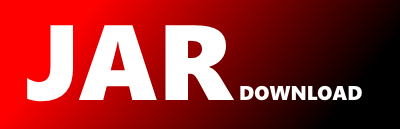
com.hashicorp.cdktf.AzurermBackendConfig Maven / Gradle / Ivy
package com.hashicorp.cdktf;
/**
* (experimental) Stores the state as a Blob with the given Key within the Blob Container within the Blob Storage Account.
*
* This backend supports state locking and consistency checking
* with Azure Blob Storage native capabilities.
*
* Note: By default the Azure Backend uses ADAL for authentication which is deprecated
* in favour of MSAL - MSAL can be used by setting use_microsoft_graph to true.
* The default for this will change in Terraform 1.2,
* so that MSAL authentication is used by default.
*
* Read more about this backend in the Terraform docs:
* https://developer.hashicorp.com/terraform/language/settings/backends/azurerm
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.98.0 (build 00b106d)", date = "2024-05-27T11:46:32.006Z")
@software.amazon.jsii.Jsii(module = com.hashicorp.cdktf.$Module.class, fqn = "cdktf.AzurermBackendConfig")
@software.amazon.jsii.Jsii.Proxy(AzurermBackendConfig.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public interface AzurermBackendConfig extends software.amazon.jsii.JsiiSerializable {
/**
* (experimental) (Required) The Name of the Storage Container within the Storage Account.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@org.jetbrains.annotations.NotNull java.lang.String getContainerName();
/**
* (experimental) (Required) The name of the Blob used to retrieve/store Terraform's State file inside the Storage Container.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@org.jetbrains.annotations.NotNull java.lang.String getKey();
/**
* (experimental) (Required) The Name of the Storage Account.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@org.jetbrains.annotations.NotNull java.lang.String getStorageAccountName();
/**
* (experimental) access_key - (Optional) The Access Key used to access the Blob Storage Account.
*
* This can also be sourced from the ARM_ACCESS_KEY environment variable.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getAccessKey() {
return null;
}
/**
* (experimental) (Optional) The password associated with the Client Certificate specified in client_certificate_path.
*
* This can also be sourced from the
* ARM_CLIENT_CERTIFICATE_PASSWORD environment variable.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getClientCertificatePassword() {
return null;
}
/**
* (experimental) (Optional) The path to the PFX file used as the Client Certificate when authenticating as a Service Principal.
*
* This can also be sourced from the
* ARM_CLIENT_CERTIFICATE_PATH environment variable.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getClientCertificatePath() {
return null;
}
/**
* (experimental) (Optional) The Client ID of the Service Principal.
*
* This can also be sourced from the ARM_CLIENT_ID environment variable.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getClientId() {
return null;
}
/**
* (experimental) (Optional) The Client Secret of the Service Principal.
*
* This can also be sourced from the ARM_CLIENT_SECRET environment variable.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getClientSecret() {
return null;
}
/**
* (experimental) (Optional) The Custom Endpoint for Azure Resource Manager. This can also be sourced from the ARM_ENDPOINT environment variable.
*
* NOTE: An endpoint should only be configured when using Azure Stack.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getEndpoint() {
return null;
}
/**
* (experimental) (Optional) The Azure Environment which should be used.
*
* This can also be sourced from the ARM_ENVIRONMENT environment variable.
* Possible values are public, china, german, stack and usgovernment. Defaults to public.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getEnvironment() {
return null;
}
/**
* (experimental) (Optional) The Hostname of the Azure Metadata Service (for example management.azure.com), used to obtain the Cloud Environment when using a Custom Azure Environment. This can also be sourced from the ARM_METADATA_HOSTNAME Environment Variable.).
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getMetadataHost() {
return null;
}
/**
* (experimental) (Optional) The path to a custom Managed Service Identity endpoint which is automatically determined if not specified.
*
* This can also be sourced from the ARM_MSI_ENDPOINT environment variable.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getMsiEndpoint() {
return null;
}
/**
* (experimental) (Optional) The bearer token for the request to the OIDC provider.
*
* This can
* also be sourced from the ARM_OIDC_REQUEST_TOKEN or
* ACTIONS_ID_TOKEN_REQUEST_TOKEN environment variables.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getOidcRequestToken() {
return null;
}
/**
* (experimental) (Optional) The URL for the OIDC provider from which to request an ID token.
*
* This can also be sourced from the ARM_OIDC_REQUEST_URL or
* ACTIONS_ID_TOKEN_REQUEST_URL environment variables.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getOidcRequestUrl() {
return null;
}
/**
* (experimental) (Optional) The ID token when authenticating using OpenID Connect (OIDC).
*
* This can also be sourced from the ARM_OIDC_TOKEN environment variable.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getOidcToken() {
return null;
}
/**
* (experimental) (Optional) The path to a file containing an ID token when authenticating using OpenID Connect (OIDC).
*
* This can also be sourced from the ARM_OIDC_TOKEN_FILE_PATH environment variable.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getOidcTokenFilePath() {
return null;
}
/**
* (experimental) (Required) The Name of the Resource Group in which the Storage Account exists.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getResourceGroupName() {
return null;
}
/**
* (experimental) (Optional) The SAS Token used to access the Blob Storage Account.
*
* This can also be sourced from the ARM_SAS_TOKEN environment variable.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getSasToken() {
return null;
}
/**
* (experimental) (Optional) Should the Blob used to store the Terraform Statefile be snapshotted before use?
*
* Defaults to false. This value can also be sourced
* from the ARM_SNAPSHOT environment variable.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getSnapshot() {
return null;
}
/**
* (experimental) (Optional) The Subscription ID in which the Storage Account exists.
*
* This can also be sourced from the ARM_SUBSCRIPTION_ID environment variable.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getSubscriptionId() {
return null;
}
/**
* (experimental) (Optional) The Tenant ID in which the Subscription exists.
*
* This can also be sourced from the ARM_TENANT_ID environment variable.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getTenantId() {
return null;
}
/**
* (experimental) (Optional) Should AzureAD Authentication be used to access the Blob Storage Account.
*
* This can also be sourced from the ARM_USE_AZUREAD environment
* variable.
*
* Note: When using AzureAD for Authentication to Storage you also need to
* ensure the Storage Blob Data Owner role is assigned.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getUseAzureadAuth() {
return null;
}
/**
* (experimental) (Optional) Should MSAL be used for authentication instead of ADAL, and should Microsoft Graph be used instead of Azure Active Directory Graph?
*
* Defaults to true.
*
* Note: In Terraform 1.2 the Azure Backend uses MSAL (and Microsoft Graph)
* rather than ADAL (and Azure Active Directory Graph) for authentication by
* default - you can disable this by setting use_microsoft_graph to false.
* This setting will be removed in Terraform 1.3, due to Microsoft's
* deprecation of ADAL.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getUseMicrosoftGraph() {
return null;
}
/**
* (experimental) (Optional) Should Managed Service Identity authentication be used?
*
* This can also be sourced from the ARM_USE_MSI environment variable.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getUseMsi() {
return null;
}
/**
* (experimental) (Optional) Should OIDC authentication be used? This can also be sourced from the ARM_USE_OIDC environment variable.
*
* Note: When using OIDC for authentication, use_microsoft_graph
* must be set to true (which is the default).
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getUseOidc() {
return null;
}
/**
* @return a {@link Builder} of {@link AzurermBackendConfig}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link AzurermBackendConfig}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String containerName;
java.lang.String key;
java.lang.String storageAccountName;
java.lang.String accessKey;
java.lang.String clientCertificatePassword;
java.lang.String clientCertificatePath;
java.lang.String clientId;
java.lang.String clientSecret;
java.lang.String endpoint;
java.lang.String environment;
java.lang.String metadataHost;
java.lang.String msiEndpoint;
java.lang.String oidcRequestToken;
java.lang.String oidcRequestUrl;
java.lang.String oidcToken;
java.lang.String oidcTokenFilePath;
java.lang.String resourceGroupName;
java.lang.String sasToken;
java.lang.Boolean snapshot;
java.lang.String subscriptionId;
java.lang.String tenantId;
java.lang.Boolean useAzureadAuth;
java.lang.Boolean useMicrosoftGraph;
java.lang.Boolean useMsi;
java.lang.Boolean useOidc;
/**
* Sets the value of {@link AzurermBackendConfig#getContainerName}
* @param containerName (Required) The Name of the Storage Container within the Storage Account. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder containerName(java.lang.String containerName) {
this.containerName = containerName;
return this;
}
/**
* Sets the value of {@link AzurermBackendConfig#getKey}
* @param key (Required) The name of the Blob used to retrieve/store Terraform's State file inside the Storage Container. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder key(java.lang.String key) {
this.key = key;
return this;
}
/**
* Sets the value of {@link AzurermBackendConfig#getStorageAccountName}
* @param storageAccountName (Required) The Name of the Storage Account. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder storageAccountName(java.lang.String storageAccountName) {
this.storageAccountName = storageAccountName;
return this;
}
/**
* Sets the value of {@link AzurermBackendConfig#getAccessKey}
* @param accessKey access_key - (Optional) The Access Key used to access the Blob Storage Account.
* This can also be sourced from the ARM_ACCESS_KEY environment variable.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder accessKey(java.lang.String accessKey) {
this.accessKey = accessKey;
return this;
}
/**
* Sets the value of {@link AzurermBackendConfig#getClientCertificatePassword}
* @param clientCertificatePassword (Optional) The password associated with the Client Certificate specified in client_certificate_path.
* This can also be sourced from the
* ARM_CLIENT_CERTIFICATE_PASSWORD environment variable.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder clientCertificatePassword(java.lang.String clientCertificatePassword) {
this.clientCertificatePassword = clientCertificatePassword;
return this;
}
/**
* Sets the value of {@link AzurermBackendConfig#getClientCertificatePath}
* @param clientCertificatePath (Optional) The path to the PFX file used as the Client Certificate when authenticating as a Service Principal.
* This can also be sourced from the
* ARM_CLIENT_CERTIFICATE_PATH environment variable.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder clientCertificatePath(java.lang.String clientCertificatePath) {
this.clientCertificatePath = clientCertificatePath;
return this;
}
/**
* Sets the value of {@link AzurermBackendConfig#getClientId}
* @param clientId (Optional) The Client ID of the Service Principal.
* This can also be sourced from the ARM_CLIENT_ID environment variable.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder clientId(java.lang.String clientId) {
this.clientId = clientId;
return this;
}
/**
* Sets the value of {@link AzurermBackendConfig#getClientSecret}
* @param clientSecret (Optional) The Client Secret of the Service Principal.
* This can also be sourced from the ARM_CLIENT_SECRET environment variable.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder clientSecret(java.lang.String clientSecret) {
this.clientSecret = clientSecret;
return this;
}
/**
* Sets the value of {@link AzurermBackendConfig#getEndpoint}
* @param endpoint (Optional) The Custom Endpoint for Azure Resource Manager. This can also be sourced from the ARM_ENDPOINT environment variable.
* NOTE: An endpoint should only be configured when using Azure Stack.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder endpoint(java.lang.String endpoint) {
this.endpoint = endpoint;
return this;
}
/**
* Sets the value of {@link AzurermBackendConfig#getEnvironment}
* @param environment (Optional) The Azure Environment which should be used.
* This can also be sourced from the ARM_ENVIRONMENT environment variable.
* Possible values are public, china, german, stack and usgovernment. Defaults to public.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder environment(java.lang.String environment) {
this.environment = environment;
return this;
}
/**
* Sets the value of {@link AzurermBackendConfig#getMetadataHost}
* @param metadataHost (Optional) The Hostname of the Azure Metadata Service (for example management.azure.com), used to obtain the Cloud Environment when using a Custom Azure Environment. This can also be sourced from the ARM_METADATA_HOSTNAME Environment Variable.).
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder metadataHost(java.lang.String metadataHost) {
this.metadataHost = metadataHost;
return this;
}
/**
* Sets the value of {@link AzurermBackendConfig#getMsiEndpoint}
* @param msiEndpoint (Optional) The path to a custom Managed Service Identity endpoint which is automatically determined if not specified.
* This can also be sourced from the ARM_MSI_ENDPOINT environment variable.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder msiEndpoint(java.lang.String msiEndpoint) {
this.msiEndpoint = msiEndpoint;
return this;
}
/**
* Sets the value of {@link AzurermBackendConfig#getOidcRequestToken}
* @param oidcRequestToken (Optional) The bearer token for the request to the OIDC provider.
* This can
* also be sourced from the ARM_OIDC_REQUEST_TOKEN or
* ACTIONS_ID_TOKEN_REQUEST_TOKEN environment variables.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder oidcRequestToken(java.lang.String oidcRequestToken) {
this.oidcRequestToken = oidcRequestToken;
return this;
}
/**
* Sets the value of {@link AzurermBackendConfig#getOidcRequestUrl}
* @param oidcRequestUrl (Optional) The URL for the OIDC provider from which to request an ID token.
* This can also be sourced from the ARM_OIDC_REQUEST_URL or
* ACTIONS_ID_TOKEN_REQUEST_URL environment variables.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder oidcRequestUrl(java.lang.String oidcRequestUrl) {
this.oidcRequestUrl = oidcRequestUrl;
return this;
}
/**
* Sets the value of {@link AzurermBackendConfig#getOidcToken}
* @param oidcToken (Optional) The ID token when authenticating using OpenID Connect (OIDC).
* This can also be sourced from the ARM_OIDC_TOKEN environment variable.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder oidcToken(java.lang.String oidcToken) {
this.oidcToken = oidcToken;
return this;
}
/**
* Sets the value of {@link AzurermBackendConfig#getOidcTokenFilePath}
* @param oidcTokenFilePath (Optional) The path to a file containing an ID token when authenticating using OpenID Connect (OIDC).
* This can also be sourced from the ARM_OIDC_TOKEN_FILE_PATH environment variable.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder oidcTokenFilePath(java.lang.String oidcTokenFilePath) {
this.oidcTokenFilePath = oidcTokenFilePath;
return this;
}
/**
* Sets the value of {@link AzurermBackendConfig#getResourceGroupName}
* @param resourceGroupName (Required) The Name of the Resource Group in which the Storage Account exists.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder resourceGroupName(java.lang.String resourceGroupName) {
this.resourceGroupName = resourceGroupName;
return this;
}
/**
* Sets the value of {@link AzurermBackendConfig#getSasToken}
* @param sasToken (Optional) The SAS Token used to access the Blob Storage Account.
* This can also be sourced from the ARM_SAS_TOKEN environment variable.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder sasToken(java.lang.String sasToken) {
this.sasToken = sasToken;
return this;
}
/**
* Sets the value of {@link AzurermBackendConfig#getSnapshot}
* @param snapshot (Optional) Should the Blob used to store the Terraform Statefile be snapshotted before use?.
* Defaults to false. This value can also be sourced
* from the ARM_SNAPSHOT environment variable.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder snapshot(java.lang.Boolean snapshot) {
this.snapshot = snapshot;
return this;
}
/**
* Sets the value of {@link AzurermBackendConfig#getSubscriptionId}
* @param subscriptionId (Optional) The Subscription ID in which the Storage Account exists.
* This can also be sourced from the ARM_SUBSCRIPTION_ID environment variable.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder subscriptionId(java.lang.String subscriptionId) {
this.subscriptionId = subscriptionId;
return this;
}
/**
* Sets the value of {@link AzurermBackendConfig#getTenantId}
* @param tenantId (Optional) The Tenant ID in which the Subscription exists.
* This can also be sourced from the ARM_TENANT_ID environment variable.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder tenantId(java.lang.String tenantId) {
this.tenantId = tenantId;
return this;
}
/**
* Sets the value of {@link AzurermBackendConfig#getUseAzureadAuth}
* @param useAzureadAuth (Optional) Should AzureAD Authentication be used to access the Blob Storage Account.
* This can also be sourced from the ARM_USE_AZUREAD environment
* variable.
*
* Note: When using AzureAD for Authentication to Storage you also need to
* ensure the Storage Blob Data Owner role is assigned.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder useAzureadAuth(java.lang.Boolean useAzureadAuth) {
this.useAzureadAuth = useAzureadAuth;
return this;
}
/**
* Sets the value of {@link AzurermBackendConfig#getUseMicrosoftGraph}
* @param useMicrosoftGraph (Optional) Should MSAL be used for authentication instead of ADAL, and should Microsoft Graph be used instead of Azure Active Directory Graph?.
* Defaults to true.
*
* Note: In Terraform 1.2 the Azure Backend uses MSAL (and Microsoft Graph)
* rather than ADAL (and Azure Active Directory Graph) for authentication by
* default - you can disable this by setting use_microsoft_graph to false.
* This setting will be removed in Terraform 1.3, due to Microsoft's
* deprecation of ADAL.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder useMicrosoftGraph(java.lang.Boolean useMicrosoftGraph) {
this.useMicrosoftGraph = useMicrosoftGraph;
return this;
}
/**
* Sets the value of {@link AzurermBackendConfig#getUseMsi}
* @param useMsi (Optional) Should Managed Service Identity authentication be used?.
* This can also be sourced from the ARM_USE_MSI environment variable.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder useMsi(java.lang.Boolean useMsi) {
this.useMsi = useMsi;
return this;
}
/**
* Sets the value of {@link AzurermBackendConfig#getUseOidc}
* @param useOidc (Optional) Should OIDC authentication be used? This can also be sourced from the ARM_USE_OIDC environment variable.
* Note: When using OIDC for authentication, use_microsoft_graph
* must be set to true (which is the default).
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder useOidc(java.lang.Boolean useOidc) {
this.useOidc = useOidc;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link AzurermBackendConfig}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public AzurermBackendConfig build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link AzurermBackendConfig}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements AzurermBackendConfig {
private final java.lang.String containerName;
private final java.lang.String key;
private final java.lang.String storageAccountName;
private final java.lang.String accessKey;
private final java.lang.String clientCertificatePassword;
private final java.lang.String clientCertificatePath;
private final java.lang.String clientId;
private final java.lang.String clientSecret;
private final java.lang.String endpoint;
private final java.lang.String environment;
private final java.lang.String metadataHost;
private final java.lang.String msiEndpoint;
private final java.lang.String oidcRequestToken;
private final java.lang.String oidcRequestUrl;
private final java.lang.String oidcToken;
private final java.lang.String oidcTokenFilePath;
private final java.lang.String resourceGroupName;
private final java.lang.String sasToken;
private final java.lang.Boolean snapshot;
private final java.lang.String subscriptionId;
private final java.lang.String tenantId;
private final java.lang.Boolean useAzureadAuth;
private final java.lang.Boolean useMicrosoftGraph;
private final java.lang.Boolean useMsi;
private final java.lang.Boolean useOidc;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.containerName = software.amazon.jsii.Kernel.get(this, "containerName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.key = software.amazon.jsii.Kernel.get(this, "key", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.storageAccountName = software.amazon.jsii.Kernel.get(this, "storageAccountName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.accessKey = software.amazon.jsii.Kernel.get(this, "accessKey", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.clientCertificatePassword = software.amazon.jsii.Kernel.get(this, "clientCertificatePassword", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.clientCertificatePath = software.amazon.jsii.Kernel.get(this, "clientCertificatePath", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.clientId = software.amazon.jsii.Kernel.get(this, "clientId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.clientSecret = software.amazon.jsii.Kernel.get(this, "clientSecret", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.endpoint = software.amazon.jsii.Kernel.get(this, "endpoint", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.environment = software.amazon.jsii.Kernel.get(this, "environment", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.metadataHost = software.amazon.jsii.Kernel.get(this, "metadataHost", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.msiEndpoint = software.amazon.jsii.Kernel.get(this, "msiEndpoint", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.oidcRequestToken = software.amazon.jsii.Kernel.get(this, "oidcRequestToken", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.oidcRequestUrl = software.amazon.jsii.Kernel.get(this, "oidcRequestUrl", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.oidcToken = software.amazon.jsii.Kernel.get(this, "oidcToken", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.oidcTokenFilePath = software.amazon.jsii.Kernel.get(this, "oidcTokenFilePath", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.resourceGroupName = software.amazon.jsii.Kernel.get(this, "resourceGroupName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.sasToken = software.amazon.jsii.Kernel.get(this, "sasToken", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.snapshot = software.amazon.jsii.Kernel.get(this, "snapshot", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.subscriptionId = software.amazon.jsii.Kernel.get(this, "subscriptionId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.tenantId = software.amazon.jsii.Kernel.get(this, "tenantId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.useAzureadAuth = software.amazon.jsii.Kernel.get(this, "useAzureadAuth", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.useMicrosoftGraph = software.amazon.jsii.Kernel.get(this, "useMicrosoftGraph", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.useMsi = software.amazon.jsii.Kernel.get(this, "useMsi", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.useOidc = software.amazon.jsii.Kernel.get(this, "useOidc", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.containerName = java.util.Objects.requireNonNull(builder.containerName, "containerName is required");
this.key = java.util.Objects.requireNonNull(builder.key, "key is required");
this.storageAccountName = java.util.Objects.requireNonNull(builder.storageAccountName, "storageAccountName is required");
this.accessKey = builder.accessKey;
this.clientCertificatePassword = builder.clientCertificatePassword;
this.clientCertificatePath = builder.clientCertificatePath;
this.clientId = builder.clientId;
this.clientSecret = builder.clientSecret;
this.endpoint = builder.endpoint;
this.environment = builder.environment;
this.metadataHost = builder.metadataHost;
this.msiEndpoint = builder.msiEndpoint;
this.oidcRequestToken = builder.oidcRequestToken;
this.oidcRequestUrl = builder.oidcRequestUrl;
this.oidcToken = builder.oidcToken;
this.oidcTokenFilePath = builder.oidcTokenFilePath;
this.resourceGroupName = builder.resourceGroupName;
this.sasToken = builder.sasToken;
this.snapshot = builder.snapshot;
this.subscriptionId = builder.subscriptionId;
this.tenantId = builder.tenantId;
this.useAzureadAuth = builder.useAzureadAuth;
this.useMicrosoftGraph = builder.useMicrosoftGraph;
this.useMsi = builder.useMsi;
this.useOidc = builder.useOidc;
}
@Override
public final java.lang.String getContainerName() {
return this.containerName;
}
@Override
public final java.lang.String getKey() {
return this.key;
}
@Override
public final java.lang.String getStorageAccountName() {
return this.storageAccountName;
}
@Override
public final java.lang.String getAccessKey() {
return this.accessKey;
}
@Override
public final java.lang.String getClientCertificatePassword() {
return this.clientCertificatePassword;
}
@Override
public final java.lang.String getClientCertificatePath() {
return this.clientCertificatePath;
}
@Override
public final java.lang.String getClientId() {
return this.clientId;
}
@Override
public final java.lang.String getClientSecret() {
return this.clientSecret;
}
@Override
public final java.lang.String getEndpoint() {
return this.endpoint;
}
@Override
public final java.lang.String getEnvironment() {
return this.environment;
}
@Override
public final java.lang.String getMetadataHost() {
return this.metadataHost;
}
@Override
public final java.lang.String getMsiEndpoint() {
return this.msiEndpoint;
}
@Override
public final java.lang.String getOidcRequestToken() {
return this.oidcRequestToken;
}
@Override
public final java.lang.String getOidcRequestUrl() {
return this.oidcRequestUrl;
}
@Override
public final java.lang.String getOidcToken() {
return this.oidcToken;
}
@Override
public final java.lang.String getOidcTokenFilePath() {
return this.oidcTokenFilePath;
}
@Override
public final java.lang.String getResourceGroupName() {
return this.resourceGroupName;
}
@Override
public final java.lang.String getSasToken() {
return this.sasToken;
}
@Override
public final java.lang.Boolean getSnapshot() {
return this.snapshot;
}
@Override
public final java.lang.String getSubscriptionId() {
return this.subscriptionId;
}
@Override
public final java.lang.String getTenantId() {
return this.tenantId;
}
@Override
public final java.lang.Boolean getUseAzureadAuth() {
return this.useAzureadAuth;
}
@Override
public final java.lang.Boolean getUseMicrosoftGraph() {
return this.useMicrosoftGraph;
}
@Override
public final java.lang.Boolean getUseMsi() {
return this.useMsi;
}
@Override
public final java.lang.Boolean getUseOidc() {
return this.useOidc;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("containerName", om.valueToTree(this.getContainerName()));
data.set("key", om.valueToTree(this.getKey()));
data.set("storageAccountName", om.valueToTree(this.getStorageAccountName()));
if (this.getAccessKey() != null) {
data.set("accessKey", om.valueToTree(this.getAccessKey()));
}
if (this.getClientCertificatePassword() != null) {
data.set("clientCertificatePassword", om.valueToTree(this.getClientCertificatePassword()));
}
if (this.getClientCertificatePath() != null) {
data.set("clientCertificatePath", om.valueToTree(this.getClientCertificatePath()));
}
if (this.getClientId() != null) {
data.set("clientId", om.valueToTree(this.getClientId()));
}
if (this.getClientSecret() != null) {
data.set("clientSecret", om.valueToTree(this.getClientSecret()));
}
if (this.getEndpoint() != null) {
data.set("endpoint", om.valueToTree(this.getEndpoint()));
}
if (this.getEnvironment() != null) {
data.set("environment", om.valueToTree(this.getEnvironment()));
}
if (this.getMetadataHost() != null) {
data.set("metadataHost", om.valueToTree(this.getMetadataHost()));
}
if (this.getMsiEndpoint() != null) {
data.set("msiEndpoint", om.valueToTree(this.getMsiEndpoint()));
}
if (this.getOidcRequestToken() != null) {
data.set("oidcRequestToken", om.valueToTree(this.getOidcRequestToken()));
}
if (this.getOidcRequestUrl() != null) {
data.set("oidcRequestUrl", om.valueToTree(this.getOidcRequestUrl()));
}
if (this.getOidcToken() != null) {
data.set("oidcToken", om.valueToTree(this.getOidcToken()));
}
if (this.getOidcTokenFilePath() != null) {
data.set("oidcTokenFilePath", om.valueToTree(this.getOidcTokenFilePath()));
}
if (this.getResourceGroupName() != null) {
data.set("resourceGroupName", om.valueToTree(this.getResourceGroupName()));
}
if (this.getSasToken() != null) {
data.set("sasToken", om.valueToTree(this.getSasToken()));
}
if (this.getSnapshot() != null) {
data.set("snapshot", om.valueToTree(this.getSnapshot()));
}
if (this.getSubscriptionId() != null) {
data.set("subscriptionId", om.valueToTree(this.getSubscriptionId()));
}
if (this.getTenantId() != null) {
data.set("tenantId", om.valueToTree(this.getTenantId()));
}
if (this.getUseAzureadAuth() != null) {
data.set("useAzureadAuth", om.valueToTree(this.getUseAzureadAuth()));
}
if (this.getUseMicrosoftGraph() != null) {
data.set("useMicrosoftGraph", om.valueToTree(this.getUseMicrosoftGraph()));
}
if (this.getUseMsi() != null) {
data.set("useMsi", om.valueToTree(this.getUseMsi()));
}
if (this.getUseOidc() != null) {
data.set("useOidc", om.valueToTree(this.getUseOidc()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("cdktf.AzurermBackendConfig"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
AzurermBackendConfig.Jsii$Proxy that = (AzurermBackendConfig.Jsii$Proxy) o;
if (!containerName.equals(that.containerName)) return false;
if (!key.equals(that.key)) return false;
if (!storageAccountName.equals(that.storageAccountName)) return false;
if (this.accessKey != null ? !this.accessKey.equals(that.accessKey) : that.accessKey != null) return false;
if (this.clientCertificatePassword != null ? !this.clientCertificatePassword.equals(that.clientCertificatePassword) : that.clientCertificatePassword != null) return false;
if (this.clientCertificatePath != null ? !this.clientCertificatePath.equals(that.clientCertificatePath) : that.clientCertificatePath != null) return false;
if (this.clientId != null ? !this.clientId.equals(that.clientId) : that.clientId != null) return false;
if (this.clientSecret != null ? !this.clientSecret.equals(that.clientSecret) : that.clientSecret != null) return false;
if (this.endpoint != null ? !this.endpoint.equals(that.endpoint) : that.endpoint != null) return false;
if (this.environment != null ? !this.environment.equals(that.environment) : that.environment != null) return false;
if (this.metadataHost != null ? !this.metadataHost.equals(that.metadataHost) : that.metadataHost != null) return false;
if (this.msiEndpoint != null ? !this.msiEndpoint.equals(that.msiEndpoint) : that.msiEndpoint != null) return false;
if (this.oidcRequestToken != null ? !this.oidcRequestToken.equals(that.oidcRequestToken) : that.oidcRequestToken != null) return false;
if (this.oidcRequestUrl != null ? !this.oidcRequestUrl.equals(that.oidcRequestUrl) : that.oidcRequestUrl != null) return false;
if (this.oidcToken != null ? !this.oidcToken.equals(that.oidcToken) : that.oidcToken != null) return false;
if (this.oidcTokenFilePath != null ? !this.oidcTokenFilePath.equals(that.oidcTokenFilePath) : that.oidcTokenFilePath != null) return false;
if (this.resourceGroupName != null ? !this.resourceGroupName.equals(that.resourceGroupName) : that.resourceGroupName != null) return false;
if (this.sasToken != null ? !this.sasToken.equals(that.sasToken) : that.sasToken != null) return false;
if (this.snapshot != null ? !this.snapshot.equals(that.snapshot) : that.snapshot != null) return false;
if (this.subscriptionId != null ? !this.subscriptionId.equals(that.subscriptionId) : that.subscriptionId != null) return false;
if (this.tenantId != null ? !this.tenantId.equals(that.tenantId) : that.tenantId != null) return false;
if (this.useAzureadAuth != null ? !this.useAzureadAuth.equals(that.useAzureadAuth) : that.useAzureadAuth != null) return false;
if (this.useMicrosoftGraph != null ? !this.useMicrosoftGraph.equals(that.useMicrosoftGraph) : that.useMicrosoftGraph != null) return false;
if (this.useMsi != null ? !this.useMsi.equals(that.useMsi) : that.useMsi != null) return false;
return this.useOidc != null ? this.useOidc.equals(that.useOidc) : that.useOidc == null;
}
@Override
public final int hashCode() {
int result = this.containerName.hashCode();
result = 31 * result + (this.key.hashCode());
result = 31 * result + (this.storageAccountName.hashCode());
result = 31 * result + (this.accessKey != null ? this.accessKey.hashCode() : 0);
result = 31 * result + (this.clientCertificatePassword != null ? this.clientCertificatePassword.hashCode() : 0);
result = 31 * result + (this.clientCertificatePath != null ? this.clientCertificatePath.hashCode() : 0);
result = 31 * result + (this.clientId != null ? this.clientId.hashCode() : 0);
result = 31 * result + (this.clientSecret != null ? this.clientSecret.hashCode() : 0);
result = 31 * result + (this.endpoint != null ? this.endpoint.hashCode() : 0);
result = 31 * result + (this.environment != null ? this.environment.hashCode() : 0);
result = 31 * result + (this.metadataHost != null ? this.metadataHost.hashCode() : 0);
result = 31 * result + (this.msiEndpoint != null ? this.msiEndpoint.hashCode() : 0);
result = 31 * result + (this.oidcRequestToken != null ? this.oidcRequestToken.hashCode() : 0);
result = 31 * result + (this.oidcRequestUrl != null ? this.oidcRequestUrl.hashCode() : 0);
result = 31 * result + (this.oidcToken != null ? this.oidcToken.hashCode() : 0);
result = 31 * result + (this.oidcTokenFilePath != null ? this.oidcTokenFilePath.hashCode() : 0);
result = 31 * result + (this.resourceGroupName != null ? this.resourceGroupName.hashCode() : 0);
result = 31 * result + (this.sasToken != null ? this.sasToken.hashCode() : 0);
result = 31 * result + (this.snapshot != null ? this.snapshot.hashCode() : 0);
result = 31 * result + (this.subscriptionId != null ? this.subscriptionId.hashCode() : 0);
result = 31 * result + (this.tenantId != null ? this.tenantId.hashCode() : 0);
result = 31 * result + (this.useAzureadAuth != null ? this.useAzureadAuth.hashCode() : 0);
result = 31 * result + (this.useMicrosoftGraph != null ? this.useMicrosoftGraph.hashCode() : 0);
result = 31 * result + (this.useMsi != null ? this.useMsi.hashCode() : 0);
result = 31 * result + (this.useOidc != null ? this.useOidc.hashCode() : 0);
return result;
}
}
}