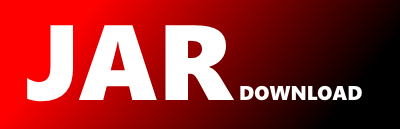
com.hazelcast.org.codehaus.commons.compiler.IExpressionEvaluator Maven / Gradle / Ivy
/*
* Janino - An embedded Java[TM] compiler
*
* Copyright (c) 2001-2010 Arno Unkrig. All rights reserved.
*
* Redistribution and use in source and binary forms, with or without modification, are permitted provided that the
* following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice, this list of conditions and the
* following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the
* following disclaimer in the documentation and/or other materials provided with the distribution.
* 3. Neither the name of the copyright holder nor the names of its contributors may be used to endorse or promote
* products derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES,
* INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
* SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
* SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY,
* WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package com.hazelcast.org.codehaus.commons.compiler;
import java.io.IOException;
import java.io.Reader;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import com.hazelcast.org.codehaus.commons.compiler.lang.ClassLoaders;
import com.hazelcast.org.codehaus.commons.nullanalysis.Nullable;
/**
* An engine that evaluates expressions in JVM bytecode.
*
* The syntax of the expression to compile is that of a Java expression, as defined in JLS7, section 15. Notice
* that a Java expression does not have a concluding semicolon.
*
*
* Example:
*
*
* a + 7 * b
*
*
* (Notice that this expression refers to two parameters "a" and "b", as explained below.)
*
*
* The expression may optionally be preceeded with a sequence of import directives like
*
*
* import java.text.*;
* new DecimalFormat("####,###.##").format(10200020.345345)
*
*
* (Notice that the import directive is concluded with a semicolon, while the expression is not.) This feature is not
* available if you compile many expressions at a time (see below).
*
*
* To set up an {@link IExpressionEvaluator} object, proceed as follows:
*
*
* - Create an {@link IExpressionEvaluator}-derived class
* - Configure the {@link IExpressionEvaluator} by calling any of the following methods:
*
* - {@link #setExpressionType(Class)}
*
- {@link #setParameters(String[], Class[])}
*
- {@link #setThrownExceptions(Class[])}
*
- {@link #setParentClassLoader(ClassLoader)}
*
- {@link #setDefaultImports(String[])}
*
* -
* Call any of the {@link #cook(String, java.io.Reader)} methods to scan, parse, compile and load the expression
* into the JVM.
*
*
*
* After the {@link IExpressionEvaluator} object is set up, the expression can be evaluated as often with different
* parameter values (see {@link #evaluate(Object[])}). This evaluation is very fast, compared to the compilation.
*
*
* Less common methods exist that allow for the specification of the name of the generated class, the class it
* extends, the interfaces it implements, the name of the method that executes the expression, the exceptions that
* this method (i.e. the expression) is allowed to throw, and the {@link ClassLoader} that is used to define the
* generated class and to load classes referenced by the expression.
*
*
* If you want to compile many expressions at the same time, you have the option to cook an array of
* expressions in one {@link IExpressionEvaluator} by using the following methods:
*
*
* - {@link #setMethodNames(String[])}
*
- {@link #setParameters(String[][], Class[][])}
*
- {@link #setExpressionTypes(Class[])}
*
- {@link #setStaticMethod(boolean[])}
*
- {@link #setThrownExceptions(Class[][])}
*
- {@link #cook(String[], Reader[])}
*
- {@link #evaluate(int, Object[])}
*
*
* Notice that these methods have array parameters in contrast to their one-expression brethren.
*
*
* Notice that for functionally identical {@link IExpressionEvaluator}s, {@link
* java.lang.Object#equals(java.lang.Object)} will return {@code true}. E.g. "a+b" and "c + d" are functionally
* identical if "a" and "c" have the same type, and so do "b" and "d".
*
*
* 'JLS7' refers to the Java Language Specification, Java SE 7
* Edition.
*
*/
public
interface IExpressionEvaluator extends ICookable, IMultiCookable {
/**
* The fully qualified name of the generated class, iff not reconfigured by {@link #setClassName(String)}.
*/
String DEFAULT_CLASS_NAME = "SC";
/**
* The type of all expressions that were not reconfigured with {@link #setExpressionTypes(Class[])}.
*/
Class> DEFAULT_EXPRESSION_TYPE = Object.class;
/**
* Special value for {@link #setExpressionType(Class)} that indicates that the expression may have any type.
*
* @deprecated Since autoboxing was introduced in JANINO, this feature is no longer necessary because you can use
* expression type {@link Object}{@code .class}
*/
@Deprecated Class> ANY_TYPE = Object.class;
/**
* The "parent class loader" is used to load referenced classes. Useful values are:
*
* {@code System.getSystemClassLoader()}
* The running JVM's class path
*
* {@code Thread.currentThread().getContextClassLoader()} or {@code null}
* The class loader effective for the invoking thread
*
* {@link ClassLoaders#BOOTCLASSPATH_CLASS_LOADER}
* The running JVM's boot class path
*
*
* The parent class loader defaults to the current thread's context class loader.
*
*/
void setParentClassLoader(@Nullable ClassLoader parentClassLoader);
/**
* Determines what kind of debugging information is included in the generates classes. The default is typically
* "{@code -g:none}".
*/
void setDebuggingInformation(boolean debugSource, boolean debugLines, boolean debugVars);
/**
* By default, {@link CompileException}s are thrown on compile errors, but an application my install its own
* {@link ErrorHandler}.
*
* Be aware that a single problem during compilation often causes a bunch of compile errors, so a good {@link
* ErrorHandler} counts errors and throws a {@link CompileException} when a limit is reached.
*
*
* If the given {@link ErrorHandler} throws {@link CompileException}s, then the compilation is terminated and
* the exception is propagated.
*
*
* If the given {@link ErrorHandler} does not throw {@link CompileException}s, then the compiler may or may not
* continue compilation, but must eventually throw a {@link CompileException}.
*
*
* In other words: The {@link ErrorHandler} may throw a {@link CompileException} or not, but the compiler must
* definitely throw a {@link CompileException} if one or more compile errors have occurred.
*
*
* @param compileErrorHandler {@code null} to restore the default behavior (throwing a {@link CompileException}
*/
void setCompileErrorHandler(@Nullable ErrorHandler compileErrorHandler);
/**
* By default, warnings are discarded, but an application my install a custom {@link WarningHandler}.
*
* @param warningHandler {@code null} to indicate that no warnings be issued
*/
void setWarningHandler(@Nullable WarningHandler warningHandler);
/**
* Evaluates the expression with concrete parameter values.
*
* Each argument value must have the same type as specified through the "parameterTypes" parameter of {@link
* #setParameters(String[], Class[])}.
*
*
* Arguments of primitive type must passed with their wrapper class objects.
*
*
* The object returned has the class as specified through {@link #setExpressionType(Class)}.
*
*
* This method is thread-safe.
*
*
* {@code Null} arguments is equivalent with {@code new Object[0]}.
*
*
* @param arguments The actual parameter values
*/
@Nullable Object evaluate(@Nullable Object... arguments) throws InvocationTargetException;
/**
* Reconfigures the "default expression type"; if no expression type is configured for an expression, then, when
* cooking this {@link IExpressionEvaluator}, the "default expression type" is used for the expression
*/
void setDefaultExpressionType(Class> defaultExpressionType);
/**
* @return The currently configured "default expression type"
* @see #setDefaultExpressionType(Class)
*/
Class> getDefaultExpressionType();
/**
* Configures the interfaces that the generated class implements.
*/
void setImplementedInterfaces(Class>[] implementedTypes);
/**
* @deprecated Use {@link #setExpressionType(Class)} instead
*/
@Deprecated void setReturnType(@Deprecated Class> returnType);
/**
* Defines the type of the expression.
*
* Defaults to {@link Object}{@code .class}, which, thanks to autoboxing, allows for any expression type
* (including primitive types).
*
*
* If {@code expressionType} is {@code void.class}, then the expression must be an invocation of a {@code void}
* method.
*
*/
void setExpressionType(Class> expressionType);
/**
* Configures the types of the expressions.
*
* Unless this method is called, all expressions have type {@link Object}{@code .class}.
*
*
* If any expression has type {@code void.class}, then it must be an invocation of a {@code void} method.
*
*/
void setExpressionTypes(Class>[] expressionTypes);
/** @see IScriptEvaluator#setOverrideMethod(boolean) */
void setOverrideMethod(boolean overrideMethod);
/** @see IScriptEvaluator#setOverrideMethod(boolean[]) */
void setOverrideMethod(boolean[] overrideMethod);
/** @see IScriptEvaluator#setParameters(String[], Class[]) */
void setParameters(String[] parameterNames, Class>[] parameterTypes);
/** @see IScriptEvaluator#setParameters(String[][], Class[][]) */
void setParameters(String[][] parameterNames, Class>[][] parameterTypes);
/** @see IClassBodyEvaluator#setClassName(String) */
void setClassName(String className);
/** @see IClassBodyEvaluator#setExtendedClass(Class) */
void setExtendedClass(Class> extendedType);
/** @see IClassBodyEvaluator#setDefaultImports(String...) */
void setDefaultImports(String... defaultImports);
/** @see IClassBodyEvaluator#getDefaultImports() */
String[] getDefaultImports();
/** @see IScriptEvaluator#setStaticMethod(boolean) */
void setStaticMethod(boolean staticMethod);
/** @see IScriptEvaluator#setStaticMethod(boolean[]) */
void setStaticMethod(boolean[] staticMethod);
/** @see IScriptEvaluator#setMethodName(String) */
void setMethodName(String methodName);
/** @see IScriptEvaluator#setMethodNames(String[]) */
void setMethodNames(String[] methodNames);
/** @see IScriptEvaluator#setThrownExceptions(Class[]) */
void setThrownExceptions(Class>[] thrownExceptions);
/** @see IScriptEvaluator#setThrownExceptions(Class[][]) */
void setThrownExceptions(Class>[][] thrownExceptions);
/**
* {@code Null} arguments is equivalent with {@code new Object[0]}.
*/
@Nullable Object evaluate(int idx, @Nullable Object... arguments) throws InvocationTargetException;
/**
* If the parameter and return types of the expression are known at compile time, then a "fast" expression evaluator
* can be instantiated through {@link #createFastEvaluator(String, Class, String[])}. Expression evaluation is
* faster than through {@link #evaluate(Object[])}, because it is not done through reflection but through direct
* method invocation.
*
* Example:
*
*
* public interface Foo {
* int bar(int a, int b);
* }
* ...
* ExpressionEvaluator ee = CompilerFactoryFactory.getDefaultCompilerFactory().newExpressionEvaluator();
*
* // Optionally configure the EE here...
* ee.{@link #setClassName(String) setClassName}("Bar");
* ee.{@link #setDefaultImports(String[]) setDefaultImports}(new String[] { "java.util.*" });
* ee.{@link #setExtendedClass(Class) setExtendedClass}(SomeOtherClass.class);
* ee.{@link #setParentClassLoader(ClassLoader) setParentClassLoader}(someClassLoader);
*
* // Optionally configure the EE here...
* Foo f = (Foo) ee.createFastEvaluator(
* "a + b", // expression to evaluate
* Foo.class, // interface that describes the expression's signature
* new String[] { "a", "b" } // the parameters' names
* );
* System.out.println("1 + 2 = " + f.bar(1, 2)); // Evaluate the expression
*
*
* All other configuration (implemented type, static method, return type, method name, parameter names and types,
* thrown exceptions) are predetermined by the interfaceToImplement.
*
*
* Notice: The {@code interfaceToImplement} must be accessible by the compiled class, i.e. either be declared
* {@code public}, or with {@code protected} or default access in the package of the compiled class (see {@link
* #setClassName(String)}.
*
*/
T
createFastEvaluator(String expression, Class extends T> interfaceToImplement, String... parameterNames)
throws CompileException;
/** @see #createFastEvaluator(String, Class, String[]) */
T
createFastEvaluator(Reader reader, Class extends T> interfaceToImplement, String... parameterNames)
throws CompileException, IOException;
/** @see IScriptEvaluator#getMethod() */
Method getMethod();
/** @see IScriptEvaluator#getMethod(int) */
Method getMethod(int idx);
/** @see IClassBodyEvaluator#getClazz() */
Class> getClazz();
/** @see IScriptEvaluator#getResult() */
Method[] getResult();
}