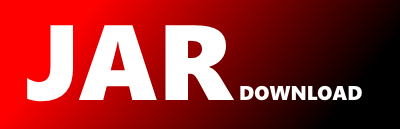
com.hashmapinc.tempus.WitsmlObjects.v1411.CsStimJobInterval Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.8-b130911.1802
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2017.06.26 at 03:00:38 PM CDT
//
package com.hashmapinc.tempus.WitsmlObjects.v1411;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
/**
* Information for a well stimulation job treatment interval
*
* Java class for cs_stimJobInterval complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
{@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "cs_stimJobInterval", propOrder = {
"uidTreatmentInterval",
"name",
"number",
"dTimStart",
"dTimEnd",
"formationName",
"mdFormationTop",
"mdFormationBottom",
"tvdFormationTop",
"tvdFormationBottom",
"openHoleName",
"openHoleDiameter",
"mdOpenHoleTop",
"mdOpenHoleBottom",
"tvdOpenHoleTop",
"tvdOpenHoleBottom",
"totalFrictionPresLoss",
"totalPumpTime",
"maxPresTubing",
"maxPresCasing",
"maxPresAnnulus",
"maxFluidRateTubing",
"maxFluidRateCasing",
"maxFluidRateAnnulus",
"avgPresTubing",
"avgPresCasing",
"avgPresAnnulus",
"breakDownPres",
"averagePres",
"avgBaseFluidReturnRate",
"avgSlurryReturnRate",
"avgBottomholeRate",
"totalVolume",
"maxProppantConcSurface",
"maxProppantConcBottomhole",
"avgProppantConcSurface",
"avgProppantConcBottomhole",
"perfproppantConc",
"totalProppantMass",
"totalProppantUsage",
"percentProppantPumped",
"wellboreProppantMass",
"proppantName",
"formationProppantMass",
"perfBallCount",
"totalN2StdVolume",
"totalCO2Mass",
"fluidName",
"fractureGradient",
"finalFractureGradient",
"initialShutinPres",
"shutinPres",
"screenOutPres",
"hhpOrderedCO2",
"hhpOrderedFluid",
"hhpUsedCO2",
"hhpUsedFluid",
"perfBallSize",
"screenedOut",
"avgFractureWidth",
"avgConductivity",
"netPres",
"closurePres",
"closureDuration",
"flowPath",
"pdatSession",
"reservoirInterval",
"perforationInterval",
"avgBHStaticTemperature",
"avgBHTreatingTemperature",
"additive",
"extensionNameValue"
})
public class CsStimJobInterval {
@XmlElement(required = true)
protected String uidTreatmentInterval;
@XmlElement(required = true)
protected String name;
protected short number;
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar dTimStart;
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar dTimEnd;
protected String formationName;
protected MeasuredDepthCoord mdFormationTop;
protected MeasuredDepthCoord mdFormationBottom;
protected WellVerticalDepthCoord tvdFormationTop;
protected WellVerticalDepthCoord tvdFormationBottom;
protected String openHoleName;
protected LengthMeasure openHoleDiameter;
protected MeasuredDepthCoord mdOpenHoleTop;
protected MeasuredDepthCoord mdOpenHoleBottom;
protected WellVerticalDepthCoord tvdOpenHoleTop;
protected WellVerticalDepthCoord tvdOpenHoleBottom;
protected PressureMeasure totalFrictionPresLoss;
protected TimeMeasure totalPumpTime;
protected PressureMeasure maxPresTubing;
protected PressureMeasure maxPresCasing;
protected PressureMeasure maxPresAnnulus;
protected VolumeFlowRateMeasure maxFluidRateTubing;
protected VolumeFlowRateMeasure maxFluidRateCasing;
protected VolumeFlowRateMeasure maxFluidRateAnnulus;
protected PressureMeasure avgPresTubing;
protected PressureMeasure avgPresCasing;
protected PressureMeasure avgPresAnnulus;
protected PressureMeasure breakDownPres;
protected PressureMeasure averagePres;
protected VolumeFlowRateMeasure avgBaseFluidReturnRate;
protected VolumeFlowRateMeasure avgSlurryReturnRate;
protected VolumeFlowRateMeasure avgBottomholeRate;
protected VolumeMeasure totalVolume;
protected DensityMeasure maxProppantConcSurface;
protected DensityMeasure maxProppantConcBottomhole;
protected DensityMeasure avgProppantConcSurface;
protected DensityMeasure avgProppantConcBottomhole;
protected DensityMeasure perfproppantConc;
protected MassMeasure totalProppantMass;
protected List totalProppantUsage;
protected VolumePerVolumeMeasurePercent percentProppantPumped;
protected MassMeasure wellboreProppantMass;
protected List proppantName;
protected MassMeasure formationProppantMass;
protected Short perfBallCount;
protected StandardVolumeMeasure totalN2StdVolume;
protected MassMeasure totalCO2Mass;
protected List fluidName;
protected ForcePerVolumeMeasure fractureGradient;
protected ForcePerVolumeMeasure finalFractureGradient;
protected PressureMeasure initialShutinPres;
protected List shutinPres;
protected PressureMeasure screenOutPres;
protected PowerMeasure hhpOrderedCO2;
protected PowerMeasure hhpOrderedFluid;
protected PowerMeasure hhpUsedCO2;
protected PowerMeasure hhpUsedFluid;
protected LengthMeasure perfBallSize;
protected Boolean screenedOut;
protected LengthMeasure avgFractureWidth;
protected VelocityMeasure avgConductivity;
protected PressureMeasure netPres;
protected PressureMeasure closurePres;
protected TimeMeasure closureDuration;
protected List flowPath;
protected List pdatSession;
protected List reservoirInterval;
protected List perforationInterval;
protected ThermodynamicTemperatureMeasure avgBHStaticTemperature;
protected ThermodynamicTemperatureMeasure avgBHTreatingTemperature;
protected List additive;
protected List extensionNameValue;
@XmlAttribute(name = "uid")
protected String uid;
/**
* Gets the value of the uidTreatmentInterval property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUidTreatmentInterval() {
return uidTreatmentInterval;
}
/**
* Sets the value of the uidTreatmentInterval property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUidTreatmentInterval(String value) {
this.uidTreatmentInterval = value;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the number property.
*
*/
public short getNumber() {
return number;
}
/**
* Sets the value of the number property.
*
*/
public void setNumber(short value) {
this.number = value;
}
/**
* Gets the value of the dTimStart property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getDTimStart() {
return dTimStart;
}
/**
* Sets the value of the dTimStart property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setDTimStart(XMLGregorianCalendar value) {
this.dTimStart = value;
}
/**
* Gets the value of the dTimEnd property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getDTimEnd() {
return dTimEnd;
}
/**
* Sets the value of the dTimEnd property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setDTimEnd(XMLGregorianCalendar value) {
this.dTimEnd = value;
}
/**
* Gets the value of the formationName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFormationName() {
return formationName;
}
/**
* Sets the value of the formationName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFormationName(String value) {
this.formationName = value;
}
/**
* Gets the value of the mdFormationTop property.
*
* @return
* possible object is
* {@link MeasuredDepthCoord }
*
*/
public MeasuredDepthCoord getMdFormationTop() {
return mdFormationTop;
}
/**
* Sets the value of the mdFormationTop property.
*
* @param value
* allowed object is
* {@link MeasuredDepthCoord }
*
*/
public void setMdFormationTop(MeasuredDepthCoord value) {
this.mdFormationTop = value;
}
/**
* Gets the value of the mdFormationBottom property.
*
* @return
* possible object is
* {@link MeasuredDepthCoord }
*
*/
public MeasuredDepthCoord getMdFormationBottom() {
return mdFormationBottom;
}
/**
* Sets the value of the mdFormationBottom property.
*
* @param value
* allowed object is
* {@link MeasuredDepthCoord }
*
*/
public void setMdFormationBottom(MeasuredDepthCoord value) {
this.mdFormationBottom = value;
}
/**
* Gets the value of the tvdFormationTop property.
*
* @return
* possible object is
* {@link WellVerticalDepthCoord }
*
*/
public WellVerticalDepthCoord getTvdFormationTop() {
return tvdFormationTop;
}
/**
* Sets the value of the tvdFormationTop property.
*
* @param value
* allowed object is
* {@link WellVerticalDepthCoord }
*
*/
public void setTvdFormationTop(WellVerticalDepthCoord value) {
this.tvdFormationTop = value;
}
/**
* Gets the value of the tvdFormationBottom property.
*
* @return
* possible object is
* {@link WellVerticalDepthCoord }
*
*/
public WellVerticalDepthCoord getTvdFormationBottom() {
return tvdFormationBottom;
}
/**
* Sets the value of the tvdFormationBottom property.
*
* @param value
* allowed object is
* {@link WellVerticalDepthCoord }
*
*/
public void setTvdFormationBottom(WellVerticalDepthCoord value) {
this.tvdFormationBottom = value;
}
/**
* Gets the value of the openHoleName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOpenHoleName() {
return openHoleName;
}
/**
* Sets the value of the openHoleName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOpenHoleName(String value) {
this.openHoleName = value;
}
/**
* Gets the value of the openHoleDiameter property.
*
* @return
* possible object is
* {@link LengthMeasure }
*
*/
public LengthMeasure getOpenHoleDiameter() {
return openHoleDiameter;
}
/**
* Sets the value of the openHoleDiameter property.
*
* @param value
* allowed object is
* {@link LengthMeasure }
*
*/
public void setOpenHoleDiameter(LengthMeasure value) {
this.openHoleDiameter = value;
}
/**
* Gets the value of the mdOpenHoleTop property.
*
* @return
* possible object is
* {@link MeasuredDepthCoord }
*
*/
public MeasuredDepthCoord getMdOpenHoleTop() {
return mdOpenHoleTop;
}
/**
* Sets the value of the mdOpenHoleTop property.
*
* @param value
* allowed object is
* {@link MeasuredDepthCoord }
*
*/
public void setMdOpenHoleTop(MeasuredDepthCoord value) {
this.mdOpenHoleTop = value;
}
/**
* Gets the value of the mdOpenHoleBottom property.
*
* @return
* possible object is
* {@link MeasuredDepthCoord }
*
*/
public MeasuredDepthCoord getMdOpenHoleBottom() {
return mdOpenHoleBottom;
}
/**
* Sets the value of the mdOpenHoleBottom property.
*
* @param value
* allowed object is
* {@link MeasuredDepthCoord }
*
*/
public void setMdOpenHoleBottom(MeasuredDepthCoord value) {
this.mdOpenHoleBottom = value;
}
/**
* Gets the value of the tvdOpenHoleTop property.
*
* @return
* possible object is
* {@link WellVerticalDepthCoord }
*
*/
public WellVerticalDepthCoord getTvdOpenHoleTop() {
return tvdOpenHoleTop;
}
/**
* Sets the value of the tvdOpenHoleTop property.
*
* @param value
* allowed object is
* {@link WellVerticalDepthCoord }
*
*/
public void setTvdOpenHoleTop(WellVerticalDepthCoord value) {
this.tvdOpenHoleTop = value;
}
/**
* Gets the value of the tvdOpenHoleBottom property.
*
* @return
* possible object is
* {@link WellVerticalDepthCoord }
*
*/
public WellVerticalDepthCoord getTvdOpenHoleBottom() {
return tvdOpenHoleBottom;
}
/**
* Sets the value of the tvdOpenHoleBottom property.
*
* @param value
* allowed object is
* {@link WellVerticalDepthCoord }
*
*/
public void setTvdOpenHoleBottom(WellVerticalDepthCoord value) {
this.tvdOpenHoleBottom = value;
}
/**
* Gets the value of the totalFrictionPresLoss property.
*
* @return
* possible object is
* {@link PressureMeasure }
*
*/
public PressureMeasure getTotalFrictionPresLoss() {
return totalFrictionPresLoss;
}
/**
* Sets the value of the totalFrictionPresLoss property.
*
* @param value
* allowed object is
* {@link PressureMeasure }
*
*/
public void setTotalFrictionPresLoss(PressureMeasure value) {
this.totalFrictionPresLoss = value;
}
/**
* Gets the value of the totalPumpTime property.
*
* @return
* possible object is
* {@link TimeMeasure }
*
*/
public TimeMeasure getTotalPumpTime() {
return totalPumpTime;
}
/**
* Sets the value of the totalPumpTime property.
*
* @param value
* allowed object is
* {@link TimeMeasure }
*
*/
public void setTotalPumpTime(TimeMeasure value) {
this.totalPumpTime = value;
}
/**
* Gets the value of the maxPresTubing property.
*
* @return
* possible object is
* {@link PressureMeasure }
*
*/
public PressureMeasure getMaxPresTubing() {
return maxPresTubing;
}
/**
* Sets the value of the maxPresTubing property.
*
* @param value
* allowed object is
* {@link PressureMeasure }
*
*/
public void setMaxPresTubing(PressureMeasure value) {
this.maxPresTubing = value;
}
/**
* Gets the value of the maxPresCasing property.
*
* @return
* possible object is
* {@link PressureMeasure }
*
*/
public PressureMeasure getMaxPresCasing() {
return maxPresCasing;
}
/**
* Sets the value of the maxPresCasing property.
*
* @param value
* allowed object is
* {@link PressureMeasure }
*
*/
public void setMaxPresCasing(PressureMeasure value) {
this.maxPresCasing = value;
}
/**
* Gets the value of the maxPresAnnulus property.
*
* @return
* possible object is
* {@link PressureMeasure }
*
*/
public PressureMeasure getMaxPresAnnulus() {
return maxPresAnnulus;
}
/**
* Sets the value of the maxPresAnnulus property.
*
* @param value
* allowed object is
* {@link PressureMeasure }
*
*/
public void setMaxPresAnnulus(PressureMeasure value) {
this.maxPresAnnulus = value;
}
/**
* Gets the value of the maxFluidRateTubing property.
*
* @return
* possible object is
* {@link VolumeFlowRateMeasure }
*
*/
public VolumeFlowRateMeasure getMaxFluidRateTubing() {
return maxFluidRateTubing;
}
/**
* Sets the value of the maxFluidRateTubing property.
*
* @param value
* allowed object is
* {@link VolumeFlowRateMeasure }
*
*/
public void setMaxFluidRateTubing(VolumeFlowRateMeasure value) {
this.maxFluidRateTubing = value;
}
/**
* Gets the value of the maxFluidRateCasing property.
*
* @return
* possible object is
* {@link VolumeFlowRateMeasure }
*
*/
public VolumeFlowRateMeasure getMaxFluidRateCasing() {
return maxFluidRateCasing;
}
/**
* Sets the value of the maxFluidRateCasing property.
*
* @param value
* allowed object is
* {@link VolumeFlowRateMeasure }
*
*/
public void setMaxFluidRateCasing(VolumeFlowRateMeasure value) {
this.maxFluidRateCasing = value;
}
/**
* Gets the value of the maxFluidRateAnnulus property.
*
* @return
* possible object is
* {@link VolumeFlowRateMeasure }
*
*/
public VolumeFlowRateMeasure getMaxFluidRateAnnulus() {
return maxFluidRateAnnulus;
}
/**
* Sets the value of the maxFluidRateAnnulus property.
*
* @param value
* allowed object is
* {@link VolumeFlowRateMeasure }
*
*/
public void setMaxFluidRateAnnulus(VolumeFlowRateMeasure value) {
this.maxFluidRateAnnulus = value;
}
/**
* Gets the value of the avgPresTubing property.
*
* @return
* possible object is
* {@link PressureMeasure }
*
*/
public PressureMeasure getAvgPresTubing() {
return avgPresTubing;
}
/**
* Sets the value of the avgPresTubing property.
*
* @param value
* allowed object is
* {@link PressureMeasure }
*
*/
public void setAvgPresTubing(PressureMeasure value) {
this.avgPresTubing = value;
}
/**
* Gets the value of the avgPresCasing property.
*
* @return
* possible object is
* {@link PressureMeasure }
*
*/
public PressureMeasure getAvgPresCasing() {
return avgPresCasing;
}
/**
* Sets the value of the avgPresCasing property.
*
* @param value
* allowed object is
* {@link PressureMeasure }
*
*/
public void setAvgPresCasing(PressureMeasure value) {
this.avgPresCasing = value;
}
/**
* Gets the value of the avgPresAnnulus property.
*
* @return
* possible object is
* {@link PressureMeasure }
*
*/
public PressureMeasure getAvgPresAnnulus() {
return avgPresAnnulus;
}
/**
* Sets the value of the avgPresAnnulus property.
*
* @param value
* allowed object is
* {@link PressureMeasure }
*
*/
public void setAvgPresAnnulus(PressureMeasure value) {
this.avgPresAnnulus = value;
}
/**
* Gets the value of the breakDownPres property.
*
* @return
* possible object is
* {@link PressureMeasure }
*
*/
public PressureMeasure getBreakDownPres() {
return breakDownPres;
}
/**
* Sets the value of the breakDownPres property.
*
* @param value
* allowed object is
* {@link PressureMeasure }
*
*/
public void setBreakDownPres(PressureMeasure value) {
this.breakDownPres = value;
}
/**
* Gets the value of the averagePres property.
*
* @return
* possible object is
* {@link PressureMeasure }
*
*/
public PressureMeasure getAveragePres() {
return averagePres;
}
/**
* Sets the value of the averagePres property.
*
* @param value
* allowed object is
* {@link PressureMeasure }
*
*/
public void setAveragePres(PressureMeasure value) {
this.averagePres = value;
}
/**
* Gets the value of the avgBaseFluidReturnRate property.
*
* @return
* possible object is
* {@link VolumeFlowRateMeasure }
*
*/
public VolumeFlowRateMeasure getAvgBaseFluidReturnRate() {
return avgBaseFluidReturnRate;
}
/**
* Sets the value of the avgBaseFluidReturnRate property.
*
* @param value
* allowed object is
* {@link VolumeFlowRateMeasure }
*
*/
public void setAvgBaseFluidReturnRate(VolumeFlowRateMeasure value) {
this.avgBaseFluidReturnRate = value;
}
/**
* Gets the value of the avgSlurryReturnRate property.
*
* @return
* possible object is
* {@link VolumeFlowRateMeasure }
*
*/
public VolumeFlowRateMeasure getAvgSlurryReturnRate() {
return avgSlurryReturnRate;
}
/**
* Sets the value of the avgSlurryReturnRate property.
*
* @param value
* allowed object is
* {@link VolumeFlowRateMeasure }
*
*/
public void setAvgSlurryReturnRate(VolumeFlowRateMeasure value) {
this.avgSlurryReturnRate = value;
}
/**
* Gets the value of the avgBottomholeRate property.
*
* @return
* possible object is
* {@link VolumeFlowRateMeasure }
*
*/
public VolumeFlowRateMeasure getAvgBottomholeRate() {
return avgBottomholeRate;
}
/**
* Sets the value of the avgBottomholeRate property.
*
* @param value
* allowed object is
* {@link VolumeFlowRateMeasure }
*
*/
public void setAvgBottomholeRate(VolumeFlowRateMeasure value) {
this.avgBottomholeRate = value;
}
/**
* Gets the value of the totalVolume property.
*
* @return
* possible object is
* {@link VolumeMeasure }
*
*/
public VolumeMeasure getTotalVolume() {
return totalVolume;
}
/**
* Sets the value of the totalVolume property.
*
* @param value
* allowed object is
* {@link VolumeMeasure }
*
*/
public void setTotalVolume(VolumeMeasure value) {
this.totalVolume = value;
}
/**
* Gets the value of the maxProppantConcSurface property.
*
* @return
* possible object is
* {@link DensityMeasure }
*
*/
public DensityMeasure getMaxProppantConcSurface() {
return maxProppantConcSurface;
}
/**
* Sets the value of the maxProppantConcSurface property.
*
* @param value
* allowed object is
* {@link DensityMeasure }
*
*/
public void setMaxProppantConcSurface(DensityMeasure value) {
this.maxProppantConcSurface = value;
}
/**
* Gets the value of the maxProppantConcBottomhole property.
*
* @return
* possible object is
* {@link DensityMeasure }
*
*/
public DensityMeasure getMaxProppantConcBottomhole() {
return maxProppantConcBottomhole;
}
/**
* Sets the value of the maxProppantConcBottomhole property.
*
* @param value
* allowed object is
* {@link DensityMeasure }
*
*/
public void setMaxProppantConcBottomhole(DensityMeasure value) {
this.maxProppantConcBottomhole = value;
}
/**
* Gets the value of the avgProppantConcSurface property.
*
* @return
* possible object is
* {@link DensityMeasure }
*
*/
public DensityMeasure getAvgProppantConcSurface() {
return avgProppantConcSurface;
}
/**
* Sets the value of the avgProppantConcSurface property.
*
* @param value
* allowed object is
* {@link DensityMeasure }
*
*/
public void setAvgProppantConcSurface(DensityMeasure value) {
this.avgProppantConcSurface = value;
}
/**
* Gets the value of the avgProppantConcBottomhole property.
*
* @return
* possible object is
* {@link DensityMeasure }
*
*/
public DensityMeasure getAvgProppantConcBottomhole() {
return avgProppantConcBottomhole;
}
/**
* Sets the value of the avgProppantConcBottomhole property.
*
* @param value
* allowed object is
* {@link DensityMeasure }
*
*/
public void setAvgProppantConcBottomhole(DensityMeasure value) {
this.avgProppantConcBottomhole = value;
}
/**
* Gets the value of the perfproppantConc property.
*
* @return
* possible object is
* {@link DensityMeasure }
*
*/
public DensityMeasure getPerfproppantConc() {
return perfproppantConc;
}
/**
* Sets the value of the perfproppantConc property.
*
* @param value
* allowed object is
* {@link DensityMeasure }
*
*/
public void setPerfproppantConc(DensityMeasure value) {
this.perfproppantConc = value;
}
/**
* Gets the value of the totalProppantMass property.
*
* @return
* possible object is
* {@link MassMeasure }
*
*/
public MassMeasure getTotalProppantMass() {
return totalProppantMass;
}
/**
* Sets the value of the totalProppantMass property.
*
* @param value
* allowed object is
* {@link MassMeasure }
*
*/
public void setTotalProppantMass(MassMeasure value) {
this.totalProppantMass = value;
}
/**
* Gets the value of the totalProppantUsage property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the totalProppantUsage property.
*
*
* For example, to add a new item, do as follows:
* {@code
* getTotalProppantUsage().add(newItem);
* }
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CsStimProppantUsage }
*
*
*/
public List getTotalProppantUsage() {
if (totalProppantUsage == null) {
totalProppantUsage = new ArrayList();
}
return this.totalProppantUsage;
}
/**
* Gets the value of the percentProppantPumped property.
*
* @return
* possible object is
* {@link VolumePerVolumeMeasurePercent }
*
*/
public VolumePerVolumeMeasurePercent getPercentProppantPumped() {
return percentProppantPumped;
}
/**
* Sets the value of the percentProppantPumped property.
*
* @param value
* allowed object is
* {@link VolumePerVolumeMeasurePercent }
*
*/
public void setPercentProppantPumped(VolumePerVolumeMeasurePercent value) {
this.percentProppantPumped = value;
}
/**
* Gets the value of the wellboreProppantMass property.
*
* @return
* possible object is
* {@link MassMeasure }
*
*/
public MassMeasure getWellboreProppantMass() {
return wellboreProppantMass;
}
/**
* Sets the value of the wellboreProppantMass property.
*
* @param value
* allowed object is
* {@link MassMeasure }
*
*/
public void setWellboreProppantMass(MassMeasure value) {
this.wellboreProppantMass = value;
}
/**
* Gets the value of the proppantName property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the proppantName property.
*
*
* For example, to add a new item, do as follows:
* {@code
* getProppantName().add(newItem);
* }
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getProppantName() {
if (proppantName == null) {
proppantName = new ArrayList();
}
return this.proppantName;
}
/**
* Gets the value of the formationProppantMass property.
*
* @return
* possible object is
* {@link MassMeasure }
*
*/
public MassMeasure getFormationProppantMass() {
return formationProppantMass;
}
/**
* Sets the value of the formationProppantMass property.
*
* @param value
* allowed object is
* {@link MassMeasure }
*
*/
public void setFormationProppantMass(MassMeasure value) {
this.formationProppantMass = value;
}
/**
* Gets the value of the perfBallCount property.
*
* @return
* possible object is
* {@link Short }
*
*/
public Short getPerfBallCount() {
return perfBallCount;
}
/**
* Sets the value of the perfBallCount property.
*
* @param value
* allowed object is
* {@link Short }
*
*/
public void setPerfBallCount(Short value) {
this.perfBallCount = value;
}
/**
* Gets the value of the totalN2StdVolume property.
*
* @return
* possible object is
* {@link StandardVolumeMeasure }
*
*/
public StandardVolumeMeasure getTotalN2StdVolume() {
return totalN2StdVolume;
}
/**
* Sets the value of the totalN2StdVolume property.
*
* @param value
* allowed object is
* {@link StandardVolumeMeasure }
*
*/
public void setTotalN2StdVolume(StandardVolumeMeasure value) {
this.totalN2StdVolume = value;
}
/**
* Gets the value of the totalCO2Mass property.
*
* @return
* possible object is
* {@link MassMeasure }
*
*/
public MassMeasure getTotalCO2Mass() {
return totalCO2Mass;
}
/**
* Sets the value of the totalCO2Mass property.
*
* @param value
* allowed object is
* {@link MassMeasure }
*
*/
public void setTotalCO2Mass(MassMeasure value) {
this.totalCO2Mass = value;
}
/**
* Gets the value of the fluidName property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the fluidName property.
*
*
* For example, to add a new item, do as follows:
* {@code
* getFluidName().add(newItem);
* }
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getFluidName() {
if (fluidName == null) {
fluidName = new ArrayList();
}
return this.fluidName;
}
/**
* Gets the value of the fractureGradient property.
*
* @return
* possible object is
* {@link ForcePerVolumeMeasure }
*
*/
public ForcePerVolumeMeasure getFractureGradient() {
return fractureGradient;
}
/**
* Sets the value of the fractureGradient property.
*
* @param value
* allowed object is
* {@link ForcePerVolumeMeasure }
*
*/
public void setFractureGradient(ForcePerVolumeMeasure value) {
this.fractureGradient = value;
}
/**
* Gets the value of the finalFractureGradient property.
*
* @return
* possible object is
* {@link ForcePerVolumeMeasure }
*
*/
public ForcePerVolumeMeasure getFinalFractureGradient() {
return finalFractureGradient;
}
/**
* Sets the value of the finalFractureGradient property.
*
* @param value
* allowed object is
* {@link ForcePerVolumeMeasure }
*
*/
public void setFinalFractureGradient(ForcePerVolumeMeasure value) {
this.finalFractureGradient = value;
}
/**
* Gets the value of the initialShutinPres property.
*
* @return
* possible object is
* {@link PressureMeasure }
*
*/
public PressureMeasure getInitialShutinPres() {
return initialShutinPres;
}
/**
* Sets the value of the initialShutinPres property.
*
* @param value
* allowed object is
* {@link PressureMeasure }
*
*/
public void setInitialShutinPres(PressureMeasure value) {
this.initialShutinPres = value;
}
/**
* Gets the value of the shutinPres property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the shutinPres property.
*
*
* For example, to add a new item, do as follows:
* {@code
* getShutinPres().add(newItem);
* }
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CsStimShutInPressure }
*
*
*/
public List getShutinPres() {
if (shutinPres == null) {
shutinPres = new ArrayList();
}
return this.shutinPres;
}
/**
* Gets the value of the screenOutPres property.
*
* @return
* possible object is
* {@link PressureMeasure }
*
*/
public PressureMeasure getScreenOutPres() {
return screenOutPres;
}
/**
* Sets the value of the screenOutPres property.
*
* @param value
* allowed object is
* {@link PressureMeasure }
*
*/
public void setScreenOutPres(PressureMeasure value) {
this.screenOutPres = value;
}
/**
* Gets the value of the hhpOrderedCO2 property.
*
* @return
* possible object is
* {@link PowerMeasure }
*
*/
public PowerMeasure getHhpOrderedCO2() {
return hhpOrderedCO2;
}
/**
* Sets the value of the hhpOrderedCO2 property.
*
* @param value
* allowed object is
* {@link PowerMeasure }
*
*/
public void setHhpOrderedCO2(PowerMeasure value) {
this.hhpOrderedCO2 = value;
}
/**
* Gets the value of the hhpOrderedFluid property.
*
* @return
* possible object is
* {@link PowerMeasure }
*
*/
public PowerMeasure getHhpOrderedFluid() {
return hhpOrderedFluid;
}
/**
* Sets the value of the hhpOrderedFluid property.
*
* @param value
* allowed object is
* {@link PowerMeasure }
*
*/
public void setHhpOrderedFluid(PowerMeasure value) {
this.hhpOrderedFluid = value;
}
/**
* Gets the value of the hhpUsedCO2 property.
*
* @return
* possible object is
* {@link PowerMeasure }
*
*/
public PowerMeasure getHhpUsedCO2() {
return hhpUsedCO2;
}
/**
* Sets the value of the hhpUsedCO2 property.
*
* @param value
* allowed object is
* {@link PowerMeasure }
*
*/
public void setHhpUsedCO2(PowerMeasure value) {
this.hhpUsedCO2 = value;
}
/**
* Gets the value of the hhpUsedFluid property.
*
* @return
* possible object is
* {@link PowerMeasure }
*
*/
public PowerMeasure getHhpUsedFluid() {
return hhpUsedFluid;
}
/**
* Sets the value of the hhpUsedFluid property.
*
* @param value
* allowed object is
* {@link PowerMeasure }
*
*/
public void setHhpUsedFluid(PowerMeasure value) {
this.hhpUsedFluid = value;
}
/**
* Gets the value of the perfBallSize property.
*
* @return
* possible object is
* {@link LengthMeasure }
*
*/
public LengthMeasure getPerfBallSize() {
return perfBallSize;
}
/**
* Sets the value of the perfBallSize property.
*
* @param value
* allowed object is
* {@link LengthMeasure }
*
*/
public void setPerfBallSize(LengthMeasure value) {
this.perfBallSize = value;
}
/**
* Gets the value of the screenedOut property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isScreenedOut() {
return screenedOut;
}
/**
* Sets the value of the screenedOut property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setScreenedOut(Boolean value) {
this.screenedOut = value;
}
/**
* Gets the value of the avgFractureWidth property.
*
* @return
* possible object is
* {@link LengthMeasure }
*
*/
public LengthMeasure getAvgFractureWidth() {
return avgFractureWidth;
}
/**
* Sets the value of the avgFractureWidth property.
*
* @param value
* allowed object is
* {@link LengthMeasure }
*
*/
public void setAvgFractureWidth(LengthMeasure value) {
this.avgFractureWidth = value;
}
/**
* Gets the value of the avgConductivity property.
*
* @return
* possible object is
* {@link VelocityMeasure }
*
*/
public VelocityMeasure getAvgConductivity() {
return avgConductivity;
}
/**
* Sets the value of the avgConductivity property.
*
* @param value
* allowed object is
* {@link VelocityMeasure }
*
*/
public void setAvgConductivity(VelocityMeasure value) {
this.avgConductivity = value;
}
/**
* Gets the value of the netPres property.
*
* @return
* possible object is
* {@link PressureMeasure }
*
*/
public PressureMeasure getNetPres() {
return netPres;
}
/**
* Sets the value of the netPres property.
*
* @param value
* allowed object is
* {@link PressureMeasure }
*
*/
public void setNetPres(PressureMeasure value) {
this.netPres = value;
}
/**
* Gets the value of the closurePres property.
*
* @return
* possible object is
* {@link PressureMeasure }
*
*/
public PressureMeasure getClosurePres() {
return closurePres;
}
/**
* Sets the value of the closurePres property.
*
* @param value
* allowed object is
* {@link PressureMeasure }
*
*/
public void setClosurePres(PressureMeasure value) {
this.closurePres = value;
}
/**
* Gets the value of the closureDuration property.
*
* @return
* possible object is
* {@link TimeMeasure }
*
*/
public TimeMeasure getClosureDuration() {
return closureDuration;
}
/**
* Sets the value of the closureDuration property.
*
* @param value
* allowed object is
* {@link TimeMeasure }
*
*/
public void setClosureDuration(TimeMeasure value) {
this.closureDuration = value;
}
/**
* Gets the value of the flowPath property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the flowPath property.
*
*
* For example, to add a new item, do as follows:
* {@code
* getFlowPath().add(newItem);
* }
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CsStimFlowPath }
*
*
*/
public List getFlowPath() {
if (flowPath == null) {
flowPath = new ArrayList();
}
return this.flowPath;
}
/**
* Gets the value of the pdatSession property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the pdatSession property.
*
*
* For example, to add a new item, do as follows:
* {@code
* getPdatSession().add(newItem);
* }
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CsStimPdatSession }
*
*
*/
public List getPdatSession() {
if (pdatSession == null) {
pdatSession = new ArrayList();
}
return this.pdatSession;
}
/**
* Gets the value of the reservoirInterval property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the reservoirInterval property.
*
*
* For example, to add a new item, do as follows:
* {@code
* getReservoirInterval().add(newItem);
* }
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CsStimReservoirInterval }
*
*
*/
public List getReservoirInterval() {
if (reservoirInterval == null) {
reservoirInterval = new ArrayList();
}
return this.reservoirInterval;
}
/**
* Gets the value of the perforationInterval property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the perforationInterval property.
*
*
* For example, to add a new item, do as follows:
* {@code
* getPerforationInterval().add(newItem);
* }
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CsStimPerforationSet }
*
*
*/
public List getPerforationInterval() {
if (perforationInterval == null) {
perforationInterval = new ArrayList();
}
return this.perforationInterval;
}
/**
* Gets the value of the avgBHStaticTemperature property.
*
* @return
* possible object is
* {@link ThermodynamicTemperatureMeasure }
*
*/
public ThermodynamicTemperatureMeasure getAvgBHStaticTemperature() {
return avgBHStaticTemperature;
}
/**
* Sets the value of the avgBHStaticTemperature property.
*
* @param value
* allowed object is
* {@link ThermodynamicTemperatureMeasure }
*
*/
public void setAvgBHStaticTemperature(ThermodynamicTemperatureMeasure value) {
this.avgBHStaticTemperature = value;
}
/**
* Gets the value of the avgBHTreatingTemperature property.
*
* @return
* possible object is
* {@link ThermodynamicTemperatureMeasure }
*
*/
public ThermodynamicTemperatureMeasure getAvgBHTreatingTemperature() {
return avgBHTreatingTemperature;
}
/**
* Sets the value of the avgBHTreatingTemperature property.
*
* @param value
* allowed object is
* {@link ThermodynamicTemperatureMeasure }
*
*/
public void setAvgBHTreatingTemperature(ThermodynamicTemperatureMeasure value) {
this.avgBHTreatingTemperature = value;
}
/**
* Gets the value of the additive property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the additive property.
*
*
* For example, to add a new item, do as follows:
* {@code
* getAdditive().add(newItem);
* }
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CsStimAdditive }
*
*
*/
public List getAdditive() {
if (additive == null) {
additive = new ArrayList();
}
return this.additive;
}
/**
* Gets the value of the extensionNameValue property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the extensionNameValue property.
*
*
* For example, to add a new item, do as follows:
* {@code
* getExtensionNameValue().add(newItem);
* }
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CsExtensionNameValue }
*
*
*/
public List getExtensionNameValue() {
if (extensionNameValue == null) {
extensionNameValue = new ArrayList();
}
return this.extensionNameValue;
}
/**
* Gets the value of the uid property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUid() {
return uid;
}
/**
* Sets the value of the uid property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUid(String value) {
this.uid = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy