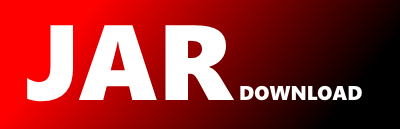
com.hazelcast.org.apache.calcite.schema.impl.ViewTableMacro Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to you under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.hazelcast.org.apache.calcite.schema.impl;
import com.hazelcast.org.apache.calcite.adapter.java.JavaTypeFactory;
import com.hazelcast.org.apache.calcite.jdbc.CalciteConnection;
import com.hazelcast.org.apache.calcite.jdbc.CalcitePrepare;
import com.hazelcast.org.apache.calcite.jdbc.CalciteSchema;
import com.hazelcast.org.apache.calcite.rel.type.RelDataTypeImpl;
import com.hazelcast.org.apache.calcite.schema.FunctionParameter;
import com.hazelcast.org.apache.calcite.schema.Schemas;
import com.hazelcast.org.apache.calcite.schema.TableMacro;
import com.hazelcast.org.apache.calcite.schema.TranslatableTable;
import com.hazelcast.com.google.common.collect.ImmutableList;
import com.hazelcast.org.checkerframework.checker.nullness.qual.Nullable;
import java.lang.reflect.Type;
import java.util.Collections;
import java.util.List;
import static java.util.Objects.requireNonNull;
/** Table function that implements a view. It returns the operator
* tree of the view's SQL query. */
public class ViewTableMacro implements TableMacro {
protected final String viewSql;
protected final CalciteSchema schema;
private final @Nullable Boolean modifiable;
/** Typically null. If specified, overrides the path of the schema as the
* context for validating {@code viewSql}. */
protected final @Nullable List schemaPath;
protected final @Nullable List viewPath;
/**
* Creates a ViewTableMacro.
*
* @param schema Root schema
* @param viewSql SQL defining the view
* @param schemaPath Schema path relative to the root schema
* @param viewPath View path relative to the schema path
* @param modifiable Request that a view is modifiable (dependent on analysis
* of {@code viewSql})
*/
public ViewTableMacro(CalciteSchema schema, String viewSql,
@Nullable List schemaPath, @Nullable List viewPath,
@Nullable Boolean modifiable) {
this.viewSql = viewSql;
this.schema = schema;
this.viewPath = viewPath == null ? null : ImmutableList.copyOf(viewPath);
this.modifiable = modifiable;
this.schemaPath =
schemaPath == null ? null : ImmutableList.copyOf(schemaPath);
}
@Override public List getParameters() {
return Collections.emptyList();
}
@Override public TranslatableTable apply(List extends @Nullable Object> arguments) {
final CalciteConnection connection =
MaterializedViewTable.MATERIALIZATION_CONNECTION;
CalcitePrepare.AnalyzeViewResult parsed =
Schemas.analyzeView(connection, schema, schemaPath, viewSql, viewPath,
modifiable != null && modifiable);
final List schemaPath1 =
schemaPath != null ? schemaPath : schema.path(null);
if ((modifiable == null || modifiable)
&& parsed.modifiable
&& parsed.table != null) {
return modifiableViewTable(parsed, viewSql, schemaPath1, viewPath, schema);
} else {
return viewTable(parsed, viewSql, schemaPath1, viewPath);
}
}
/** Allows a sub-class to return an extension of {@link ModifiableViewTable}
* by overriding this method. */
protected ModifiableViewTable modifiableViewTable(CalcitePrepare.AnalyzeViewResult parsed,
String viewSql, List schemaPath, @Nullable List viewPath,
CalciteSchema schema) {
final JavaTypeFactory typeFactory = (JavaTypeFactory) parsed.typeFactory;
final Type elementType = typeFactory.getJavaClass(parsed.rowType);
return new ModifiableViewTable(elementType,
RelDataTypeImpl.proto(parsed.rowType), viewSql, schemaPath, viewPath,
requireNonNull(parsed.table, "parsed.table"),
Schemas.path(schema.root(), requireNonNull(parsed.tablePath, "parsed.tablePath")),
requireNonNull(parsed.constraint, "parsed.constraint"),
requireNonNull(parsed.columnMapping, "parsed.columnMapping"));
}
/** Allows a sub-class to return an extension of {@link ViewTable} by
* overriding this method. */
protected ViewTable viewTable(CalcitePrepare.AnalyzeViewResult parsed,
String viewSql, List schemaPath, @Nullable List viewPath) {
final JavaTypeFactory typeFactory = (JavaTypeFactory) parsed.typeFactory;
final Type elementType = typeFactory.getJavaClass(parsed.rowType);
return new ViewTable(elementType,
RelDataTypeImpl.proto(parsed.rowType), viewSql, schemaPath, viewPath);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy