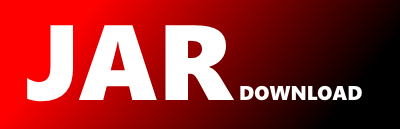
com.hashmapinc.tempus.WitsmlObjects.v1311.ObjMessage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of WitsmlObjects Show documentation
Show all versions of WitsmlObjects Show documentation
This library assists in serializing and deserializing WITSML 1.3.1.1 and 1.4.1.1 Objects
package com.hashmapinc.tempus.WitsmlObjects.v1311;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
/**
* Java class for obj_message complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
{@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "obj_message", propOrder = {
"nameWell",
"nameWellbore",
"name",
"objectReference",
"subObjectReference",
"dTim",
"activityCode",
"detailActivity",
"md",
"mdBit",
"typeMessage",
"messageText",
"param",
"severity",
"warnProbability",
"commonData",
"customData"
})
public class ObjMessage {
@XmlElement(required = true)
protected String nameWell;
@XmlElement(required = true)
protected String nameWellbore;
@XmlElement(required = true)
protected String name;
protected RefObjectString objectReference;
protected RefObjectString subObjectReference;
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar dTim;
protected String activityCode;
protected String detailActivity;
protected MeasuredDepthCoord md;
protected MeasuredDepthCoord mdBit;
@XmlSchemaType(name = "string")
protected MessageType typeMessage;
protected String messageText;
protected List param;
@XmlSchemaType(name = "string")
protected MessageSeverity severity;
@XmlSchemaType(name = "string")
protected MessageProbability warnProbability;
protected CsCommonData commonData;
protected CsCustomData customData;
@XmlAttribute(name = "uidWell")
protected String uidWell;
@XmlAttribute(name = "uidWellbore")
protected String uidWellbore;
@XmlAttribute(name = "uid")
protected String uid;
/**
* Gets the value of the nameWell property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNameWell() {
return nameWell;
}
/**
* Sets the value of the nameWell property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNameWell(String value) {
this.nameWell = value;
}
/**
* Gets the value of the nameWellbore property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNameWellbore() {
return nameWellbore;
}
/**
* Sets the value of the nameWellbore property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNameWellbore(String value) {
this.nameWellbore = value;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the objectReference property.
*
* @return
* possible object is
* {@link RefObjectString }
*
*/
public RefObjectString getObjectReference() {
return objectReference;
}
/**
* Sets the value of the objectReference property.
*
* @param value
* allowed object is
* {@link RefObjectString }
*
*/
public void setObjectReference(RefObjectString value) {
this.objectReference = value;
}
/**
* Gets the value of the subObjectReference property.
*
* @return
* possible object is
* {@link RefObjectString }
*
*/
public RefObjectString getSubObjectReference() {
return subObjectReference;
}
/**
* Sets the value of the subObjectReference property.
*
* @param value
* allowed object is
* {@link RefObjectString }
*
*/
public void setSubObjectReference(RefObjectString value) {
this.subObjectReference = value;
}
/**
* Gets the value of the dTim property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getDTim() {
return dTim;
}
/**
* Sets the value of the dTim property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setDTim(XMLGregorianCalendar value) {
this.dTim = value;
}
/**
* Gets the value of the activityCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getActivityCode() {
return activityCode;
}
/**
* Sets the value of the activityCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setActivityCode(String value) {
this.activityCode = value;
}
/**
* Gets the value of the detailActivity property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDetailActivity() {
return detailActivity;
}
/**
* Sets the value of the detailActivity property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDetailActivity(String value) {
this.detailActivity = value;
}
/**
* Gets the value of the md property.
*
* @return
* possible object is
* {@link MeasuredDepthCoord }
*
*/
public MeasuredDepthCoord getMd() {
return md;
}
/**
* Sets the value of the md property.
*
* @param value
* allowed object is
* {@link MeasuredDepthCoord }
*
*/
public void setMd(MeasuredDepthCoord value) {
this.md = value;
}
/**
* Gets the value of the mdBit property.
*
* @return
* possible object is
* {@link MeasuredDepthCoord }
*
*/
public MeasuredDepthCoord getMdBit() {
return mdBit;
}
/**
* Sets the value of the mdBit property.
*
* @param value
* allowed object is
* {@link MeasuredDepthCoord }
*
*/
public void setMdBit(MeasuredDepthCoord value) {
this.mdBit = value;
}
/**
* Gets the value of the typeMessage property.
*
* @return
* possible object is
* {@link MessageType }
*
*/
public MessageType getTypeMessage() {
return typeMessage;
}
/**
* Sets the value of the typeMessage property.
*
* @param value
* allowed object is
* {@link MessageType }
*
*/
public void setTypeMessage(MessageType value) {
this.typeMessage = value;
}
/**
* Gets the value of the messageText property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMessageText() {
return messageText;
}
/**
* Sets the value of the messageText property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMessageText(String value) {
this.messageText = value;
}
/**
* Gets the value of the param property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the param property.
*
*
* For example, to add a new item, do as follows:
* {@code
* getParam().add(newItem);
* }
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link IndexedObject }
*
*
*/
public List getParam() {
if (param == null) {
param = new ArrayList();
}
return this.param;
}
/**
* Gets the value of the severity property.
*
* @return
* possible object is
* {@link MessageSeverity }
*
*/
public MessageSeverity getSeverity() {
return severity;
}
/**
* Sets the value of the severity property.
*
* @param value
* allowed object is
* {@link MessageSeverity }
*
*/
public void setSeverity(MessageSeverity value) {
this.severity = value;
}
/**
* Gets the value of the warnProbability property.
*
* @return
* possible object is
* {@link MessageProbability }
*
*/
public MessageProbability getWarnProbability() {
return warnProbability;
}
/**
* Sets the value of the warnProbability property.
*
* @param value
* allowed object is
* {@link MessageProbability }
*
*/
public void setWarnProbability(MessageProbability value) {
this.warnProbability = value;
}
/**
* Gets the value of the commonData property.
*
* @return
* possible object is
* {@link CsCommonData }
*
*/
public CsCommonData getCommonData() {
return commonData;
}
/**
* Sets the value of the commonData property.
*
* @param value
* allowed object is
* {@link CsCommonData }
*
*/
public void setCommonData(CsCommonData value) {
this.commonData = value;
}
/**
* Gets the value of the customData property.
*
* @return
* possible object is
* {@link CsCustomData }
*
*/
public CsCustomData getCustomData() {
return customData;
}
/**
* Sets the value of the customData property.
*
* @param value
* allowed object is
* {@link CsCustomData }
*
*/
public void setCustomData(CsCustomData value) {
this.customData = value;
}
/**
* Gets the value of the uidWell property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUidWell() {
return uidWell;
}
/**
* Sets the value of the uidWell property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUidWell(String value) {
this.uidWell = value;
}
/**
* Gets the value of the uidWellbore property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUidWellbore() {
return uidWellbore;
}
/**
* Sets the value of the uidWellbore property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUidWellbore(String value) {
this.uidWellbore = value;
}
/**
* Gets the value of the uid property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUid() {
return uid;
}
/**
* Sets the value of the uid property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUid(String value) {
this.uid = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy