cloud Storage (GCS).
*
* The bucket must exist prior to configuring the backend.
*
* This backend supports state locking.
*
* Warning! It is highly recommended that you enable Object Versioning on the GCS bucket
* to allow for state recovery in the case of accidental deletions and human error.
*
* Read more about this backend in the Terraform docs:
* https://developer.hashicorp.com/terraform/language/settings/backends/gcs
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.102.0 (build e354887)", date = "2024-11-11T10:07:41.972Z")
@software.amazon.jsii.Jsii(module = com.hashicorp.cdktf.$Module.class, fqn = "cdktf.GcsBackendConfig")
@software.amazon.jsii.Jsii.Proxy(GcsBackendConfig.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public interface GcsBackendConfig extends software.amazon.jsii.JsiiSerializable {
/**
* (experimental) (Required) The name of the GCS bucket.
*
* This name must be globally unique.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@org.jetbrains.annotations.NotNull java.lang.String getBucket();
/**
* (experimental) (Optional) A temporary [OAuth 2.0 access token] obtained from the Google Authorization server, i.e. the Authorization: Bearer token used to authenticate HTTP requests to GCP APIs. This is an alternative to credentials. If both are specified, access_token will be used over the credentials field.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getAccessToken() {
return null;
}
/**
* (experimental) (Optional) Local path to Google Cloud Platform account credentials in JSON format.
*
* If unset, Google Application Default Credentials are used.
* The provided credentials must have Storage Object Admin role on the bucket.
*
* Warning: if using the Google Cloud Platform provider as well,
* it will also pick up the GOOGLE_CREDENTIALS environment variable.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getCredentials() {
return null;
}
/**
* (experimental) (Optional) A 32 byte base64 encoded 'customer supplied encryption key' used to encrypt all state.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getEncryptionKey() {
return null;
}
/**
* (experimental) (Optional) The service account to impersonate for accessing the State Bucket.
*
* You must have roles/iam.serviceAccountTokenCreator role on that account for the impersonation to succeed.
* If you are using a delegation chain, you can specify that using the impersonate_service_account_delegates field.
* Alternatively, this can be specified using the GOOGLE_IMPERSONATE_SERVICE_ACCOUNT environment variable.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getImpersonateServiceAccount() {
return null;
}
/**
* (experimental) (Optional) The delegation chain for an impersonating a service account.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.util.List getImpersonateServiceAccountDelegates() {
return null;
}
/**
* (experimental) (Optional) A Cloud KMS key ('customer-managed encryption key') used when reading and writing state files in the bucket.
*
* Format should be projects/{{project}}/locations/{{location}}/keyRings/{{keyRing}}/cryptoKeys/{{name}}.
* For more information, including IAM requirements, see {@link https://cloud.google.com/storage/docs/encryption/customer-managed-keys Customer-managed Encryption Keys}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getKmsEncryptionKey() {
return null;
}
/**
* (experimental) (Optional) GCS prefix inside the bucket.
*
* Named states for workspaces are stored in an object called /.tfstate.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getPrefix() {
return null;
}
/**
* (experimental) (Optional) A URL containing three parts: the protocol, the DNS name pointing to a Private Service Connect endpoint, and the path for the Cloud Storage API (/storage/v1/b).
*
* {@link https://developer.hashicorp.com/terraform/language/settings/backends/gcs#storage_custom_endpoint See here for more details}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getStoreageCustomEndpoint() {
return null;
}
/**
* @return a {@link Builder} of {@link GcsBackendConfig}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link GcsBackendConfig}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String bucket;
java.lang.String accessToken;
java.lang.String credentials;
java.lang.String encryptionKey;
java.lang.String impersonateServiceAccount;
java.util.List impersonateServiceAccountDelegates;
java.lang.String kmsEncryptionKey;
java.lang.String prefix;
java.lang.String storeageCustomEndpoint;
/**
* Sets the value of {@link GcsBackendConfig#getBucket}
* @param bucket (Required) The name of the GCS bucket. This parameter is required.
* This name must be globally unique.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder bucket(java.lang.String bucket) {
this.bucket = bucket;
return this;
}
/**
* Sets the value of {@link GcsBackendConfig#getAccessToken}
* @param accessToken (Optional) A temporary [OAuth 2.0 access token] obtained from the Google Authorization server, i.e. the Authorization: Bearer token used to authenticate HTTP requests to GCP APIs. This is an alternative to credentials. If both are specified, access_token will be used over the credentials field.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder accessToken(java.lang.String accessToken) {
this.accessToken = accessToken;
return this;
}
/**
* Sets the value of {@link GcsBackendConfig#getCredentials}
* @param credentials (Optional) Local path to Google Cloud Platform account credentials in JSON format.
* If unset, Google Application Default Credentials are used.
* The provided credentials must have Storage Object Admin role on the bucket.
*
* Warning: if using the Google Cloud Platform provider as well,
* it will also pick up the GOOGLE_CREDENTIALS environment variable.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder credentials(java.lang.String credentials) {
this.credentials = credentials;
return this;
}
/**
* Sets the value of {@link GcsBackendConfig#getEncryptionKey}
* @param encryptionKey (Optional) A 32 byte base64 encoded 'customer supplied encryption key' used to encrypt all state.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder encryptionKey(java.lang.String encryptionKey) {
this.encryptionKey = encryptionKey;
return this;
}
/**
* Sets the value of {@link GcsBackendConfig#getImpersonateServiceAccount}
* @param impersonateServiceAccount (Optional) The service account to impersonate for accessing the State Bucket.
* You must have roles/iam.serviceAccountTokenCreator role on that account for the impersonation to succeed.
* If you are using a delegation chain, you can specify that using the impersonate_service_account_delegates field.
* Alternatively, this can be specified using the GOOGLE_IMPERSONATE_SERVICE_ACCOUNT environment variable.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder impersonateServiceAccount(java.lang.String impersonateServiceAccount) {
this.impersonateServiceAccount = impersonateServiceAccount;
return this;
}
/**
* Sets the value of {@link GcsBackendConfig#getImpersonateServiceAccountDelegates}
* @param impersonateServiceAccountDelegates (Optional) The delegation chain for an impersonating a service account.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder impersonateServiceAccountDelegates(java.util.List impersonateServiceAccountDelegates) {
this.impersonateServiceAccountDelegates = impersonateServiceAccountDelegates;
return this;
}
/**
* Sets the value of {@link GcsBackendConfig#getKmsEncryptionKey}
* @param kmsEncryptionKey (Optional) A Cloud KMS key ('customer-managed encryption key') used when reading and writing state files in the bucket.
* Format should be projects/{{project}}/locations/{{location}}/keyRings/{{keyRing}}/cryptoKeys/{{name}}.
* For more information, including IAM requirements, see {@link https://cloud.google.com/storage/docs/encryption/customer-managed-keys Customer-managed Encryption Keys}.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder kmsEncryptionKey(java.lang.String kmsEncryptionKey) {
this.kmsEncryptionKey = kmsEncryptionKey;
return this;
}
/**
* Sets the value of {@link GcsBackendConfig#getPrefix}
* @param prefix (Optional) GCS prefix inside the bucket.
* Named states for workspaces are stored in an object called /.tfstate.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder prefix(java.lang.String prefix) {
this.prefix = prefix;
return this;
}
/**
* Sets the value of {@link GcsBackendConfig#getStoreageCustomEndpoint}
* @param storeageCustomEndpoint (Optional) A URL containing three parts: the protocol, the DNS name pointing to a Private Service Connect endpoint, and the path for the Cloud Storage API (/storage/v1/b).
* {@link https://developer.hashicorp.com/terraform/language/settings/backends/gcs#storage_custom_endpoint See here for more details}
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder storeageCustomEndpoint(java.lang.String storeageCustomEndpoint) {
this.storeageCustomEndpoint = storeageCustomEndpoint;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link GcsBackendConfig}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public GcsBackendConfig build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link GcsBackendConfig}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements GcsBackendConfig {
private final java.lang.String bucket;
private final java.lang.String accessToken;
private final java.lang.String credentials;
private final java.lang.String encryptionKey;
private final java.lang.String impersonateServiceAccount;
private final java.util.List impersonateServiceAccountDelegates;
private final java.lang.String kmsEncryptionKey;
private final java.lang.String prefix;
private final java.lang.String storeageCustomEndpoint;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.bucket = software.amazon.jsii.Kernel.get(this, "bucket", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.accessToken = software.amazon.jsii.Kernel.get(this, "accessToken", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.credentials = software.amazon.jsii.Kernel.get(this, "credentials", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.encryptionKey = software.amazon.jsii.Kernel.get(this, "encryptionKey", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.impersonateServiceAccount = software.amazon.jsii.Kernel.get(this, "impersonateServiceAccount", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.impersonateServiceAccountDelegates = software.amazon.jsii.Kernel.get(this, "impersonateServiceAccountDelegates", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.kmsEncryptionKey = software.amazon.jsii.Kernel.get(this, "kmsEncryptionKey", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.prefix = software.amazon.jsii.Kernel.get(this, "prefix", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.storeageCustomEndpoint = software.amazon.jsii.Kernel.get(this, "storeageCustomEndpoint", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.bucket = java.util.Objects.requireNonNull(builder.bucket, "bucket is required");
this.accessToken = builder.accessToken;
this.credentials = builder.credentials;
this.encryptionKey = builder.encryptionKey;
this.impersonateServiceAccount = builder.impersonateServiceAccount;
this.impersonateServiceAccountDelegates = builder.impersonateServiceAccountDelegates;
this.kmsEncryptionKey = builder.kmsEncryptionKey;
this.prefix = builder.prefix;
this.storeageCustomEndpoint = builder.storeageCustomEndpoint;
}
@Override
public final java.lang.String getBucket() {
return this.bucket;
}
@Override
public final java.lang.String getAccessToken() {
return this.accessToken;
}
@Override
public final java.lang.String getCredentials() {
return this.credentials;
}
@Override
public final java.lang.String getEncryptionKey() {
return this.encryptionKey;
}
@Override
public final java.lang.String getImpersonateServiceAccount() {
return this.impersonateServiceAccount;
}
@Override
public final java.util.List getImpersonateServiceAccountDelegates() {
return this.impersonateServiceAccountDelegates;
}
@Override
public final java.lang.String getKmsEncryptionKey() {
return this.kmsEncryptionKey;
}
@Override
public final java.lang.String getPrefix() {
return this.prefix;
}
@Override
public final java.lang.String getStoreageCustomEndpoint() {
return this.storeageCustomEndpoint;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("bucket", om.valueToTree(this.getBucket()));
if (this.getAccessToken() != null) {
data.set("accessToken", om.valueToTree(this.getAccessToken()));
}
if (this.getCredentials() != null) {
data.set("credentials", om.valueToTree(this.getCredentials()));
}
if (this.getEncryptionKey() != null) {
data.set("encryptionKey", om.valueToTree(this.getEncryptionKey()));
}
if (this.getImpersonateServiceAccount() != null) {
data.set("impersonateServiceAccount", om.valueToTree(this.getImpersonateServiceAccount()));
}
if (this.getImpersonateServiceAccountDelegates() != null) {
data.set("impersonateServiceAccountDelegates", om.valueToTree(this.getImpersonateServiceAccountDelegates()));
}
if (this.getKmsEncryptionKey() != null) {
data.set("kmsEncryptionKey", om.valueToTree(this.getKmsEncryptionKey()));
}
if (this.getPrefix() != null) {
data.set("prefix", om.valueToTree(this.getPrefix()));
}
if (this.getStoreageCustomEndpoint() != null) {
data.set("storeageCustomEndpoint", om.valueToTree(this.getStoreageCustomEndpoint()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("cdktf.GcsBackendConfig"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
GcsBackendConfig.Jsii$Proxy that = (GcsBackendConfig.Jsii$Proxy) o;
if (!bucket.equals(that.bucket)) return false;
if (this.accessToken != null ? !this.accessToken.equals(that.accessToken) : that.accessToken != null) return false;
if (this.credentials != null ? !this.credentials.equals(that.credentials) : that.credentials != null) return false;
if (this.encryptionKey != null ? !this.encryptionKey.equals(that.encryptionKey) : that.encryptionKey != null) return false;
if (this.impersonateServiceAccount != null ? !this.impersonateServiceAccount.equals(that.impersonateServiceAccount) : that.impersonateServiceAccount != null) return false;
if (this.impersonateServiceAccountDelegates != null ? !this.impersonateServiceAccountDelegates.equals(that.impersonateServiceAccountDelegates) : that.impersonateServiceAccountDelegates != null) return false;
if (this.kmsEncryptionKey != null ? !this.kmsEncryptionKey.equals(that.kmsEncryptionKey) : that.kmsEncryptionKey != null) return false;
if (this.prefix != null ? !this.prefix.equals(that.prefix) : that.prefix != null) return false;
return this.storeageCustomEndpoint != null ? this.storeageCustomEndpoint.equals(that.storeageCustomEndpoint) : that.storeageCustomEndpoint == null;
}
@Override
public final int hashCode() {
int result = this.bucket.hashCode();
result = 31 * result + (this.accessToken != null ? this.accessToken.hashCode() : 0);
result = 31 * result + (this.credentials != null ? this.credentials.hashCode() : 0);
result = 31 * result + (this.encryptionKey != null ? this.encryptionKey.hashCode() : 0);
result = 31 * result + (this.impersonateServiceAccount != null ? this.impersonateServiceAccount.hashCode() : 0);
result = 31 * result + (this.impersonateServiceAccountDelegates != null ? this.impersonateServiceAccountDelegates.hashCode() : 0);
result = 31 * result + (this.kmsEncryptionKey != null ? this.kmsEncryptionKey.hashCode() : 0);
result = 31 * result + (this.prefix != null ? this.prefix.hashCode() : 0);
result = 31 * result + (this.storeageCustomEndpoint != null ? this.storeageCustomEndpoint.hashCode() : 0);
return result;
}
}
}