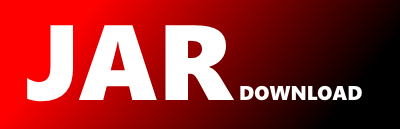
com.hazelcast.config.ConfigXmlGenerator Maven / Gradle / Ivy
/*
* Copyright (c) 2008-2016, Hazelcast, Inc. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.hazelcast.config;
import com.hazelcast.config.CacheSimpleConfig.ExpiryPolicyFactoryConfig;
import com.hazelcast.config.CacheSimpleConfig.ExpiryPolicyFactoryConfig.DurationConfig;
import com.hazelcast.config.CacheSimpleConfig.ExpiryPolicyFactoryConfig.TimedExpiryPolicyFactoryConfig;
import com.hazelcast.config.CacheSimpleConfig.ExpiryPolicyFactoryConfig.TimedExpiryPolicyFactoryConfig.ExpiryPolicyType;
import com.hazelcast.logging.ILogger;
import com.hazelcast.logging.Logger;
import com.hazelcast.util.StringUtil;
import javax.xml.transform.OutputKeys;
import javax.xml.transform.Source;
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerFactory;
import javax.xml.transform.stream.StreamResult;
import javax.xml.transform.stream.StreamSource;
import java.io.StringReader;
import java.io.StringWriter;
import java.util.Collection;
import java.util.List;
import java.util.Properties;
import java.util.Set;
import static com.hazelcast.nio.IOUtil.closeResource;
import static com.hazelcast.util.Preconditions.isNotNull;
/**
* The ConfigXmlGenerator is responsible for transforming a {@link Config} to a Hazelcast XML string.
*/
public class ConfigXmlGenerator {
private static final ILogger LOGGER = Logger.getLogger(ConfigXmlGenerator.class);
private static final int INDENT = 5;
private final boolean formatted;
/**
* Creates a ConfigXmlGenerator that will format the code.
*/
public ConfigXmlGenerator() {
this(true);
}
/**
* Creates a ConfigXmlGenerator.
*
* @param formatted true if the XML should be formatted, false otherwise.
*/
public ConfigXmlGenerator(boolean formatted) {
this.formatted = formatted;
}
/**
* Generates the XML string based on some Config.
*
* @param config the configuration.
* @return the XML string.
*/
public String generate(Config config) {
isNotNull(config, "Config");
final StringBuilder xml = new StringBuilder();
xml.append("");
xml.append("");
xml.append("").append(config.getGroupConfig().getName()).append(" ");
xml.append("").append("****").append(" ");
xml.append(" ");
if (config.getLicenseKey() != null) {
xml.append("").append(config.getLicenseKey()).append(" ");
}
if (config.getManagementCenterConfig() != null) {
ManagementCenterConfig mcConfig = config.getManagementCenterConfig();
xml.append("")
.append(mcConfig.getUrl()).append(" ");
}
appendProperties(xml, config.getProperties());
wanReplicationXmlGenerator(xml, config);
networkConfigXmlGenerator(xml, config);
mapConfigXmlGenerator(xml, config);
replicatedMapConfigXmlGenerator(xml, config);
cacheConfigXmlGenerator(xml, config);
queueXmlGenerator(xml, config);
multiMapXmlGenerator(xml, config);
topicXmlGenerator(xml, config);
semaphoreXmlGenerator(xml, config);
ringbufferXmlGenerator(xml, config);
executorXmlGenerator(xml, config);
partitionGroupXmlGenerator(xml, config);
listenerXmlGenerator(xml, config);
reliableTopicXmlGenerator(xml, config);
liteMemberXmlGenerator(xml, config);
hotRestartXmlGenerator(xml, config);
xml.append(" ");
return format(xml.toString(), INDENT);
}
private void replicatedMapConfigXmlGenerator(StringBuilder xml, Config config) {
final Collection replicatedMapConfigs = config.getReplicatedMapConfigs().values();
for (ReplicatedMapConfig r : replicatedMapConfigs) {
xml.append("");
xml.append("").append(r.getInMemoryFormat()).append(" ");
xml.append("").append(r.getConcurrencyLevel()).append(" ");
xml.append("").append(r.getReplicationDelayMillis()).append(" ");
xml.append("").append(r.isAsyncFillup()).append(" ");
xml.append("").append(r.isStatisticsEnabled()).append(" ");
if (!r.getListenerConfigs().isEmpty()) {
xml.append("");
for (ListenerConfig lc : r.getListenerConfigs()) {
xml.append("");
final String clazz = lc.getImplementation()
!= null ? lc.getImplementation().getClass().getName() : lc.getClassName();
xml.append(clazz);
xml.append(" ");
}
xml.append(" ");
}
xml.append(" ");
}
}
private void listenerXmlGenerator(StringBuilder xml, Config config) {
if (!config.getListenerConfigs().isEmpty()) {
xml.append("");
for (ListenerConfig lc : config.getListenerConfigs()) {
xml.append("");
final String clazz = lc.getImplementation()
!= null ? lc.getImplementation().getClass().getName() : lc.getClassName();
xml.append(clazz);
xml.append(" ");
}
xml.append(" ");
}
}
private void partitionGroupXmlGenerator(StringBuilder xml, Config config) {
final PartitionGroupConfig pg = config.getPartitionGroupConfig();
if (pg != null) {
xml.append(" ");
}
}
private void executorXmlGenerator(StringBuilder xml, Config config) {
final Collection exCfgs = config.getExecutorConfigs().values();
for (ExecutorConfig ex : exCfgs) {
xml.append("");
xml.append("").append(ex.getPoolSize()).append(" ");
xml.append("").append(ex.getQueueCapacity()).append(" ");
xml.append(" ");
}
}
private void semaphoreXmlGenerator(StringBuilder xml, Config config) {
final Collection semaphoreCfgs = config.getSemaphoreConfigs();
for (SemaphoreConfig sc : semaphoreCfgs) {
xml.append("");
xml.append("").append(sc.getInitialPermits()).append(" ");
xml.append("").append(sc.getBackupCount()).append(" ");
xml.append("").append(sc.getAsyncBackupCount()).append(" ");
xml.append(" ");
}
}
private void topicXmlGenerator(StringBuilder xml, Config config) {
final Collection tCfgs = config.getTopicConfigs().values();
for (TopicConfig t : tCfgs) {
xml.append("");
xml.append("").append(t.isGlobalOrderingEnabled())
.append(" ");
if (!t.getMessageListenerConfigs().isEmpty()) {
xml.append("");
for (ListenerConfig lc : t.getMessageListenerConfigs()) {
xml.append("");
final String clazz = lc.getImplementation()
!= null ? lc.getImplementation().getClass().getName() : lc.getClassName();
xml.append(clazz);
xml.append(" ");
}
xml.append(" ");
}
xml.append(" ");
}
}
private void reliableTopicXmlGenerator(StringBuilder xml, Config config) {
final Collection tCfgs = config.getReliableTopicConfigs().values();
for (ReliableTopicConfig t : tCfgs) {
xml.append("");
xml.append("").append(t.getReadBatchSize()).append(" ");
xml.append("").append(t.isStatisticsEnabled()).append(" ");
xml.append("").append(t.getTopicOverloadPolicy().name()).append(" ");
if (!t.getMessageListenerConfigs().isEmpty()) {
xml.append("");
for (ListenerConfig lc : t.getMessageListenerConfigs()) {
xml.append("");
final String clazz = lc.getImplementation()
!= null ? lc.getImplementation().getClass().getName() : lc.getClassName();
xml.append(clazz);
xml.append(" ");
}
xml.append(" ");
}
xml.append(" ");
}
}
private void multiMapXmlGenerator(StringBuilder xml, Config config) {
final Collection mmCfgs = config.getMultiMapConfigs().values();
for (MultiMapConfig mm : mmCfgs) {
xml.append("");
xml.append("").append(mm.getValueCollectionType()).append(" ");
if (!mm.getEntryListenerConfigs().isEmpty()) {
xml.append("");
for (EntryListenerConfig lc : mm.getEntryListenerConfigs()) {
xml.append("");
final String clazz = lc.getImplementation()
!= null ? lc.getImplementation().getClass().getName() : lc.getClassName();
xml.append(clazz);
xml.append(" ");
}
xml.append(" ");
}
// if (mm.getPartitioningStrategyConfig() != null) {
// xml.append("");
// PartitioningStrategyConfig psc = mm.getPartitioningStrategyConfig();
// if (psc.getPartitioningStrategy() != null) {
// xml.append(psc.getPartitioningStrategy().getClass().getName());
// } else {
// xml.append(psc.getPartitioningStrategyClass());
// }
// xml.append(" ");
// }
xml.append(" ");
}
}
private void queueXmlGenerator(StringBuilder xml, Config config) {
final Collection qCfgs = config.getQueueConfigs().values();
for (QueueConfig q : qCfgs) {
xml.append("");
xml.append("").append(q.getMaxSize()).append(" ");
xml.append("").append(q.getBackupCount()).append(" ");
xml.append("").append(q.getAsyncBackupCount()).append(" ");
if (!q.getItemListenerConfigs().isEmpty()) {
xml.append("");
for (ItemListenerConfig lc : q.getItemListenerConfigs()) {
xml.append("");
xml.append(lc.getClassName());
xml.append(" ");
}
xml.append(" ");
}
xml.append(" ");
}
}
private void ringbufferXmlGenerator(StringBuilder xml, Config config) {
final Collection configs = config.getRingbufferConfigs().values();
for (RingbufferConfig rbConfig : configs) {
xml.append("");
xml.append("").append(rbConfig.getCapacity()).append(" ");
xml.append("").append(rbConfig.getBackupCount()).append(" ");
xml.append("").append(rbConfig.getAsyncBackupCount()).append(" ");
xml.append("").append(rbConfig.getTimeToLiveSeconds()).append(" ");
xml.append("").append(rbConfig.getInMemoryFormat().toString()).append(" ");
xml.append(" ");
}
}
private void wanReplicationXmlGenerator(StringBuilder xml, Config config) {
final Collection wanRepConfigs = config.getWanReplicationConfigs().values();
for (WanReplicationConfig wan : wanRepConfigs) {
xml.append("");
final List targets = wan.getTargetClusterConfigs();
for (WanTargetClusterConfig t : targets) {
xml.append("");
xml.append("").append(t.getReplicationImpl()).append(" ");
xml.append("");
final List eps = t.getEndpoints();
for (String ep : eps) {
xml.append("").append(ep).append("");
}
xml.append(" ").append(" ");
}
xml.append(" ");
}
}
private void networkConfigXmlGenerator(StringBuilder xml, Config config) {
final NetworkConfig netCfg = config.getNetworkConfig();
xml.append("");
if (netCfg.getPublicAddress() != null) {
xml.append("").append(netCfg.getPublicAddress()).append(" ");
}
xml.append("")
.append(netCfg.getPort()).append(" ");
final JoinConfig join = netCfg.getJoin();
xml.append("");
multicastConfigXmlGenerator(xml, join);
tcpConfigXmlGenerator(xml, join);
awsConfigXmlGenerator(xml, join);
xml.append(" ");
interfacesConfigXmlGenerator(xml, netCfg);
sslConfigXmlGenerator(xml, netCfg);
socketInterceptorConfigXmlGenerator(xml, netCfg);
symmetricEncInterceptorConfigXmlGenerator(xml, netCfg);
xml.append(" ");
}
private void mapConfigXmlGenerator(StringBuilder xml, Config config) {
final Collection mCfgs = config.getMapConfigs().values();
for (MapConfig m : mCfgs) {
xml.append("");
}
private void mapEntryListenerConfigXmlGenerator(StringBuilder xml, MapConfig m) {
if (!m.getEntryListenerConfigs().isEmpty()) {
xml.append("");
for (EntryListenerConfig lc : m.getEntryListenerConfigs()) {
xml.append("");
final String clazz = lc.getImplementation()
!= null ? lc.getImplementation().getClass().getName() : lc.getClassName();
xml.append(clazz);
xml.append(" ");
}
xml.append(" ");
}
}
private void mapPartitionLostListenerConfigXmlGenerator(StringBuilder xml, MapConfig m) {
if (!m.getPartitionLostListenerConfigs().isEmpty()) {
xml.append("");
for (MapPartitionLostListenerConfig c : m.getPartitionLostListenerConfigs()) {
xml.append("");
final String clazz = c.getImplementation()
!= null ? c.getImplementation().getClass().getName() : c.getClassName();
xml.append(clazz);
xml.append(" ");
}
xml.append(" ");
}
}
private void mapIndexConfigXmlGenerator(StringBuilder xml, MapConfig m) {
if (!m.getMapIndexConfigs().isEmpty()) {
xml.append("");
for (MapIndexConfig indexCfg : m.getMapIndexConfigs()) {
xml.append("");
xml.append(indexCfg.getAttribute());
xml.append(" ");
}
xml.append(" ");
}
}
private void mapAttributeConfigXmlGenerator(StringBuilder xml, MapConfig m) {
if (!m.getMapAttributeConfigs().isEmpty()) {
xml.append("");
for (MapAttributeConfig attributeCfg : m.getMapAttributeConfigs()) {
xml.append("");
xml.append(attributeCfg.getName());
xml.append(" ");
}
xml.append(" ");
}
}
private void wanReplicationConfigXmlGenerator(StringBuilder xml, WanReplicationRef wan) {
if (wan != null) {
xml.append("");
xml.append("").append(wan.getMergePolicy()).append(" ");
xml.append("").append(wan.isRepublishingEnabled()).append(" ");
xml.append(" ");
}
}
private void mapStoreConfigXmlGenerator(StringBuilder xml, MapConfig m) {
if (m.getMapStoreConfig() != null) {
final MapStoreConfig s = m.getMapStoreConfig();
xml.append("");
final String clazz = s.getImplementation()
!= null ? s.getImplementation().getClass().getName() : s.getClassName();
xml.append("").append(clazz).append(" ");
final String factoryClass = s.getFactoryImplementation() != null
? s.getFactoryImplementation().getClass().getName()
: s.getFactoryClassName();
if (factoryClass != null) {
xml.append("").append(factoryClass).append(" ");
}
xml.append("").append(s.getWriteDelaySeconds()).append(" ");
xml.append("").append(s.getWriteBatchSize()).append(" ");
appendProperties(xml, s.getProperties());
xml.append(" ");
}
}
private void nearCacheConfigXmlGenerator(StringBuilder xml, NearCacheConfig n) {
if (n != null) {
xml.append("");
xml.append("").append(n.getMaxSize()).append(" ");
xml.append("").append(n.getTimeToLiveSeconds()).append(" ");
xml.append("").append(n.getMaxIdleSeconds()).append(" ");
xml.append("").append(n.getEvictionPolicy()).append(" ");
xml.append("").append(n.isInvalidateOnChange()).append(" ");
xml.append("").append(n.getLocalUpdatePolicy()).append(" ");
xml.append("").append(n.getInMemoryFormat()).append(" ");
evictionConfigXmlGenerator(xml, n.getEvictionConfig());
xml.append(" ");
}
}
private void evictionConfigXmlGenerator(StringBuilder xml, EvictionConfig e) {
if (e != null) {
xml.append(" ");
}
}
private void multicastConfigXmlGenerator(StringBuilder xml, JoinConfig join) {
final MulticastConfig mcast = join.getMulticastConfig();
xml.append("");
xml.append("").append(mcast.getMulticastGroup()).append(" ");
xml.append("").append(mcast.getMulticastPort()).append(" ");
xml.append("").append(mcast.getMulticastTimeoutSeconds())
.append(" ");
xml.append("").append(mcast.getMulticastTimeToLive())
.append(" ");
if (!mcast.getTrustedInterfaces().isEmpty()) {
xml.append("");
for (String trustedInterface : mcast.getTrustedInterfaces()) {
xml.append("").append(trustedInterface).append(" ");
}
xml.append(" ");
}
xml.append(" ");
}
private void tcpConfigXmlGenerator(StringBuilder xml, JoinConfig join) {
final TcpIpConfig tcpCfg = join.getTcpIpConfig();
xml.append("");
final List members = tcpCfg.getMembers();
xml.append("");
for (String m : members) {
xml.append("").append(m).append(" ");
}
xml.append(" ");
if (tcpCfg.getRequiredMember() != null) {
xml.append("").append(tcpCfg.getRequiredMember()).append(" ");
}
xml.append(" ");
}
private void awsConfigXmlGenerator(StringBuilder xml, JoinConfig join) {
final AwsConfig awsConfig = join.getAwsConfig();
xml.append("");
xml.append("").append(awsConfig.getAccessKey()).append(" ");
xml.append("").append(awsConfig.getSecretKey()).append(" ");
xml.append("").append(awsConfig.getRegion()).append(" ");
xml.append("").append(awsConfig.getSecurityGroupName()).append(" ");
xml.append("").append(awsConfig.getTagKey()).append(" ");
xml.append("").append(awsConfig.getTagValue()).append(" ");
xml.append(" ");
}
private void interfacesConfigXmlGenerator(StringBuilder xml, NetworkConfig netCfg) {
final InterfacesConfig interfaces = netCfg.getInterfaces();
xml.append("");
final Collection interfaceList = interfaces.getInterfaces();
for (String i : interfaceList) {
xml.append("").append(i).append(" ");
}
xml.append(" ");
}
private void sslConfigXmlGenerator(StringBuilder xml, NetworkConfig netCfg) {
final SSLConfig ssl = netCfg.getSSLConfig();
xml.append("");
if (ssl != null) {
String className = ssl.getFactoryImplementation() != null
? ssl.getFactoryImplementation().getClass().getName()
: ssl.getFactoryClassName();
xml.append("").append(className).append(" ");
appendProperties(xml, ssl.getProperties());
}
xml.append(" ");
}
private void socketInterceptorConfigXmlGenerator(StringBuilder xml, NetworkConfig netCfg) {
final SocketInterceptorConfig socket = netCfg.getSocketInterceptorConfig();
xml.append("");
if (socket != null) {
String className = socket.getImplementation() != null
? socket.getImplementation().getClass().getName() : socket.getClassName();
xml.append("").append(className).append(" ");
appendProperties(xml, socket.getProperties());
}
xml.append(" ");
}
private void symmetricEncInterceptorConfigXmlGenerator(StringBuilder xml, NetworkConfig netCfg) {
final SymmetricEncryptionConfig sec = netCfg.getSymmetricEncryptionConfig();
if (sec != null) {
xml.append("");
xml.append("").append(sec.getAlgorithm()).append(" ");
xml.append("").append(sec.getSalt()).append(" ");
xml.append("").append(sec.getPassword()).append(" ");
xml.append("").append(sec.getIterationCount()).append(" ");
xml.append(" ");
}
}
private void hotRestartXmlGenerator(StringBuilder xml, Config config) {
HotRestartPersistenceConfig hotRestartPersistenceConfig = config.getHotRestartPersistenceConfig();
if (hotRestartPersistenceConfig == null) {
xml.append(" ");
return;
}
xml.append("");
xml.append("").append(hotRestartPersistenceConfig.getBaseDir().getAbsolutePath()).append(" ");
xml.append("")
.append(hotRestartPersistenceConfig.getValidationTimeoutSeconds())
.append(" ");
xml.append("")
.append(hotRestartPersistenceConfig.getDataLoadTimeoutSeconds())
.append(" ");
xml.append(" ");
}
private void liteMemberXmlGenerator(StringBuilder xml, Config config) {
xml.append("");
}
private String format(final String input, int indent) {
if (!formatted) {
return input;
}
StreamResult xmlOutput = null;
try {
final Source xmlInput = new StreamSource(new StringReader(input));
xmlOutput = new StreamResult(new StringWriter());
TransformerFactory transformerFactory = TransformerFactory.newInstance();
/* Older versions of Xalan still use this method of setting indent values.
* Attempt to make this work but don't completely fail if it's a problem.
*/
try {
transformerFactory.setAttribute("indent-number", indent);
} catch (IllegalArgumentException e) {
if (LOGGER.isFinestEnabled()) {
LOGGER.finest("Failed to set indent-number attribute; cause: " + e.getMessage());
}
}
Transformer transformer = transformerFactory.newTransformer();
transformer.setOutputProperty(OutputKeys.OMIT_XML_DECLARATION, "yes");
transformer.setOutputProperty(OutputKeys.ENCODING, "UTF-8");
transformer.setOutputProperty(OutputKeys.INDENT, "yes");
/* Newer versions of Xalan will look for a fully-qualified output property in order to specify amount of
* indentation to use. Attempt to make this work as well but again don't completely fail if it's a problem.
*/
try {
transformer.setOutputProperty("{http://xml.apache.org/xslt}indent-amount", Integer.toString(indent));
} catch (IllegalArgumentException e) {
if (LOGGER.isFinestEnabled()) {
LOGGER.finest("Failed to set indent-amount property; cause: " + e.getMessage());
}
}
transformer.transform(xmlInput, xmlOutput);
String response = xmlOutput.getWriter().toString();
return response;
} catch (Exception e) {
LOGGER.warning(e);
return input;
} finally {
if (xmlOutput != null) {
closeResource(xmlOutput.getWriter());
}
}
}
private void appendProperties(StringBuilder xml, Properties props) {
if (!props.isEmpty()) {
xml.append("");
Set keys = props.keySet();
for (Object key : keys) {
xml.append("")
.append(props.getProperty(key.toString()))
.append(" ");
}
xml.append(" ");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy