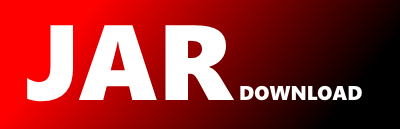
com.hazelcast.simulator.tests.helpers.HazelcastTestUtils Maven / Gradle / Ivy
/*
* Copyright (c) 2008-2017, Hazelcast, Inc. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.hazelcast.simulator.tests.helpers;
import com.hazelcast.core.HazelcastInstance;
import com.hazelcast.core.HazelcastInstanceAware;
import com.hazelcast.core.IExecutorService;
import com.hazelcast.core.IMap;
import com.hazelcast.core.Member;
import com.hazelcast.core.Partition;
import com.hazelcast.core.PartitionService;
import com.hazelcast.instance.BuildInfo;
import com.hazelcast.instance.BuildInfoProvider;
import com.hazelcast.instance.HazelcastInstanceImpl;
import com.hazelcast.instance.HazelcastInstanceProxy;
import com.hazelcast.instance.Node;
import com.hazelcast.logging.ILogger;
import com.hazelcast.logging.Logger;
import com.hazelcast.map.impl.MapService;
import com.hazelcast.map.impl.MapServiceContext;
import com.hazelcast.map.impl.proxy.MapProxyImpl;
import com.hazelcast.simulator.test.TestException;
import com.hazelcast.spi.OperationService;
import com.hazelcast.spi.impl.NodeEngineImpl;
import java.io.Serializable;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.Callable;
import java.util.concurrent.Future;
import static com.hazelcast.simulator.utils.CommonUtils.sleepSeconds;
import static com.hazelcast.simulator.utils.FormatUtils.NEW_LINE;
import static com.hazelcast.simulator.utils.Preconditions.checkNotNull;
import static com.hazelcast.simulator.utils.ReflectionUtils.getFieldValue;
import static com.hazelcast.simulator.utils.VersionUtils.isMinVersion;
import static java.lang.String.format;
import static org.junit.Assert.fail;
public final class HazelcastTestUtils {
private static final ILogger LOGGER = Logger.getLogger(HazelcastTestUtils.class);
private HazelcastTestUtils() {
}
public static RuntimeException rethrow(Throwable throwable) {
if (throwable instanceof RuntimeException) {
throw (RuntimeException) throwable;
} else {
throw new TestException(throwable);
}
}
public static String getPartitionDistributionInformation(HazelcastInstance hz) {
Map partitionCountMap = new HashMap();
int totalPartitions = 0;
for (Partition partition : hz.getPartitionService().getPartitions()) {
totalPartitions++;
Member member = partition.getOwner();
Integer count = partitionCountMap.get(member);
if (count == null) {
count = 0;
}
count++;
partitionCountMap.put(member, count);
}
StringBuilder sb = new StringBuilder();
sb.append("total partitions: ").append(totalPartitions).append(NEW_LINE);
for (Map.Entry entry : partitionCountMap.entrySet()) {
Member member = entry.getKey();
long count = entry.getValue();
double percentage = count * 100d / totalPartitions;
sb.append(member).append(" total: ").append(count)
.append(" percentage: ").append(percentage).append('%').append(NEW_LINE);
}
return sb.toString();
}
public static String getOperationCountInformation(HazelcastInstance hz) {
Map operationCountMap = getOperationCount(hz);
long totalOps = 0;
for (Long count : operationCountMap.values()) {
totalOps += count;
}
StringBuilder sb = new StringBuilder();
sb.append("total operations: ").append(totalOps).append(NEW_LINE);
for (Map.Entry entry : operationCountMap.entrySet()) {
Member member = entry.getKey();
long opsOnMember = entry.getValue();
double percentage = opsOnMember * 100d / totalOps;
sb.append(member)
.append(" operations: ").append(opsOnMember)
.append(" percentage: ").append(percentage).append('%').append(NEW_LINE);
}
return sb.toString();
}
public static Map getOperationCount(HazelcastInstance hz) {
IExecutorService executorService = hz.getExecutorService("operationCountExecutor");
Map> futures = new HashMap>();
for (Member member : hz.getCluster().getMembers()) {
Future future = executorService.submitToMember(new GetOperationCount(), member);
futures.put(member, future);
}
Map result = new HashMap();
for (Map.Entry> entry : futures.entrySet()) {
try {
Member member = entry.getKey();
Long value = entry.getValue().get();
if (value == null) {
value = 0L;
}
result.put(member, value);
} catch (Exception e) {
throw rethrow(e);
}
}
return result;
}
public static void logPartitionStatistics(ILogger log, String name, IMap
© 2015 - 2025 Weber Informatics LLC | Privacy Policy