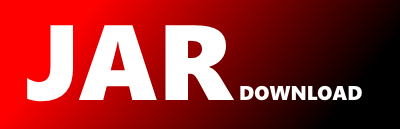
com.hashmapinc.tempus.WitsmlObjects.v1411.CsRotarySteerableTool Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of WitsmlObjects Show documentation
Show all versions of WitsmlObjects Show documentation
This library assists in serializing and deserializing WITSML 1.3.1.1 and 1.4.1.1 Objects
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.8-b130911.1802
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2017.06.26 at 03:00:38 PM CDT
//
package com.hashmapinc.tempus.WitsmlObjects.v1411;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
/**
* WITSML - Rotary Steerable Tool Component Schema
*
* Java class for cs_rotarySteerableTool complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
{@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "cs_rotarySteerableTool", propOrder = {
"deflectionMethod",
"bendAngle",
"bendOffset",
"holeSizeMn",
"holeSizeMx",
"wobMx",
"operatingSpeed",
"speedMx",
"flowRateMn",
"flowRateMx",
"downLinkFlowRateMn",
"downLinkFlowRateMx",
"pressLossFact",
"padCount",
"padLen",
"padWidth",
"padOffset",
"openPadOd",
"closePadOd",
"sensor",
"customData",
"extensionAny"
})
public class CsRotarySteerableTool {
@XmlElement(required = true)
@XmlSchemaType(name = "string")
protected DeflectionMethod deflectionMethod;
protected PlaneAngleMeasure bendAngle;
protected LengthMeasure bendOffset;
protected LengthMeasure holeSizeMn;
protected LengthMeasure holeSizeMx;
protected ForceMeasure wobMx;
protected AnglePerTimeMeasure operatingSpeed;
protected AnglePerTimeMeasure speedMx;
protected VolumeFlowRateMeasure flowRateMn;
protected VolumeFlowRateMeasure flowRateMx;
protected VolumeFlowRateMeasure downLinkFlowRateMn;
protected VolumeFlowRateMeasure downLinkFlowRateMx;
protected Double pressLossFact;
protected Short padCount;
protected LengthMeasure padLen;
protected LengthMeasure padWidth;
protected LengthMeasure padOffset;
protected LengthMeasure openPadOd;
protected LengthMeasure closePadOd;
protected List sensor;
protected CsCustomData customData;
protected CsExtensionAny extensionAny;
/**
* Gets the value of the deflectionMethod property.
*
* @return
* possible object is
* {@link DeflectionMethod }
*
*/
public DeflectionMethod getDeflectionMethod() {
return deflectionMethod;
}
/**
* Sets the value of the deflectionMethod property.
*
* @param value
* allowed object is
* {@link DeflectionMethod }
*
*/
public void setDeflectionMethod(DeflectionMethod value) {
this.deflectionMethod = value;
}
/**
* Gets the value of the bendAngle property.
*
* @return
* possible object is
* {@link PlaneAngleMeasure }
*
*/
public PlaneAngleMeasure getBendAngle() {
return bendAngle;
}
/**
* Sets the value of the bendAngle property.
*
* @param value
* allowed object is
* {@link PlaneAngleMeasure }
*
*/
public void setBendAngle(PlaneAngleMeasure value) {
this.bendAngle = value;
}
/**
* Gets the value of the bendOffset property.
*
* @return
* possible object is
* {@link LengthMeasure }
*
*/
public LengthMeasure getBendOffset() {
return bendOffset;
}
/**
* Sets the value of the bendOffset property.
*
* @param value
* allowed object is
* {@link LengthMeasure }
*
*/
public void setBendOffset(LengthMeasure value) {
this.bendOffset = value;
}
/**
* Gets the value of the holeSizeMn property.
*
* @return
* possible object is
* {@link LengthMeasure }
*
*/
public LengthMeasure getHoleSizeMn() {
return holeSizeMn;
}
/**
* Sets the value of the holeSizeMn property.
*
* @param value
* allowed object is
* {@link LengthMeasure }
*
*/
public void setHoleSizeMn(LengthMeasure value) {
this.holeSizeMn = value;
}
/**
* Gets the value of the holeSizeMx property.
*
* @return
* possible object is
* {@link LengthMeasure }
*
*/
public LengthMeasure getHoleSizeMx() {
return holeSizeMx;
}
/**
* Sets the value of the holeSizeMx property.
*
* @param value
* allowed object is
* {@link LengthMeasure }
*
*/
public void setHoleSizeMx(LengthMeasure value) {
this.holeSizeMx = value;
}
/**
* Gets the value of the wobMx property.
*
* @return
* possible object is
* {@link ForceMeasure }
*
*/
public ForceMeasure getWobMx() {
return wobMx;
}
/**
* Sets the value of the wobMx property.
*
* @param value
* allowed object is
* {@link ForceMeasure }
*
*/
public void setWobMx(ForceMeasure value) {
this.wobMx = value;
}
/**
* Gets the value of the operatingSpeed property.
*
* @return
* possible object is
* {@link AnglePerTimeMeasure }
*
*/
public AnglePerTimeMeasure getOperatingSpeed() {
return operatingSpeed;
}
/**
* Sets the value of the operatingSpeed property.
*
* @param value
* allowed object is
* {@link AnglePerTimeMeasure }
*
*/
public void setOperatingSpeed(AnglePerTimeMeasure value) {
this.operatingSpeed = value;
}
/**
* Gets the value of the speedMx property.
*
* @return
* possible object is
* {@link AnglePerTimeMeasure }
*
*/
public AnglePerTimeMeasure getSpeedMx() {
return speedMx;
}
/**
* Sets the value of the speedMx property.
*
* @param value
* allowed object is
* {@link AnglePerTimeMeasure }
*
*/
public void setSpeedMx(AnglePerTimeMeasure value) {
this.speedMx = value;
}
/**
* Gets the value of the flowRateMn property.
*
* @return
* possible object is
* {@link VolumeFlowRateMeasure }
*
*/
public VolumeFlowRateMeasure getFlowRateMn() {
return flowRateMn;
}
/**
* Sets the value of the flowRateMn property.
*
* @param value
* allowed object is
* {@link VolumeFlowRateMeasure }
*
*/
public void setFlowRateMn(VolumeFlowRateMeasure value) {
this.flowRateMn = value;
}
/**
* Gets the value of the flowRateMx property.
*
* @return
* possible object is
* {@link VolumeFlowRateMeasure }
*
*/
public VolumeFlowRateMeasure getFlowRateMx() {
return flowRateMx;
}
/**
* Sets the value of the flowRateMx property.
*
* @param value
* allowed object is
* {@link VolumeFlowRateMeasure }
*
*/
public void setFlowRateMx(VolumeFlowRateMeasure value) {
this.flowRateMx = value;
}
/**
* Gets the value of the downLinkFlowRateMn property.
*
* @return
* possible object is
* {@link VolumeFlowRateMeasure }
*
*/
public VolumeFlowRateMeasure getDownLinkFlowRateMn() {
return downLinkFlowRateMn;
}
/**
* Sets the value of the downLinkFlowRateMn property.
*
* @param value
* allowed object is
* {@link VolumeFlowRateMeasure }
*
*/
public void setDownLinkFlowRateMn(VolumeFlowRateMeasure value) {
this.downLinkFlowRateMn = value;
}
/**
* Gets the value of the downLinkFlowRateMx property.
*
* @return
* possible object is
* {@link VolumeFlowRateMeasure }
*
*/
public VolumeFlowRateMeasure getDownLinkFlowRateMx() {
return downLinkFlowRateMx;
}
/**
* Sets the value of the downLinkFlowRateMx property.
*
* @param value
* allowed object is
* {@link VolumeFlowRateMeasure }
*
*/
public void setDownLinkFlowRateMx(VolumeFlowRateMeasure value) {
this.downLinkFlowRateMx = value;
}
/**
* Gets the value of the pressLossFact property.
*
* @return
* possible object is
* {@link Double }
*
*/
public Double getPressLossFact() {
return pressLossFact;
}
/**
* Sets the value of the pressLossFact property.
*
* @param value
* allowed object is
* {@link Double }
*
*/
public void setPressLossFact(Double value) {
this.pressLossFact = value;
}
/**
* Gets the value of the padCount property.
*
* @return
* possible object is
* {@link Short }
*
*/
public Short getPadCount() {
return padCount;
}
/**
* Sets the value of the padCount property.
*
* @param value
* allowed object is
* {@link Short }
*
*/
public void setPadCount(Short value) {
this.padCount = value;
}
/**
* Gets the value of the padLen property.
*
* @return
* possible object is
* {@link LengthMeasure }
*
*/
public LengthMeasure getPadLen() {
return padLen;
}
/**
* Sets the value of the padLen property.
*
* @param value
* allowed object is
* {@link LengthMeasure }
*
*/
public void setPadLen(LengthMeasure value) {
this.padLen = value;
}
/**
* Gets the value of the padWidth property.
*
* @return
* possible object is
* {@link LengthMeasure }
*
*/
public LengthMeasure getPadWidth() {
return padWidth;
}
/**
* Sets the value of the padWidth property.
*
* @param value
* allowed object is
* {@link LengthMeasure }
*
*/
public void setPadWidth(LengthMeasure value) {
this.padWidth = value;
}
/**
* Gets the value of the padOffset property.
*
* @return
* possible object is
* {@link LengthMeasure }
*
*/
public LengthMeasure getPadOffset() {
return padOffset;
}
/**
* Sets the value of the padOffset property.
*
* @param value
* allowed object is
* {@link LengthMeasure }
*
*/
public void setPadOffset(LengthMeasure value) {
this.padOffset = value;
}
/**
* Gets the value of the openPadOd property.
*
* @return
* possible object is
* {@link LengthMeasure }
*
*/
public LengthMeasure getOpenPadOd() {
return openPadOd;
}
/**
* Sets the value of the openPadOd property.
*
* @param value
* allowed object is
* {@link LengthMeasure }
*
*/
public void setOpenPadOd(LengthMeasure value) {
this.openPadOd = value;
}
/**
* Gets the value of the closePadOd property.
*
* @return
* possible object is
* {@link LengthMeasure }
*
*/
public LengthMeasure getClosePadOd() {
return closePadOd;
}
/**
* Sets the value of the closePadOd property.
*
* @param value
* allowed object is
* {@link LengthMeasure }
*
*/
public void setClosePadOd(LengthMeasure value) {
this.closePadOd = value;
}
/**
* Gets the value of the sensor property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the sensor property.
*
*
* For example, to add a new item, do as follows:
* {@code
* getSensor().add(newItem);
* }
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CsSensor }
*
*
*/
public List getSensor() {
if (sensor == null) {
sensor = new ArrayList();
}
return this.sensor;
}
/**
* Gets the value of the customData property.
*
* @return
* possible object is
* {@link CsCustomData }
*
*/
public CsCustomData getCustomData() {
return customData;
}
/**
* Sets the value of the customData property.
*
* @param value
* allowed object is
* {@link CsCustomData }
*
*/
public void setCustomData(CsCustomData value) {
this.customData = value;
}
/**
* Gets the value of the extensionAny property.
*
* @return
* possible object is
* {@link CsExtensionAny }
*
*/
public CsExtensionAny getExtensionAny() {
return extensionAny;
}
/**
* Sets the value of the extensionAny property.
*
* @param value
* allowed object is
* {@link CsExtensionAny }
*
*/
public void setExtensionAny(CsExtensionAny value) {
this.extensionAny = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy