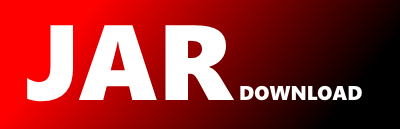
com.haventec.nativeux.adapter.java.sdk.impl.service.OidcLpIntrospectService Maven / Gradle / Ivy
Show all versions of native-ux-adapter-java-sdk Show documentation
package com.haventec.nativeux.adapter.java.sdk.impl.service;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.haventec.nativeux.adapter.java.sdk.api.exception.HaventecException;
import com.haventec.nativeux.adapter.java.sdk.api.exception.NativeUxAdapterSdkError;
import com.haventec.nativeux.adapter.java.sdk.api.models.DeleteUserRequest;
import com.haventec.nativeux.adapter.java.sdk.api.models.GenericResponse;
import com.haventec.nativeux.adapter.java.sdk.api.models.ResponseStatus;
import com.haventec.nativeux.adapter.java.sdk.api.models.UpdateUserRequest;
import com.haventec.nativeux.adapter.java.sdk.impl.model.OidcParams;
import com.haventec.nativeux.adapter.java.sdk.impl.utils.Constants;
import com.haventec.nativeux.adapter.java.sdk.impl.utils.HttpDeleteWithRequestBody;
import com.haventec.nativeux.adapter.java.sdk.impl.utils.HttpUtils;
import lombok.extern.slf4j.Slf4j;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPatch;
import org.apache.http.client.protocol.HttpClientContext;
import org.apache.http.impl.client.CloseableHttpClient;
import java.io.IOException;
import java.net.URISyntaxException;
/**
* Service class represents APIs request to Haventec connect to introspect external Identity provider and update authenticate user by an externally authenticated user e.g. a CRM user
*/
@Slf4j
public class OidcLpIntrospectService {
private static final String LP_API_URI = "/lp/api/oidc-landing-page/v1-0";
private static final String UPDATE_USER = "/iam/user?username={username}";
private static final String DELETE_USER = "/iam/user/{username}";
private final ObjectMapper objectMapper = new ObjectMapper();
private final HttpUtils httpUtils = HttpUtils.builder()
.objectMapper(objectMapper).build();
/**
* Update user details e.g. email or mobile using of authenticate user by externally authenticated user e.g. a CRM user
*
* @param request {@link UpdateUserRequest} Request contains
* clientId : client id of the application: ff187601-4027-4536-913e-7a65b2b65d0d
*
email : Email to be updated e.g. [email protected]
*
mobileNumber : Mobile number to be updated e.g. 0343434343
*
requestorUsername : username of the externally authenticated as per IAM e.g. crm-user
*
requestorToken : access token of the externally authenticated user e.g. eyJhbGciOiJSUzI1NiIsInR5cCIgOiAiSldUIiwia2lkIiA6ICJLSjNKTDUtaGFRUHdKWER4djB5M1N0MWdLQ3pMNWZ4a1lNVHVoeDZpa284In0.eyJleHAiOjE2MjkxNTE4MjcsImlhdCI6MTYyOTE1MTUyNywianRpIjoiYzcyZTNiODktNGI4NC00MjdiLWI0ZWUtNmYwYTVjNDk3NDA1IiwiaXNzIjoiaHR0cDovL2xvY2FsaG9zdDo4MTgwL2F1dGgvcmVhbG1zL2NybS1yZWFsbSIsImF1ZCI6ImFjY291bnQiLCJzdWIiOiIyMWY3ZmIxOC04NDhiLTRhZDctOTZmZS1lMGNiYjFiMDA3YTAiLCJ0eXAiOiJCZWFyZXIiLCJhenAiOiJjcm0tY2xpZW50Iiwic2Vzc2lvbl9zdGF0ZSI6IjcwNTE3ZWM0LTZhY2EtNGQzNy05ZGVhLWFhODk5MDc0NzlhZiIsImFjciI6IjEiLCJyZWFsbV9hY2Nlc3MiOnsicm9sZXMiOlsib2ZmbGluZV9hY2Nlc3MiLCJ1bWFfYXV0aG9yaXphdGlvbiJdfSwicmVzb3VyY2VfYWNjZXNzIjp7ImFjY291bnQiOnsicm9sZXMiOlsibWFuYWdlLWFjY291bnQiLCJtYW5hZ2UtYWNjb3VudC1saW5rcyIsInZpZXctcHJvZmlsZSJdfX0sInNjb3BlIjoicHJvZmlsZSBlbWFpbCIsImVtYWlsX3ZlcmlmaWVkIjpmYWxzZSwibmFtZSI6IlNhbmRlZXAgS2FtYXRoIiwicHJlZmVycmVkX3VzZXJuYW1lIjoiY3JtLXVzZXIiLCJnaXZlbl9uYW1lIjoiU2FuZGVlcCIsImZhbWlseV9uYW1lIjoiS2FtYXRoIiwiZW1haWwiOiJzYW5kZWVwLmthbWF0aEBoYXZlbnRlYy5jb20ifQ.WUXsByC3eJrCqgydWCEA-1Tj2XOKNgxHjEn5vlkAKjCnQ6m74aNdIPsU9Dl0UEhhoS_KlNbUSExLM7cfvrVHJ3zZe0YWilM33bkKw0j0dOXitQ-t5QBz9-re8aeSC_JHqBKKdHGbeSHIZ6ujYNFK1RgQ5UFqhlCKCNUWWVAu7N9VIqEmC-6UGVvqFF44r7Y-ch4An8B9Yt5kXFxutEpDr6Z1PrXhlfZDiOLNGnU2Xz_4JpJwJ70-iF_9l7p_rppikDyvBBpAUdeiOzienDoYJ_enZUYtqPc5BNQTSimJcoPEHye8pAnJSg-yVbVOFQzUu6SuCSqV5PO0xaQ-1UayIA
*
otpUuid : uuid used in generating the otp e.g. ff187601-4027-4536-913e-7a65b2b65d0d
*
purpose : the purpose used in generating the otp e.g. Update username
*
otpValue : the value of the otp e.g. 123456
*
newUsername : the new username to update in the system e.g. new_user
* @param haventecUsername Haventec authenticate username e.g. 2cfbef2d-7e2f-448c-a404-000cd103e1be
* @param params Oidc parameters obtained from IAM e.g. Haventec Connect host details
* @param httpClient http client object to call APIs request in Haventec Connect
* @param context HttpContext represents execution state of an HTTP process
* @return generic response with status success or failure
* @throws HaventecException Exception is thrown for user case e.g. unable to connect to Haventec Connect
* @throws IOException IO exception e.g. unable to execute httpClient request
* @throws URISyntaxException URI exception e.g. if the url is not build properly
*/
public GenericResponse updateUser(UpdateUserRequest request, String haventecUsername, OidcParams params, CloseableHttpClient httpClient, HttpClientContext context) throws IOException, HaventecException, URISyntaxException {
String methodName = "updateUser";
String urlPath = String.format("%s%s", LP_API_URI, UPDATE_USER.replace("{username}", haventecUsername));
HttpPatch httpPatch = httpUtils.createHttpPatch(request, urlPath, params, null);
try (CloseableHttpResponse execute = httpClient.execute(httpPatch, context)) {
JsonNode result = httpUtils.getObjectMapper().readTree(execute.getEntity().getContent());
if (execute.getStatusLine().getStatusCode() != 200) {
log.error("{} - Unsuccessful response from Oidc LP while updating user for host: {} ", methodName, params.getLandingPageHost());
throw new HaventecException(result.get(Constants.RESPONSE_STATUS).get(Constants.CODE).asText(), result.get(Constants.RESPONSE_STATUS).get(Constants.MESSAGE).asText());
}
JsonNode responseStatusJson = result.get(Constants.RESPONSE_STATUS);
ResponseStatus responseStatus = new ResponseStatus.ResponseStatusBuilder(responseStatusJson.get(Constants.STATUS).asText())
.code(responseStatusJson.get(Constants.CODE) != null ? responseStatusJson.get(Constants.CODE).asText() : null)
.message(responseStatusJson.get(Constants.MESSAGE) != null ? responseStatusJson.get(Constants.MESSAGE).asText() : null).build();
return GenericResponse.builder()
.responseStatus(responseStatus).build();
} catch (JsonParseException e) {
log.error("{} - Unable to communicate with Oidc LP while updating user in oidc landing page at host={}", methodName, params.getLandingPageHost());
throw new HaventecException(NativeUxAdapterSdkError.UNABLE_TO_COMMUNICATE_WITH_OIDC_LP, e);
}
}
/**
* Delete authenticate user by externally authenticated user e.g. a CRM user
*
* @param request {@link DeleteUserRequest} Request contains
*
clientId : client id of the application: ff187601-4027-4536-913e-7a65b2b65d0d
*
requestorUsername : username of the externally authenticated as per IAM e.g. crm-user
*
requestorToken : access token of the externally authenticated user e.g. eyJhbGciOiJSUzI1NiIsInR5cCIgOiAiSldUIiwia2lkIiA6ICJLSjNKTDUtaGFRUHdKWER4djB5M1N0MWdLQ3pMNWZ4a1lNVHVoeDZpa284In0.eyJleHAiOjE2MjkxNTE4MjcsImlhdCI6MTYyOTE1MTUyNywianRpIjoiYzcyZTNiODktNGI4NC00MjdiLWI0ZWUtNmYwYTVjNDk3NDA1IiwiaXNzIjoiaHR0cDovL2xvY2FsaG9zdDo4MTgwL2F1dGgvcmVhbG1zL2NybS1yZWFsbSIsImF1ZCI6ImFjY291bnQiLCJzdWIiOiIyMWY3ZmIxOC04NDhiLTRhZDctOTZmZS1lMGNiYjFiMDA3YTAiLCJ0eXAiOiJCZWFyZXIiLCJhenAiOiJjcm0tY2xpZW50Iiwic2Vzc2lvbl9zdGF0ZSI6IjcwNTE3ZWM0LTZhY2EtNGQzNy05ZGVhLWFhODk5MDc0NzlhZiIsImFjciI6IjEiLCJyZWFsbV9hY2Nlc3MiOnsicm9sZXMiOlsib2ZmbGluZV9hY2Nlc3MiLCJ1bWFfYXV0aG9yaXphdGlvbiJdfSwicmVzb3VyY2VfYWNjZXNzIjp7ImFjY291bnQiOnsicm9sZXMiOlsibWFuYWdlLWFjY291bnQiLCJtYW5hZ2UtYWNjb3VudC1saW5rcyIsInZpZXctcHJvZmlsZSJdfX0sInNjb3BlIjoicHJvZmlsZSBlbWFpbCIsImVtYWlsX3ZlcmlmaWVkIjpmYWxzZSwibmFtZSI6IlNhbmRlZXAgS2FtYXRoIiwicHJlZmVycmVkX3VzZXJuYW1lIjoiY3JtLXVzZXIiLCJnaXZlbl9uYW1lIjoiU2FuZGVlcCIsImZhbWlseV9uYW1lIjoiS2FtYXRoIiwiZW1haWwiOiJzYW5kZWVwLmthbWF0aEBoYXZlbnRlYy5jb20ifQ.WUXsByC3eJrCqgydWCEA-1Tj2XOKNgxHjEn5vlkAKjCnQ6m74aNdIPsU9Dl0UEhhoS_KlNbUSExLM7cfvrVHJ3zZe0YWilM33bkKw0j0dOXitQ-t5QBz9-re8aeSC_JHqBKKdHGbeSHIZ6ujYNFK1RgQ5UFqhlCKCNUWWVAu7N9VIqEmC-6UGVvqFF44r7Y-ch4An8B9Yt5kXFxutEpDr6Z1PrXhlfZDiOLNGnU2Xz_4JpJwJ70-iF_9l7p_rppikDyvBBpAUdeiOzienDoYJ_enZUYtqPc5BNQTSimJcoPEHye8pAnJSg-yVbVOFQzUu6SuCSqV5PO0xaQ-1UayIA
* @param haventecUsername Haventec authenticate username e.g. 2cfbef2d-7e2f-448c-a404-000cd103e1be
* @param params Oidc parameters obtained from IAM e.g. Haventec Connect host details
* @param httpClient http client object to call APIs request in Haventec Connect
* @param context HttpContext represents execution state of an HTTP process
* @return generic response with status success or failure
* @throws HaventecException Exception is thrown for user case e.g. unable to connect to Haventec Connect
* @throws URISyntaxException URI exception e.g. if the url is not build properly
*/
public GenericResponse deleteUser(DeleteUserRequest request, String haventecUsername, OidcParams params, CloseableHttpClient httpClient, HttpClientContext context) throws URISyntaxException, HaventecException {
String methodName = "deleteUser";
String urlPath = String.format("%s%s", LP_API_URI, DELETE_USER.replace("{username}", haventecUsername));
HttpDeleteWithRequestBody httpDelete = httpUtils.createHttpDelete(request, urlPath, params, null);
try (CloseableHttpResponse execute = httpClient.execute(httpDelete, context)) {
JsonNode result = httpUtils.getObjectMapper().readTree(execute.getEntity().getContent());
if (execute.getStatusLine().getStatusCode() != 200) {
log.error("{} - Unsuccessful response from Oidc LP while deleting user for host: {} ", methodName, params.getLandingPageHost());
throw new HaventecException(result.get(Constants.RESPONSE_STATUS).get(Constants.CODE).asText(), result.get(Constants.RESPONSE_STATUS).get(Constants.MESSAGE).asText());
}
JsonNode responseStatusJson = result.get(Constants.RESPONSE_STATUS);
ResponseStatus responseStatus = new ResponseStatus.ResponseStatusBuilder(responseStatusJson.get(Constants.STATUS).asText())
.code(responseStatusJson.get(Constants.CODE) != null ? responseStatusJson.get(Constants.CODE).asText() : null)
.message(responseStatusJson.get(Constants.MESSAGE) != null ? responseStatusJson.get(Constants.MESSAGE).asText() : null).build();
return GenericResponse.builder()
.responseStatus(responseStatus).build();
} catch (IOException e) {
log.error("{} - Unable to communicate with Oidc LP while deleting user in oidc landing page at host={}", methodName, params.getLandingPageHost());
throw new HaventecException(NativeUxAdapterSdkError.UNABLE_TO_COMMUNICATE_WITH_OIDC_LP, e);
}
}
}