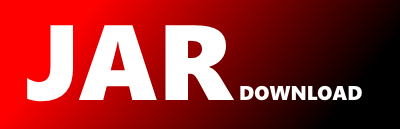
com.hashicorp.cdktf.OssBackendConfig Maven / Gradle / Ivy
package com.hashicorp.cdktf;
/**
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.98.0 (build 00b106d)", date = "2024-05-27T11:46:32.223Z")
@software.amazon.jsii.Jsii(module = com.hashicorp.cdktf.$Module.class, fqn = "cdktf.OssBackendConfig")
@software.amazon.jsii.Jsii.Proxy(OssBackendConfig.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public interface OssBackendConfig extends software.amazon.jsii.JsiiSerializable {
/**
* (experimental) (Required) The name of the OSS bucket.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@org.jetbrains.annotations.NotNull java.lang.String getBucket();
/**
* (experimental) (Optional) Alibaba Cloud access key.
*
* It supports environment variables ALICLOUD_ACCESS_KEY and ALICLOUD_ACCESS_KEY_ID.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getAccessKey() {
return null;
}
/**
* (experimental) (Optional) Object ACL to be applied to the state file.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getAcl() {
return null;
}
/**
* @deprecated Use flattened assume role options
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
default @org.jetbrains.annotations.Nullable com.hashicorp.cdktf.OssAssumeRole getAssumeRole() {
return null;
}
/**
* (experimental) (Optional, Available in 1.1.0+) A more restrictive policy to apply to the temporary credentials. This gives you a way to further restrict the permissions for the resulting temporary security credentials. You cannot use this policy to grant permissions that exceed those of the role that is being assumed.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getAssumeRolePolicy() {
return null;
}
/**
* (experimental) (Optional, Available in 1.1.0+) The ARN of the role to assume. If ARN is set to an empty string, it does not perform role switching. It supports the environment variable ALICLOUD_ASSUME_ROLE_ARN. Terraform executes configuration on account with provided credentials.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getAssumeRoleRoleArn() {
return null;
}
/**
* (experimental) (Optional, Available in 1.1.0+) The time after which the established session for assuming role expires. Valid value range: [900-3600] seconds. Default to 3600 (in this case Alibaba Cloud uses its own default value). It supports environment variable ALICLOUD_ASSUME_ROLE_SESSION_EXPIRATION.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.Number getAssumeRoleSessionExpiration() {
return null;
}
/**
* (experimental) (Optional, Available in 1.1.0+) The session name to use when assuming the role. If omitted, 'terraform' is passed to the AssumeRole call as session name. It supports environment variable ALICLOUD_ASSUME_ROLE_SESSION_NAME.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getAssumeRoleSessionName() {
return null;
}
/**
* (experimental) (Optional, Available in 0.12.14+) The RAM Role Name attached on a ECS instance for API operations. You can retrieve this from the 'Access Control' section of the Alibaba Cloud console.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getEcsRoleName() {
return null;
}
/**
* (experimental) (Optional) Whether to enable server side encryption of the state file.
*
* If it is true, OSS will use 'AES256' encryption algorithm to encrypt state file.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getEncrypt() {
return null;
}
/**
* (experimental) (Optional) A custom endpoint for the OSS API.
*
* It supports environment variables ALICLOUD_OSS_ENDPOINT and OSS_ENDPOINT.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getEndpoint() {
return null;
}
/**
* (experimental) (Optional) The name of the state file.
*
* Defaults to terraform.tfstate.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getKey() {
return null;
}
/**
* (experimental) (Optional) The path directory of the state file will be stored.
*
* Default to "env:".
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getPrefix() {
return null;
}
/**
* (experimental) (Optional, Available in 0.12.8+) This is the Alibaba Cloud profile name as set in the shared credentials file. It can also be sourced from the ALICLOUD_PROFILE environment variable.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getProfile() {
return null;
}
/**
* (experimental) (Optional) The region of the OSS bucket.
*
* It supports environment variables ALICLOUD_REGION and ALICLOUD_DEFAULT_REGION.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getRegion() {
return null;
}
/**
* (experimental) (Optional) Alibaba Cloud secret access key.
*
* It supports environment variables ALICLOUD_SECRET_KEY and ALICLOUD_ACCESS_KEY_SECRET.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getSecretKey() {
return null;
}
/**
* (experimental) (Optional) STS access token.
*
* It supports environment variable ALICLOUD_SECURITY_TOKEN.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getSecurityToken() {
return null;
}
/**
* (experimental) (Optional, Available in 0.12.8+) This is the path to the shared credentials file. It can also be sourced from the ALICLOUD_SHARED_CREDENTIALS_FILE environment variable. If this is not set and a profile is specified, ~/.aliyun/config.json will be used.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getSharedCredentialsFile() {
return null;
}
/**
* (experimental) (Optional, Available in 1.0.11+) Custom endpoint for the AliCloud Security Token Service (STS) API. It supports environment variable ALICLOUD_STS_ENDPOINT.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getStsEndpoint() {
return null;
}
/**
* (experimental) (Optional) A custom endpoint for the TableStore API.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getTablestoreEndpoint() {
return null;
}
/**
* (experimental) (Optional) A TableStore table for state locking and consistency.
*
* The table must have a primary key named LockID of type String.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getTablestoreTable() {
return null;
}
/**
* @return a {@link Builder} of {@link OssBackendConfig}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link OssBackendConfig}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String bucket;
java.lang.String accessKey;
java.lang.String acl;
com.hashicorp.cdktf.OssAssumeRole assumeRole;
java.lang.String assumeRolePolicy;
java.lang.String assumeRoleRoleArn;
java.lang.Number assumeRoleSessionExpiration;
java.lang.String assumeRoleSessionName;
java.lang.String ecsRoleName;
java.lang.Boolean encrypt;
java.lang.String endpoint;
java.lang.String key;
java.lang.String prefix;
java.lang.String profile;
java.lang.String region;
java.lang.String secretKey;
java.lang.String securityToken;
java.lang.String sharedCredentialsFile;
java.lang.String stsEndpoint;
java.lang.String tablestoreEndpoint;
java.lang.String tablestoreTable;
/**
* Sets the value of {@link OssBackendConfig#getBucket}
* @param bucket (Required) The name of the OSS bucket. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder bucket(java.lang.String bucket) {
this.bucket = bucket;
return this;
}
/**
* Sets the value of {@link OssBackendConfig#getAccessKey}
* @param accessKey (Optional) Alibaba Cloud access key.
* It supports environment variables ALICLOUD_ACCESS_KEY and ALICLOUD_ACCESS_KEY_ID.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder accessKey(java.lang.String accessKey) {
this.accessKey = accessKey;
return this;
}
/**
* Sets the value of {@link OssBackendConfig#getAcl}
* @param acl (Optional) Object ACL to be applied to the state file.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder acl(java.lang.String acl) {
this.acl = acl;
return this;
}
/**
* Sets the value of {@link OssBackendConfig#getAssumeRole}
* @param assumeRole the value to be set.
* @return {@code this}
* @deprecated Use flattened assume role options
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder assumeRole(com.hashicorp.cdktf.OssAssumeRole assumeRole) {
this.assumeRole = assumeRole;
return this;
}
/**
* Sets the value of {@link OssBackendConfig#getAssumeRolePolicy}
* @param assumeRolePolicy (Optional, Available in 1.1.0+) A more restrictive policy to apply to the temporary credentials. This gives you a way to further restrict the permissions for the resulting temporary security credentials. You cannot use this policy to grant permissions that exceed those of the role that is being assumed.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder assumeRolePolicy(java.lang.String assumeRolePolicy) {
this.assumeRolePolicy = assumeRolePolicy;
return this;
}
/**
* Sets the value of {@link OssBackendConfig#getAssumeRoleRoleArn}
* @param assumeRoleRoleArn (Optional, Available in 1.1.0+) The ARN of the role to assume. If ARN is set to an empty string, it does not perform role switching. It supports the environment variable ALICLOUD_ASSUME_ROLE_ARN. Terraform executes configuration on account with provided credentials.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder assumeRoleRoleArn(java.lang.String assumeRoleRoleArn) {
this.assumeRoleRoleArn = assumeRoleRoleArn;
return this;
}
/**
* Sets the value of {@link OssBackendConfig#getAssumeRoleSessionExpiration}
* @param assumeRoleSessionExpiration (Optional, Available in 1.1.0+) The time after which the established session for assuming role expires. Valid value range: [900-3600] seconds. Default to 3600 (in this case Alibaba Cloud uses its own default value). It supports environment variable ALICLOUD_ASSUME_ROLE_SESSION_EXPIRATION.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder assumeRoleSessionExpiration(java.lang.Number assumeRoleSessionExpiration) {
this.assumeRoleSessionExpiration = assumeRoleSessionExpiration;
return this;
}
/**
* Sets the value of {@link OssBackendConfig#getAssumeRoleSessionName}
* @param assumeRoleSessionName (Optional, Available in 1.1.0+) The session name to use when assuming the role. If omitted, 'terraform' is passed to the AssumeRole call as session name. It supports environment variable ALICLOUD_ASSUME_ROLE_SESSION_NAME.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder assumeRoleSessionName(java.lang.String assumeRoleSessionName) {
this.assumeRoleSessionName = assumeRoleSessionName;
return this;
}
/**
* Sets the value of {@link OssBackendConfig#getEcsRoleName}
* @param ecsRoleName (Optional, Available in 0.12.14+) The RAM Role Name attached on a ECS instance for API operations. You can retrieve this from the 'Access Control' section of the Alibaba Cloud console.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder ecsRoleName(java.lang.String ecsRoleName) {
this.ecsRoleName = ecsRoleName;
return this;
}
/**
* Sets the value of {@link OssBackendConfig#getEncrypt}
* @param encrypt (Optional) Whether to enable server side encryption of the state file.
* If it is true, OSS will use 'AES256' encryption algorithm to encrypt state file.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder encrypt(java.lang.Boolean encrypt) {
this.encrypt = encrypt;
return this;
}
/**
* Sets the value of {@link OssBackendConfig#getEndpoint}
* @param endpoint (Optional) A custom endpoint for the OSS API.
* It supports environment variables ALICLOUD_OSS_ENDPOINT and OSS_ENDPOINT.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder endpoint(java.lang.String endpoint) {
this.endpoint = endpoint;
return this;
}
/**
* Sets the value of {@link OssBackendConfig#getKey}
* @param key (Optional) The name of the state file.
* Defaults to terraform.tfstate.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder key(java.lang.String key) {
this.key = key;
return this;
}
/**
* Sets the value of {@link OssBackendConfig#getPrefix}
* @param prefix (Optional) The path directory of the state file will be stored.
* Default to "env:".
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder prefix(java.lang.String prefix) {
this.prefix = prefix;
return this;
}
/**
* Sets the value of {@link OssBackendConfig#getProfile}
* @param profile (Optional, Available in 0.12.8+) This is the Alibaba Cloud profile name as set in the shared credentials file. It can also be sourced from the ALICLOUD_PROFILE environment variable.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder profile(java.lang.String profile) {
this.profile = profile;
return this;
}
/**
* Sets the value of {@link OssBackendConfig#getRegion}
* @param region (Optional) The region of the OSS bucket.
* It supports environment variables ALICLOUD_REGION and ALICLOUD_DEFAULT_REGION.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder region(java.lang.String region) {
this.region = region;
return this;
}
/**
* Sets the value of {@link OssBackendConfig#getSecretKey}
* @param secretKey (Optional) Alibaba Cloud secret access key.
* It supports environment variables ALICLOUD_SECRET_KEY and ALICLOUD_ACCESS_KEY_SECRET.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder secretKey(java.lang.String secretKey) {
this.secretKey = secretKey;
return this;
}
/**
* Sets the value of {@link OssBackendConfig#getSecurityToken}
* @param securityToken (Optional) STS access token.
* It supports environment variable ALICLOUD_SECURITY_TOKEN.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder securityToken(java.lang.String securityToken) {
this.securityToken = securityToken;
return this;
}
/**
* Sets the value of {@link OssBackendConfig#getSharedCredentialsFile}
* @param sharedCredentialsFile (Optional, Available in 0.12.8+) This is the path to the shared credentials file. It can also be sourced from the ALICLOUD_SHARED_CREDENTIALS_FILE environment variable. If this is not set and a profile is specified, ~/.aliyun/config.json will be used.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder sharedCredentialsFile(java.lang.String sharedCredentialsFile) {
this.sharedCredentialsFile = sharedCredentialsFile;
return this;
}
/**
* Sets the value of {@link OssBackendConfig#getStsEndpoint}
* @param stsEndpoint (Optional, Available in 1.0.11+) Custom endpoint for the AliCloud Security Token Service (STS) API. It supports environment variable ALICLOUD_STS_ENDPOINT.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder stsEndpoint(java.lang.String stsEndpoint) {
this.stsEndpoint = stsEndpoint;
return this;
}
/**
* Sets the value of {@link OssBackendConfig#getTablestoreEndpoint}
* @param tablestoreEndpoint (Optional) A custom endpoint for the TableStore API.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder tablestoreEndpoint(java.lang.String tablestoreEndpoint) {
this.tablestoreEndpoint = tablestoreEndpoint;
return this;
}
/**
* Sets the value of {@link OssBackendConfig#getTablestoreTable}
* @param tablestoreTable (Optional) A TableStore table for state locking and consistency.
* The table must have a primary key named LockID of type String.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder tablestoreTable(java.lang.String tablestoreTable) {
this.tablestoreTable = tablestoreTable;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link OssBackendConfig}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public OssBackendConfig build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link OssBackendConfig}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements OssBackendConfig {
private final java.lang.String bucket;
private final java.lang.String accessKey;
private final java.lang.String acl;
private final com.hashicorp.cdktf.OssAssumeRole assumeRole;
private final java.lang.String assumeRolePolicy;
private final java.lang.String assumeRoleRoleArn;
private final java.lang.Number assumeRoleSessionExpiration;
private final java.lang.String assumeRoleSessionName;
private final java.lang.String ecsRoleName;
private final java.lang.Boolean encrypt;
private final java.lang.String endpoint;
private final java.lang.String key;
private final java.lang.String prefix;
private final java.lang.String profile;
private final java.lang.String region;
private final java.lang.String secretKey;
private final java.lang.String securityToken;
private final java.lang.String sharedCredentialsFile;
private final java.lang.String stsEndpoint;
private final java.lang.String tablestoreEndpoint;
private final java.lang.String tablestoreTable;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.bucket = software.amazon.jsii.Kernel.get(this, "bucket", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.accessKey = software.amazon.jsii.Kernel.get(this, "accessKey", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.acl = software.amazon.jsii.Kernel.get(this, "acl", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.assumeRole = software.amazon.jsii.Kernel.get(this, "assumeRole", software.amazon.jsii.NativeType.forClass(com.hashicorp.cdktf.OssAssumeRole.class));
this.assumeRolePolicy = software.amazon.jsii.Kernel.get(this, "assumeRolePolicy", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.assumeRoleRoleArn = software.amazon.jsii.Kernel.get(this, "assumeRoleRoleArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.assumeRoleSessionExpiration = software.amazon.jsii.Kernel.get(this, "assumeRoleSessionExpiration", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.assumeRoleSessionName = software.amazon.jsii.Kernel.get(this, "assumeRoleSessionName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.ecsRoleName = software.amazon.jsii.Kernel.get(this, "ecsRoleName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.encrypt = software.amazon.jsii.Kernel.get(this, "encrypt", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.endpoint = software.amazon.jsii.Kernel.get(this, "endpoint", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.key = software.amazon.jsii.Kernel.get(this, "key", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.prefix = software.amazon.jsii.Kernel.get(this, "prefix", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.profile = software.amazon.jsii.Kernel.get(this, "profile", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.region = software.amazon.jsii.Kernel.get(this, "region", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.secretKey = software.amazon.jsii.Kernel.get(this, "secretKey", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.securityToken = software.amazon.jsii.Kernel.get(this, "securityToken", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.sharedCredentialsFile = software.amazon.jsii.Kernel.get(this, "sharedCredentialsFile", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.stsEndpoint = software.amazon.jsii.Kernel.get(this, "stsEndpoint", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.tablestoreEndpoint = software.amazon.jsii.Kernel.get(this, "tablestoreEndpoint", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.tablestoreTable = software.amazon.jsii.Kernel.get(this, "tablestoreTable", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.bucket = java.util.Objects.requireNonNull(builder.bucket, "bucket is required");
this.accessKey = builder.accessKey;
this.acl = builder.acl;
this.assumeRole = builder.assumeRole;
this.assumeRolePolicy = builder.assumeRolePolicy;
this.assumeRoleRoleArn = builder.assumeRoleRoleArn;
this.assumeRoleSessionExpiration = builder.assumeRoleSessionExpiration;
this.assumeRoleSessionName = builder.assumeRoleSessionName;
this.ecsRoleName = builder.ecsRoleName;
this.encrypt = builder.encrypt;
this.endpoint = builder.endpoint;
this.key = builder.key;
this.prefix = builder.prefix;
this.profile = builder.profile;
this.region = builder.region;
this.secretKey = builder.secretKey;
this.securityToken = builder.securityToken;
this.sharedCredentialsFile = builder.sharedCredentialsFile;
this.stsEndpoint = builder.stsEndpoint;
this.tablestoreEndpoint = builder.tablestoreEndpoint;
this.tablestoreTable = builder.tablestoreTable;
}
@Override
public final java.lang.String getBucket() {
return this.bucket;
}
@Override
public final java.lang.String getAccessKey() {
return this.accessKey;
}
@Override
public final java.lang.String getAcl() {
return this.acl;
}
@Override
public final com.hashicorp.cdktf.OssAssumeRole getAssumeRole() {
return this.assumeRole;
}
@Override
public final java.lang.String getAssumeRolePolicy() {
return this.assumeRolePolicy;
}
@Override
public final java.lang.String getAssumeRoleRoleArn() {
return this.assumeRoleRoleArn;
}
@Override
public final java.lang.Number getAssumeRoleSessionExpiration() {
return this.assumeRoleSessionExpiration;
}
@Override
public final java.lang.String getAssumeRoleSessionName() {
return this.assumeRoleSessionName;
}
@Override
public final java.lang.String getEcsRoleName() {
return this.ecsRoleName;
}
@Override
public final java.lang.Boolean getEncrypt() {
return this.encrypt;
}
@Override
public final java.lang.String getEndpoint() {
return this.endpoint;
}
@Override
public final java.lang.String getKey() {
return this.key;
}
@Override
public final java.lang.String getPrefix() {
return this.prefix;
}
@Override
public final java.lang.String getProfile() {
return this.profile;
}
@Override
public final java.lang.String getRegion() {
return this.region;
}
@Override
public final java.lang.String getSecretKey() {
return this.secretKey;
}
@Override
public final java.lang.String getSecurityToken() {
return this.securityToken;
}
@Override
public final java.lang.String getSharedCredentialsFile() {
return this.sharedCredentialsFile;
}
@Override
public final java.lang.String getStsEndpoint() {
return this.stsEndpoint;
}
@Override
public final java.lang.String getTablestoreEndpoint() {
return this.tablestoreEndpoint;
}
@Override
public final java.lang.String getTablestoreTable() {
return this.tablestoreTable;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("bucket", om.valueToTree(this.getBucket()));
if (this.getAccessKey() != null) {
data.set("accessKey", om.valueToTree(this.getAccessKey()));
}
if (this.getAcl() != null) {
data.set("acl", om.valueToTree(this.getAcl()));
}
if (this.getAssumeRole() != null) {
data.set("assumeRole", om.valueToTree(this.getAssumeRole()));
}
if (this.getAssumeRolePolicy() != null) {
data.set("assumeRolePolicy", om.valueToTree(this.getAssumeRolePolicy()));
}
if (this.getAssumeRoleRoleArn() != null) {
data.set("assumeRoleRoleArn", om.valueToTree(this.getAssumeRoleRoleArn()));
}
if (this.getAssumeRoleSessionExpiration() != null) {
data.set("assumeRoleSessionExpiration", om.valueToTree(this.getAssumeRoleSessionExpiration()));
}
if (this.getAssumeRoleSessionName() != null) {
data.set("assumeRoleSessionName", om.valueToTree(this.getAssumeRoleSessionName()));
}
if (this.getEcsRoleName() != null) {
data.set("ecsRoleName", om.valueToTree(this.getEcsRoleName()));
}
if (this.getEncrypt() != null) {
data.set("encrypt", om.valueToTree(this.getEncrypt()));
}
if (this.getEndpoint() != null) {
data.set("endpoint", om.valueToTree(this.getEndpoint()));
}
if (this.getKey() != null) {
data.set("key", om.valueToTree(this.getKey()));
}
if (this.getPrefix() != null) {
data.set("prefix", om.valueToTree(this.getPrefix()));
}
if (this.getProfile() != null) {
data.set("profile", om.valueToTree(this.getProfile()));
}
if (this.getRegion() != null) {
data.set("region", om.valueToTree(this.getRegion()));
}
if (this.getSecretKey() != null) {
data.set("secretKey", om.valueToTree(this.getSecretKey()));
}
if (this.getSecurityToken() != null) {
data.set("securityToken", om.valueToTree(this.getSecurityToken()));
}
if (this.getSharedCredentialsFile() != null) {
data.set("sharedCredentialsFile", om.valueToTree(this.getSharedCredentialsFile()));
}
if (this.getStsEndpoint() != null) {
data.set("stsEndpoint", om.valueToTree(this.getStsEndpoint()));
}
if (this.getTablestoreEndpoint() != null) {
data.set("tablestoreEndpoint", om.valueToTree(this.getTablestoreEndpoint()));
}
if (this.getTablestoreTable() != null) {
data.set("tablestoreTable", om.valueToTree(this.getTablestoreTable()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("cdktf.OssBackendConfig"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
OssBackendConfig.Jsii$Proxy that = (OssBackendConfig.Jsii$Proxy) o;
if (!bucket.equals(that.bucket)) return false;
if (this.accessKey != null ? !this.accessKey.equals(that.accessKey) : that.accessKey != null) return false;
if (this.acl != null ? !this.acl.equals(that.acl) : that.acl != null) return false;
if (this.assumeRole != null ? !this.assumeRole.equals(that.assumeRole) : that.assumeRole != null) return false;
if (this.assumeRolePolicy != null ? !this.assumeRolePolicy.equals(that.assumeRolePolicy) : that.assumeRolePolicy != null) return false;
if (this.assumeRoleRoleArn != null ? !this.assumeRoleRoleArn.equals(that.assumeRoleRoleArn) : that.assumeRoleRoleArn != null) return false;
if (this.assumeRoleSessionExpiration != null ? !this.assumeRoleSessionExpiration.equals(that.assumeRoleSessionExpiration) : that.assumeRoleSessionExpiration != null) return false;
if (this.assumeRoleSessionName != null ? !this.assumeRoleSessionName.equals(that.assumeRoleSessionName) : that.assumeRoleSessionName != null) return false;
if (this.ecsRoleName != null ? !this.ecsRoleName.equals(that.ecsRoleName) : that.ecsRoleName != null) return false;
if (this.encrypt != null ? !this.encrypt.equals(that.encrypt) : that.encrypt != null) return false;
if (this.endpoint != null ? !this.endpoint.equals(that.endpoint) : that.endpoint != null) return false;
if (this.key != null ? !this.key.equals(that.key) : that.key != null) return false;
if (this.prefix != null ? !this.prefix.equals(that.prefix) : that.prefix != null) return false;
if (this.profile != null ? !this.profile.equals(that.profile) : that.profile != null) return false;
if (this.region != null ? !this.region.equals(that.region) : that.region != null) return false;
if (this.secretKey != null ? !this.secretKey.equals(that.secretKey) : that.secretKey != null) return false;
if (this.securityToken != null ? !this.securityToken.equals(that.securityToken) : that.securityToken != null) return false;
if (this.sharedCredentialsFile != null ? !this.sharedCredentialsFile.equals(that.sharedCredentialsFile) : that.sharedCredentialsFile != null) return false;
if (this.stsEndpoint != null ? !this.stsEndpoint.equals(that.stsEndpoint) : that.stsEndpoint != null) return false;
if (this.tablestoreEndpoint != null ? !this.tablestoreEndpoint.equals(that.tablestoreEndpoint) : that.tablestoreEndpoint != null) return false;
return this.tablestoreTable != null ? this.tablestoreTable.equals(that.tablestoreTable) : that.tablestoreTable == null;
}
@Override
public final int hashCode() {
int result = this.bucket.hashCode();
result = 31 * result + (this.accessKey != null ? this.accessKey.hashCode() : 0);
result = 31 * result + (this.acl != null ? this.acl.hashCode() : 0);
result = 31 * result + (this.assumeRole != null ? this.assumeRole.hashCode() : 0);
result = 31 * result + (this.assumeRolePolicy != null ? this.assumeRolePolicy.hashCode() : 0);
result = 31 * result + (this.assumeRoleRoleArn != null ? this.assumeRoleRoleArn.hashCode() : 0);
result = 31 * result + (this.assumeRoleSessionExpiration != null ? this.assumeRoleSessionExpiration.hashCode() : 0);
result = 31 * result + (this.assumeRoleSessionName != null ? this.assumeRoleSessionName.hashCode() : 0);
result = 31 * result + (this.ecsRoleName != null ? this.ecsRoleName.hashCode() : 0);
result = 31 * result + (this.encrypt != null ? this.encrypt.hashCode() : 0);
result = 31 * result + (this.endpoint != null ? this.endpoint.hashCode() : 0);
result = 31 * result + (this.key != null ? this.key.hashCode() : 0);
result = 31 * result + (this.prefix != null ? this.prefix.hashCode() : 0);
result = 31 * result + (this.profile != null ? this.profile.hashCode() : 0);
result = 31 * result + (this.region != null ? this.region.hashCode() : 0);
result = 31 * result + (this.secretKey != null ? this.secretKey.hashCode() : 0);
result = 31 * result + (this.securityToken != null ? this.securityToken.hashCode() : 0);
result = 31 * result + (this.sharedCredentialsFile != null ? this.sharedCredentialsFile.hashCode() : 0);
result = 31 * result + (this.stsEndpoint != null ? this.stsEndpoint.hashCode() : 0);
result = 31 * result + (this.tablestoreEndpoint != null ? this.tablestoreEndpoint.hashCode() : 0);
result = 31 * result + (this.tablestoreTable != null ? this.tablestoreTable.hashCode() : 0);
return result;
}
}
}