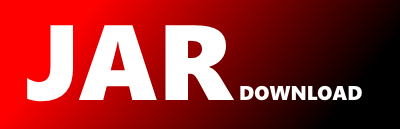
com.hazelcast.jet.elastic.ElasticClients Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hazelcast-jet-elasticsearch-7 Show documentation
Show all versions of hazelcast-jet-elasticsearch-7 Show documentation
Elasticsearch 7 support for Hazelcast Jet
/*
* Copyright 2023 Hazelcast Inc.
*
* Licensed under the Hazelcast Community License (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://hazelcast.com/hazelcast-community-license
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.hazelcast.jet.elastic;
import com.hazelcast.function.SupplierEx;
import org.apache.http.HttpHost;
import org.apache.http.auth.UsernamePasswordCredentials;
import org.apache.http.client.CredentialsProvider;
import org.apache.http.impl.client.BasicCredentialsProvider;
import org.elasticsearch.client.RestClient;
import org.elasticsearch.client.RestClientBuilder;
import javax.annotation.Nonnull;
import static org.apache.http.auth.AuthScope.ANY;
/**
* Collection of convenience factory methods for Elastic's {@link RestClientBuilder}
*
* Supposed to be used as a parameter to {@link ElasticSourceBuilder#clientFn(SupplierEx)}
* and {@link ElasticSinkBuilder#clientFn(SupplierEx)}, for example:
*
{@code
* builder.clientFn(() -> client());
* }
*/
public final class ElasticClients {
private static final int DEFAULT_PORT = 9200;
private ElasticClients() {
}
/**
* Create Elastic client for an instance running on localhost
* on default port (9200)
*/
@Nonnull
public static RestClientBuilder client() {
return client("localhost", DEFAULT_PORT);
}
/**
* Convenience method to create {@link RestClientBuilder} with given string, it must contain host, and optionally
* the scheme and a port.
*
* Valid examples:
* {@code elastic-host
* elastic-host:9200
* http://elastic-host:9200}
*
* @see HttpHost#create(String)
* @since Jet 4.3
*/
@Nonnull
public static RestClientBuilder client(@Nonnull String location) {
return RestClient.builder(HttpHost.create(location));
}
/**
* Convenience method to create {@link RestClientBuilder} with given
* hostname and port
*/
@Nonnull
public static RestClientBuilder client(@Nonnull String hostname, int port) {
return RestClient.builder(new HttpHost(hostname, port));
}
/**
* Convenience method to create {@link RestClientBuilder} with basic authentication
* and given hostname and port
*
* Usage:
*
{@code
* BatchSource source = elastic(() -> client("user", "password", "host", 9200));
* }
*/
@Nonnull
public static RestClientBuilder client(
@Nonnull String username,
@Nonnull String password,
@Nonnull String hostname,
int port
) {
return client(username, password, hostname, port, "http");
}
/**
* Convenience method to create {@link RestClientBuilder} with basic authentication
* and given hostname, port and scheme. Valid schemes are "http" and "https".
*
* Usage:
*
{@code
* BatchSource source = elastic(() -> client("user", "password", "host", 9200, "https"));
* }
*/
@Nonnull
public static RestClientBuilder client(
@Nonnull String username,
@Nonnull String password,
@Nonnull String hostname,
int port,
@Nonnull String scheme
) {
CredentialsProvider credentialsProvider = new BasicCredentialsProvider();
credentialsProvider.setCredentials(ANY, new UsernamePasswordCredentials(username, password));
return RestClient.builder(new HttpHost(hostname, port, scheme))
.setHttpClientConfigCallback(httpClientBuilder ->
httpClientBuilder.setDefaultCredentialsProvider(credentialsProvider)
);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy