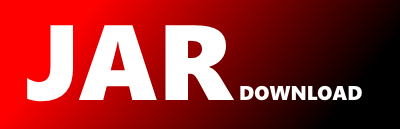
com.hashmapinc.tempus.WitsmlObjects.v1311.CsFiberInformation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of WitsmlObjects Show documentation
Show all versions of WitsmlObjects Show documentation
This library assists in serializing and deserializing WITSML 1.3.1.1 and 1.4.1.1 Objects
package com.hashmapinc.tempus.WitsmlObjects.v1311;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
/**
* The optical fiber used for distributed temperature surveys
*
* Java class for cs_fiberInformation complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
{@code
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "cs_fiberInformation", propOrder = {
"installationDate",
"installationCompany",
"deInstallationDate",
"capillaryTubeDiameter",
"fiber"
})
public class CsFiberInformation {
protected String installationDate;
protected String installationCompany;
protected String deInstallationDate;
protected LengthMeasure capillaryTubeDiameter;
protected List fiber;
/**
* Gets the value of the installationDate property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getInstallationDate() {
return installationDate;
}
/**
* Sets the value of the installationDate property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setInstallationDate(String value) {
this.installationDate = value;
}
/**
* Gets the value of the installationCompany property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getInstallationCompany() {
return installationCompany;
}
/**
* Sets the value of the installationCompany property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setInstallationCompany(String value) {
this.installationCompany = value;
}
/**
* Gets the value of the deInstallationDate property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDeInstallationDate() {
return deInstallationDate;
}
/**
* Sets the value of the deInstallationDate property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDeInstallationDate(String value) {
this.deInstallationDate = value;
}
/**
* Gets the value of the capillaryTubeDiameter property.
*
* @return
* possible object is
* {@link LengthMeasure }
*
*/
public LengthMeasure getCapillaryTubeDiameter() {
return capillaryTubeDiameter;
}
/**
* Sets the value of the capillaryTubeDiameter property.
*
* @param value
* allowed object is
* {@link LengthMeasure }
*
*/
public void setCapillaryTubeDiameter(LengthMeasure value) {
this.capillaryTubeDiameter = value;
}
/**
* Gets the value of the fiber property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the fiber property.
*
*
* For example, to add a new item, do as follows:
* {@code
* getFiber().add(newItem);
* }
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CsFiber }
*
*
*/
public List getFiber() {
if (fiber == null) {
fiber = new ArrayList();
}
return this.fiber;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy