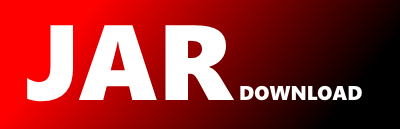
com.hcl.domino.data.DbQueryResult Maven / Gradle / Ivy
/*
* ==========================================================================
* Copyright (C) 2019-2022 HCL America, Inc. ( http://www.hcl.com/ )
* All rights reserved.
* ==========================================================================
* Licensed under the Apache License, Version 2.0 (the "License"). You may
* not use this file except in compliance with the License. You may obtain a
* copy of the License at .
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
* ==========================================================================
*/
package com.hcl.domino.data;
import java.util.Collection;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
import java.util.function.BiConsumer;
import java.util.stream.Stream;
import com.hcl.domino.data.CollectionSearchQuery.CollectionEntryProcessor;
import com.hcl.domino.misc.Loop;
public interface DbQueryResult> {
/**
* Build a result out of the collection entries
*
* @param result type
* @param skip paging offset
* @param count paging count
* @param processor builder code to produce the result
* @return result
*/
T build(int skip, int count, CollectionEntryProcessor processor);
/**
* Collect all {@link CollectionEntry} objects as list
*
* @return all entries
*/
default List collectEntries() {
return this.collectEntries(0, Integer.MAX_VALUE);
}
/**
* Collect all {@link CollectionEntry} objects as list
*
* @param skip paging offset
* @param count paging count
* @return list of collection entries
*/
List collectEntries(int skip, int count);
/**
* Collect all {@link CollectionEntry} objects in a {@link Collection}
*
* @param skip paging offset
* @param count paging count
* @param collection collection to add entries
*/
void collectEntries(int skip, int count, Collection collection);
/**
* Return the note ids of the search result as an ordered {@link Set}.
*
* Implementations are likely, but not guaranteed, to return a
* {@link LinkedHashSet}.
*
*
* @param skip paging offset
* @param count paging count
* @return set of note ids
*/
Set collectIds(int skip, int count);
/**
* Adds all note ids of the search result to a note ID collection, with special
* support
* for {@link IDTable}s
*
* @param skip paging offset
* @param count paging count
* @param idTable note ID collection
*/
void collectIds(int skip, int count, Collection idTable);
/**
* Dynamically computes virtual item values from the summary buffer data
*
* @param itemsAndFormulas map of item/formula pairs
* @return this search query
*/
CHAINTYPE computeValues(Map itemsAndFormulas);
/**
* Dynamically computes virtual item values from the summary buffer data
*
* @param itemsAndFormulas tuples with item name/formula; leave formula empty to
* read an existing document item, e.g. ["_created",
* "@Created", "Form", ""]
* @return this search query
*/
CHAINTYPE computeValues(String... itemsAndFormulas);
/**
* Iterates over each document in the search result
*
* @param skip paging offset
* @param count paging count
* @param consumer consumer to receive document
*/
void forEachDocument(int skip, int count, BiConsumer consumer);
Stream getDocuments();
/**
* Returns an {@link IDTable} of documents matching the search.
*
* @return an {@link Optional} describing the documents matched by the search,
* or
* an empty one if the search did not request a document collection
*/
Optional getNoteIds();
/**
* Returns the {@link Database} that was used to run the query
*
* @return database
*/
Database getParentDatabase();
/**
* Returns the total number of results
*
* @return total
*/
int size();
/**
* Sorts/filters the note ids of the search result like the specified
* {@link DominoCollection}.
* Note ids that are not part of the collection will be ignored.
*
* For maximum performance, please make sure to disable these settings in the view:
*
* - show response documents in a hierarchy
* - for the sort column: show multiple values as separate entries
*
* Depending on the size/structure of the view, disabling these settings can result
* in a performance improvement from 7000ms down to 10ms.
*
* @param collection collection
* @return this search query
*/
CHAINTYPE sort(DominoCollection collection);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy