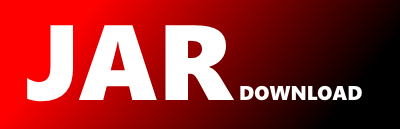
com.hazelcast.org.apache.calcite.adapter.jdbc.JdbcUtils Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to you under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.hazelcast.org.apache.calcite.adapter.jdbc;
import com.hazelcast.org.apache.calcite.avatica.ColumnMetaData;
import com.hazelcast.org.apache.calcite.avatica.util.DateTimeUtils;
import com.hazelcast.org.apache.calcite.linq4j.function.Function0;
import com.hazelcast.org.apache.calcite.linq4j.function.Function1;
import com.hazelcast.org.apache.calcite.sql.SqlDialect;
import com.hazelcast.org.apache.calcite.sql.SqlDialectFactory;
import com.hazelcast.org.apache.calcite.util.ImmutableNullableList;
import com.hazelcast.org.apache.calcite.util.Pair;
import com.hazelcast.org.apache.commons.dbcp2.BasicDataSource;
import com.hazelcast.com.google.common.cache.CacheBuilder;
import com.hazelcast.com.google.common.cache.CacheLoader;
import com.hazelcast.com.google.common.cache.LoadingCache;
import com.hazelcast.com.google.common.collect.ImmutableList;
import com.hazelcast.com.google.common.primitives.Ints;
import java.sql.Connection;
import java.sql.DatabaseMetaData;
import java.sql.Date;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Time;
import java.sql.Timestamp;
import java.sql.Types;
import java.util.HashMap;
import java.util.IdentityHashMap;
import java.util.List;
import java.util.Map;
import java.util.TimeZone;
import javax.annotation.Nonnull;
import javax.sql.DataSource;
/**
* Utilities for the JDBC provider.
*/
final class JdbcUtils {
private JdbcUtils() {
throw new AssertionError("no instances!");
}
/** Pool of dialects. */
static class DialectPool {
final Map> map0 = new IdentityHashMap<>();
final Map map = new HashMap<>();
public static final DialectPool INSTANCE = new DialectPool();
// TODO: Discuss why we need a pool. If we do, I'd like to improve performance
synchronized SqlDialect get(SqlDialectFactory dialectFactory, DataSource dataSource) {
Map dialectMap = map0.get(dataSource);
if (dialectMap != null) {
final SqlDialect sqlDialect = dialectMap.get(dialectFactory);
if (sqlDialect != null) {
return sqlDialect;
}
}
Connection connection = null;
try {
connection = dataSource.getConnection();
DatabaseMetaData metaData = connection.getMetaData();
String productName = metaData.getDatabaseProductName();
String productVersion = metaData.getDatabaseProductVersion();
List key = ImmutableList.of(productName, productVersion, dialectFactory);
SqlDialect dialect = map.get(key);
if (dialect == null) {
dialect = dialectFactory.create(metaData);
map.put(key, dialect);
if (dialectMap == null) {
dialectMap = new IdentityHashMap<>();
map0.put(dataSource, dialectMap);
}
dialectMap.put(dialectFactory, dialect);
}
connection.close();
connection = null;
return dialect;
} catch (SQLException e) {
throw new RuntimeException(e);
} finally {
if (connection != null) {
try {
connection.close();
} catch (SQLException e) {
// ignore
}
}
}
}
}
/** Builder that calls {@link ResultSet#getObject(int)} for every column,
* or {@code getXxx} if the result type is a primitive {@code xxx},
* and returns an array of objects for each row. */
static class ObjectArrayRowBuilder implements Function0
© 2015 - 2025 Weber Informatics LLC | Privacy Policy