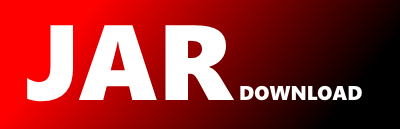
com.hazelcast.jet.sql.impl.opt.logical.DropLateItemsLogicalRel Maven / Gradle / Ivy
/*
* Copyright 2024 Hazelcast Inc.
*
* Licensed under the Hazelcast Community License (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://hazelcast.com/hazelcast-community-license
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.hazelcast.jet.sql.impl.opt.logical;
import com.hazelcast.shaded.org.apache.calcite.plan.RelOptCluster;
import com.hazelcast.shaded.org.apache.calcite.plan.RelOptCost;
import com.hazelcast.shaded.org.apache.calcite.plan.RelOptPlanner;
import com.hazelcast.shaded.org.apache.calcite.plan.RelTraitSet;
import com.hazelcast.shaded.org.apache.calcite.rel.RelNode;
import com.hazelcast.shaded.org.apache.calcite.rel.RelWriter;
import com.hazelcast.shaded.org.apache.calcite.rel.SingleRel;
import com.hazelcast.shaded.org.apache.calcite.rel.metadata.RelMetadataQuery;
import com.hazelcast.shaded.org.checkerframework.checker.nullness.qual.Nullable;
import java.util.List;
/**
* Relational operator that returns items from its input, with late items
* removed. A late item is an item whose timestamp is before the timestamp of
* the last watermark.
*/
public class DropLateItemsLogicalRel extends SingleRel implements LogicalRel {
private final int wmField;
public DropLateItemsLogicalRel(
RelOptCluster cluster,
RelTraitSet traitSet,
RelNode input,
int wmField
) {
super(cluster, traitSet, input);
this.wmField = wmField;
}
public int wmField() {
return wmField;
}
@Override
public @Nullable RelOptCost computeSelfCost(RelOptPlanner planner, RelMetadataQuery mq) {
return planner.getCostFactory().makeTinyCost();
}
@Override
public RelNode copy(RelTraitSet traitSet, List inputs) {
return new DropLateItemsLogicalRel(getCluster(), traitSet, sole(inputs), wmField);
}
public DropLateItemsLogicalRel copy(RelTraitSet traitSet, RelNode input, int wmField) {
return new DropLateItemsLogicalRel(getCluster(), traitSet, input, wmField);
}
@Override
public RelWriter explainTerms(RelWriter pw) {
return super.explainTerms(pw)
.item("traitSet", traitSet);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy