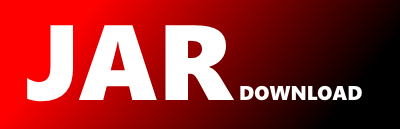
com.hcl.domino.admin.idvault.IdVault Maven / Gradle / Ivy
/*
* ==========================================================================
* Copyright (C) 2019-2022 HCL America, Inc. ( http://www.hcl.com/ )
* All rights reserved.
* ==========================================================================
* Licensed under the Apache License, Version 2.0 (the "License"). You may
* not use this file except in compliance with the License. You may obtain a
* copy of the License at .
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
* ==========================================================================
*/
package com.hcl.domino.admin.idvault;
import java.nio.file.Path;
import com.hcl.domino.DominoException;
import com.hcl.domino.data.Document;
/**
* Access to all id and cert operations
*/
public interface IdVault {
/**
* Callback interface to work with an opened ID
*
* @param computation result type
*/
@FunctionalInterface
public interface IDAccessCallback {
/**
* Implement this method to work with the passed user id. Do not store it
* anywhere, since it is disposed right after the method call!.
*
* @param id id
* @return optional computation result
*/
T accessId(UserId id);
}
public interface SyncResult {
String getVaultServer();
boolean isIdFoundInVault();
boolean isIdSyncDone();
}
/**
* This function changes the password in the specified ID file.
* You can use this function to change the password in a user's id, a server's
* id, or a certifier's id.
*
* Multiple passwords are not supported.
*
* @param idPath path to the ID file whose password should be changed
* @param oldPassword old password in the ID file. This parameter can only be
* NULL if there is no old password. If this parameter is set
* to "", then ERR_BSAFE_NULLPARAM is returned
* @param newPassword new password on the ID file. If this parameter is NULL,
* the password is cleared. If the specified ID file requires
* a password and this parameter is NULL, then
* ERR_BSAFE_PASSWORD_REQUIRED is returned. If this parameter
* is set to "", then ERR_BSAFE_NULLPARAM is returned. If the
* specified ID file is set for a minimum password length and
* this string contains less than that minimum, then
* ERR_REG_MINPSWDCHARS is returned.
*/
void changeIdPassword(Path idPath, String oldPassword, String newPassword);
/**
* The method tries to open the ID with the specified password. If the password
* is
* not correct, the method tries a {@link DominoException}
*
* @param idPath id path
* @param password password
* @throws DominoException e.g. ERR 6408 if password is incorrect
*/
void checkIdPassword(Path idPath, String password);
/**
* Will contact the server and locate a vault for userName
.
* Then extract the ID file from the vault and write it to
* idPath
.
*
* If successful returns with the vault server name.
*
* @param userName Name of user whose ID is being put into vault - either
* abbreviated or canonical format
* @param password Password to id file being uploaded to the vault
* @param idPath Path to where the download ID file should be created or
* overwritten
* @param serverName Name of server to contact
* @return the vault server name
* @throws DominoException in case of problems, e.g. ERR 22792 Wrong Password
*/
String extractUserIdFromVault(String userName, String password, Path idPath, String serverName);
/**
* Will contact the server and locate a vault for userName
.
* Then downloads the ID file from the vault and store it in memory.
*
* @param userName Name of user whose ID is being put into vault - either
* abbreviated or canonical format
* @param password Password to id file being uploaded to the vault
* @param serverName Name of server to contact
* @return the in-memory user id
* @throws DominoException in case of problems, e.g. ERR 22792 Wrong Password
*/
UserId getUserIdFromVault(String userName, String password, String serverName);
/**
* Will contact the server and retrieves the {@link UserId} associated with the
* provided
* implementation-specific token.
*
* @param token the token to use to retrieve the ID. The class of the token
* depends on the available
* vault provider implementations
* @param serverName the name of the server to contact
* @return the in-memory user ID
* @throws UnsupportedOperationException when no provider can be found to handle
* {@code token}
* @since 1.0.19
*/
UserId getUserIdWithToken(Object token, String serverName);
/**
* This function will extract the username from an ID file.
*
* @param idPath id path
* @return canonical username
*/
String getUsernameFromId(Path idPath);
/**
* Checks if the ID vault on the specified server contains an ID for a user
*
* @param userName user to check
* @param server server
* @return true if ID is in vault
*/
boolean isIdInVault(String userName, String server);
/**
* Opens an ID file and returns an in-memory handle for signing
* ({@link Document#sign(UserId, boolean)})
* and using note encrypting
* ({@link Document#copyAndEncrypt(UserId, java.util.Collection)} /
* {@link Document#decrypt(UserId)}).
*
* @param optional result type
* @param idPath id path on disk
* @param password id password
* @param callback callback code to access the opened ID; we automatically close
* the ID file when the callback invocation is done
* @return optional computation result
*/
T openUserIdFile(Path idPath, String password, IDAccessCallback callback);
/**
* Will open the ID file name provided, locate a vault server for user
* userName
,
* upload the ID file contents to the vault, then return with the vault server
* name.
*
* @param userName Name of user whose ID is being put into vault - either
* abbreviated or canonical format
* @param password Password to id file being uploaded to the vault
* @param idPath Path to where the download ID file should be created or
* overwritten
* @param serverName Name of server to contact
* @return the vault server name
* @throws DominoException in case of problems, e.g. ERR 22792 Wrong Password
*/
String putUserIdIntoVault(String userName, String password, Path idPath, String serverName);
/**
* Will locate a vault server for user userName
and
* upload the specified ID contents to the vault, then return with the vault
* server name.
*
* @param userName Name of user whose ID is being put into vault - either
* abbreviated or canonical format
* @param password Password to id file being uploaded to the vault
* @param userId user id
* @param serverName Name of server to contact
* @return the vault server name
* @throws DominoException in case of problems, e.g. ERR 22792 Wrong Password
*/
String putUserIdIntoVault(String userName, String password, UserId userId, String serverName);
/**
* Resets an ID password. This password is required by the user when they
* recover their ID file from the ID vault.
*
* @param server Name of server to contact to request the password reset.
* Can be NULL if executed from a program or agent on a
* server. Does NOT have to be a vault server. But must be
* running Domino 8.5 or later.
* @param userName Name of user to reset their vault id file password.
* @param password New password to set in the vault record for pUserName.
* @param downloadCount (max. 65535) If this user's effective policy setting
* document has "allow automatic ID downloads" set to no,
* then this parameter specifies how many downloads the
* user can now perform. If downloads are automatic this
* setting should be zero.
*/
void resetUserPasswordInVault(String server, String userName, String password, int downloadCount);
/**
* Will open the ID file name provided, locate a vault server, synch the ID file
* contents to the vault,
* then return the synched content. If successful the vault server name is
* returned.
*
* @param userName Name of user whose ID is being put into vault - either
* abbreviated or canonical format
* @param password Password to id file being uploaded to the vault
* @param idPath Path to where the download ID file should be created or
* overwritten
* @param serverName Name of server to contact
* @return sync result
*/
SyncResult syncUserIdWithVault(String userName, String password, Path idPath, String serverName);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy