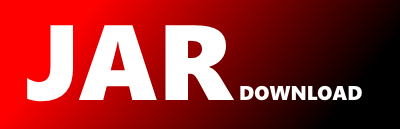
com.hazelcast.jet.impl.processor.PeekWrappedP Maven / Gradle / Ivy
/*
* Copyright (c) 2008-2023, Hazelcast, Inc. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.hazelcast.jet.impl.processor;
import com.hazelcast.function.FunctionEx;
import com.hazelcast.jet.core.Inbox;
import com.hazelcast.jet.core.Outbox;
import com.hazelcast.jet.core.Processor;
import com.hazelcast.jet.core.Watermark;
import com.hazelcast.jet.core.processor.DiagnosticProcessors;
import com.hazelcast.jet.impl.JetEvent;
import com.hazelcast.logging.ILogger;
import javax.annotation.Nonnull;
import java.util.BitSet;
import java.util.Iterator;
import java.util.NoSuchElementException;
import java.util.function.Predicate;
import java.util.stream.IntStream;
import static com.hazelcast.jet.Util.entry;
import static com.hazelcast.jet.impl.util.Util.toLocalTime;
/**
* Internal API, see {@link DiagnosticProcessors}.
*/
public final class PeekWrappedP extends ProcessorWrapper {
private final FunctionEx super T, ? extends CharSequence> toStringFn;
private final Predicate super T> shouldLogFn;
private final LoggingInbox loggingInbox;
private ILogger logger;
private final boolean peekInput;
private final boolean peekOutput;
private final boolean peekSnapshot;
private boolean peekedWatermarkLogged;
public PeekWrappedP(
@Nonnull Processor wrapped,
@Nonnull FunctionEx super T, ? extends CharSequence> toStringFn,
@Nonnull Predicate super T> shouldLogFn,
boolean peekInput, boolean peekOutput, boolean peekSnapshot
) {
super(wrapped);
this.toStringFn = toStringFn;
this.shouldLogFn = shouldLogFn;
this.peekInput = peekInput;
this.peekOutput = peekOutput;
this.peekSnapshot = peekSnapshot;
loggingInbox = peekInput ? new LoggingInbox() : null;
}
@Override
protected Outbox wrapOutbox(Outbox outbox) {
return new LoggingOutbox(outbox, peekOutput, peekSnapshot);
}
@Override
protected void initWrapper(@Nonnull Outbox outbox, @Nonnull Context context) {
logger = context.logger();
}
@Override
public void process(int ordinal, @Nonnull Inbox inbox) {
if (peekInput) {
loggingInbox.wrappedInbox = inbox;
loggingInbox.ordinal = ordinal;
super.process(ordinal, loggingInbox);
} else {
super.process(ordinal, inbox);
}
}
private void log(String prefix, @Nonnull T object) {
if (shouldLogFn.test(object)) {
logger.info(prefix + ": " + toStringFn.apply(object)
+ (object instanceof JetEvent
? " (eventTime=" + toLocalTime(((JetEvent) object).timestamp()) + ")"
: ""));
}
}
@Override
public boolean tryProcessWatermark(@Nonnull Watermark watermark) {
if (peekInput && !peekedWatermarkLogged) {
logger.info("Input coalesced WM: " + watermark);
peekedWatermarkLogged = true;
}
if (super.tryProcessWatermark(watermark)) {
peekedWatermarkLogged = false;
return true;
}
return false;
}
@Override
public boolean tryProcessWatermark(int ordinal, @Nonnull Watermark watermark) {
if (peekInput && !peekedWatermarkLogged) {
logger.info("Input edge WM, ordinal=" + ordinal + ", wm=" + watermark);
peekedWatermarkLogged = true;
}
if (super.tryProcessWatermark(ordinal, watermark)) {
peekedWatermarkLogged = false;
return true;
}
return false;
}
private class LoggingInbox implements Inbox {
private Inbox wrappedInbox;
private int ordinal;
@Override
public boolean isEmpty() {
return wrappedInbox.isEmpty();
}
@Override
public Object peek() {
return wrappedInbox.peek();
}
@Nonnull @Override
public Iterator
© 2015 - 2024 Weber Informatics LLC | Privacy Policy