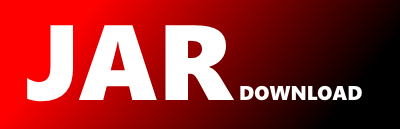
com.hazelcast.cache.impl.CacheDataSerializerHook Maven / Gradle / Ivy
/*
* Copyright (c) 2008-2016, Hazelcast, Inc. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.hazelcast.cache.impl;
import com.hazelcast.cache.HazelcastExpiryPolicy;
import com.hazelcast.cache.impl.operation.CacheBackupEntryProcessorOperation;
import com.hazelcast.cache.impl.operation.CacheClearBackupOperation;
import com.hazelcast.cache.impl.operation.CacheClearOperation;
import com.hazelcast.cache.impl.operation.CacheClearOperationFactory;
import com.hazelcast.cache.impl.operation.CacheContainsKeyOperation;
import com.hazelcast.cache.impl.operation.CacheCreateConfigOperation;
import com.hazelcast.cache.impl.operation.CacheDestroyOperation;
import com.hazelcast.cache.impl.operation.CacheEntryProcessorOperation;
import com.hazelcast.cache.impl.operation.CacheGetAllOperation;
import com.hazelcast.cache.impl.operation.CacheGetAllOperationFactory;
import com.hazelcast.cache.impl.operation.CacheGetAndRemoveOperation;
import com.hazelcast.cache.impl.operation.CacheGetAndReplaceOperation;
import com.hazelcast.cache.impl.operation.CacheGetConfigOperation;
import com.hazelcast.cache.impl.operation.CacheGetOperation;
import com.hazelcast.cache.impl.operation.CacheKeyIteratorOperation;
import com.hazelcast.cache.impl.operation.CacheListenerRegistrationOperation;
import com.hazelcast.cache.impl.operation.CacheLoadAllOperation;
import com.hazelcast.cache.impl.operation.CacheLoadAllOperationFactory;
import com.hazelcast.cache.impl.operation.CacheManagementConfigOperation;
import com.hazelcast.cache.impl.operation.CacheMergeOperation;
import com.hazelcast.cache.impl.operation.CachePutAllBackupOperation;
import com.hazelcast.cache.impl.operation.CachePutAllOperation;
import com.hazelcast.cache.impl.operation.CachePutBackupOperation;
import com.hazelcast.cache.impl.operation.CachePutIfAbsentOperation;
import com.hazelcast.cache.impl.operation.CachePutOperation;
import com.hazelcast.cache.impl.operation.CacheRemoveAllBackupOperation;
import com.hazelcast.cache.impl.operation.CacheRemoveAllOperation;
import com.hazelcast.cache.impl.operation.CacheRemoveAllOperationFactory;
import com.hazelcast.cache.impl.operation.CacheRemoveBackupOperation;
import com.hazelcast.cache.impl.operation.CacheRemoveOperation;
import com.hazelcast.cache.impl.operation.CacheReplaceOperation;
import com.hazelcast.cache.impl.operation.CacheSizeOperation;
import com.hazelcast.cache.impl.operation.CacheSizeOperationFactory;
import com.hazelcast.nio.serialization.DataSerializableFactory;
import com.hazelcast.internal.serialization.DataSerializerHook;
import com.hazelcast.nio.serialization.IdentifiedDataSerializable;
import com.hazelcast.internal.serialization.impl.ArrayDataSerializableFactory;
import com.hazelcast.internal.serialization.impl.FactoryIdHelper;
import com.hazelcast.util.ConstructorFunction;
import static com.hazelcast.internal.serialization.impl.FactoryIdHelper.CACHE_DS_FACTORY;
import static com.hazelcast.internal.serialization.impl.FactoryIdHelper.CACHE_DS_FACTORY_ID;
/**
* {@link CacheDataSerializerHook} contains all the ID hooks for {@link IdentifiedDataSerializable} classes used
* inside the JCache framework.
* CacheProxy operations are mapped here. This factory class is used by internal serialization system to create
* {@link IdentifiedDataSerializable} classes without using reflection.
*/
public final class CacheDataSerializerHook
implements DataSerializerHook {
public static final int F_ID = FactoryIdHelper.getFactoryId(CACHE_DS_FACTORY, CACHE_DS_FACTORY_ID);
public static final short GET = 1;
public static final short CONTAINS_KEY = 2;
public static final short PUT = 3;
public static final short PUT_IF_ABSENT = 4;
public static final short REMOVE = 5;
public static final short GET_AND_REMOVE = 6;
public static final short REPLACE = 7;
public static final short GET_AND_REPLACE = 8;
public static final short PUT_BACKUP = 9;
public static final short PUT_ALL_BACKUP = 10;
public static final short REMOVE_BACKUP = 11;
public static final short CLEAR_BACKUP = 12;
public static final short SIZE = 13;
public static final short SIZE_FACTORY = 14;
public static final short CLEAR = 15;
public static final short CLEAR_FACTORY = 16;
public static final short GET_ALL = 17;
public static final short GET_ALL_FACTORY = 18;
public static final short LOAD_ALL = 19;
public static final short LOAD_ALL_FACTORY = 20;
public static final short EXPIRY_POLICY = 21;
public static final short KEY_ITERATOR = 22;
public static final short KEY_ITERATION_RESULT = 23;
public static final short ENTRY_PROCESSOR = 24;
public static final short CLEAR_RESPONSE = 25;
public static final short CREATE_CONFIG = 26;
public static final short GET_CONFIG = 27;
public static final short MANAGEMENT_CONFIG = 28;
public static final short LISTENER_REGISTRATION = 29;
public static final short DESTROY_CACHE = 30;
public static final short CACHE_EVENT_DATA = 31;
public static final short CACHE_EVENT_DATA_SET = 32;
public static final short BACKUP_ENTRY_PROCESSOR = 33;
public static final short REMOVE_ALL = 34;
public static final short REMOVE_ALL_BACKUP = 35;
public static final short REMOVE_ALL_FACTORY = 36;
public static final short PUT_ALL = 37;
public static final short MERGE = 38;
private static final int LEN = 39;
public int getFactoryId() {
return F_ID;
}
public DataSerializableFactory createFactory() {
ConstructorFunction[] constructors = new ConstructorFunction[LEN];
constructors[GET] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheGetOperation();
}
};
constructors[CONTAINS_KEY] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheContainsKeyOperation();
}
};
constructors[PUT] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CachePutOperation();
}
};
constructors[PUT_IF_ABSENT] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CachePutIfAbsentOperation();
}
};
constructors[REMOVE] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheRemoveOperation();
}
};
constructors[GET_AND_REMOVE] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheGetAndRemoveOperation();
}
};
constructors[REPLACE] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheReplaceOperation();
}
};
constructors[GET_AND_REPLACE] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheGetAndReplaceOperation();
}
};
constructors[PUT_BACKUP] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CachePutBackupOperation();
}
};
constructors[PUT_ALL_BACKUP] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CachePutAllBackupOperation();
}
};
constructors[REMOVE_BACKUP] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheRemoveBackupOperation();
}
};
constructors[SIZE] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheSizeOperation();
}
};
constructors[SIZE_FACTORY] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheSizeOperationFactory();
}
};
constructors[CLEAR_FACTORY] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheClearOperationFactory();
}
};
constructors[GET_ALL] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheGetAllOperation();
}
};
constructors[GET_ALL_FACTORY] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheGetAllOperationFactory();
}
};
constructors[LOAD_ALL] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheLoadAllOperation();
}
};
constructors[LOAD_ALL_FACTORY] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheLoadAllOperationFactory();
}
};
constructors[EXPIRY_POLICY] = new ConstructorFunction() {
@Override
public IdentifiedDataSerializable createNew(Integer arg) {
return new HazelcastExpiryPolicy();
}
};
constructors[KEY_ITERATOR] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheKeyIteratorOperation();
}
};
constructors[KEY_ITERATION_RESULT] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheKeyIteratorResult();
}
};
constructors[ENTRY_PROCESSOR] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheEntryProcessorOperation();
}
};
constructors[CLEAR_RESPONSE] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheClearResponse();
}
};
constructors[CREATE_CONFIG] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheCreateConfigOperation();
}
};
constructors[GET_CONFIG] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheGetConfigOperation();
}
};
constructors[MANAGEMENT_CONFIG] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheManagementConfigOperation();
}
};
constructors[LISTENER_REGISTRATION] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheListenerRegistrationOperation();
}
};
constructors[DESTROY_CACHE] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheDestroyOperation();
}
};
constructors[CACHE_EVENT_DATA] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheEventDataImpl();
}
};
constructors[CACHE_EVENT_DATA_SET] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheEventSet();
}
};
constructors[BACKUP_ENTRY_PROCESSOR] = new ConstructorFunction() {
@Override
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheBackupEntryProcessorOperation();
}
};
constructors[CLEAR] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheClearOperation();
}
};
constructors[CLEAR_BACKUP] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheClearBackupOperation();
}
};
constructors[REMOVE_ALL] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheRemoveAllOperation();
}
};
constructors[REMOVE_ALL_BACKUP] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheRemoveAllBackupOperation();
}
};
constructors[REMOVE_ALL_FACTORY] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheRemoveAllOperationFactory();
}
};
constructors[PUT_ALL] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CachePutAllOperation();
}
};
constructors[MERGE] = new ConstructorFunction() {
public IdentifiedDataSerializable createNew(Integer arg) {
return new CacheMergeOperation();
}
};
return new ArrayDataSerializableFactory(constructors);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy