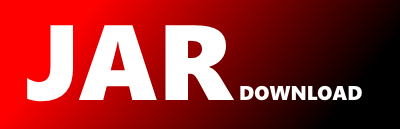
com.hcl.domino.data.Formula Maven / Gradle / Ivy
/*
* ==========================================================================
* Copyright (C) 2019-2022 HCL America, Inc. ( http://www.hcl.com/ )
* All rights reserved.
* ==========================================================================
* Licensed under the Apache License, Version 2.0 (the "License"). You may
* not use this file except in compliance with the License. You may obtain a
* copy of the License at .
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
* ==========================================================================
*/
package com.hcl.domino.data;
import java.util.Collection;
import java.util.List;
import com.hcl.domino.formula.FormulaCompiler;
import com.hcl.domino.misc.INumberEnum;
import com.hcl.domino.misc.NotesConstants;
/**
* A compiled Domino formula that can be evaluated standalone or
* on one or multiple documents.
*
* The implementation supports more than the usual 64K of return value, e.g. to use
* @DBColumn
to read the column values in views with many entries.
*/
public interface Formula {
public enum Disallow implements INumberEnum{
/** Setting of environment variables */
SETENVIRONMENT(NotesConstants.COMPUTE_CAPABILITY_SETENVIRONMENT),
UICOMMANDS(NotesConstants.COMPUTE_CAPABILITY_UICOMMANDS),
/** FIELD Foo :=
*/
ASSIGN(NotesConstants.COMPUTE_CAPABILITY_ASSIGN),
/** @SetDocField
, @DocMark
*/
SIDEEFFECTS(NotesConstants.COMPUTE_CAPABILITY_SIDEEFFECTS),
/** Any compute extension. */
EXTENSION(NotesConstants.COMPUTE_CAPABILITY_EXTENSION),
/** Any compute extension with side-effects */
UNSAFE_EXTENSION(NotesConstants.COMPUTE_CAPABILITY_UNSAFE_EXTENSION),
/** Built-in compute extensions */
FALLBACK_EXT(NotesConstants.COMPUTE_CAPABILITY_FALLBACK_EXT),
/** Unsafe is any @func
that creates/modifies anything (i.e. not "read only") */
UNSAFE(NotesConstants.COMPUTE_CAPABILITY_UNSAFE);
private final int m_value;
private Disallow(int value) {
m_value = value;
}
@Override
public Integer getValue() {
return m_value;
}
@Override
public long getLongValue() {
return m_value;
}
}
/**
* Prevents the execution of unsafe formula operations.
*
* @param actions disallowed actions
* @return this instance
* @since 1.10.11
*/
Formula disallow(Collection actions);
/**
* Prevents the execution of unsafe formula operations.
*
* @param action disallowed action
* @return this instance
* @since 1.10.11
*/
Formula disallow(Disallow action);
/**
* Checks whether a formula operation is disallowed
*
* @param action action
* @return true if disallowed
* @since 1.10.11
*/
boolean isDisallowed(Disallow action);
/**
* Returns a list of all registered (public) formula functions. Use {@link #getFunctionParameters(String)}
* to get a list of function parameters.
*
* @return functions, e.g. "@Left("
*/
public static List getAllFormulaFunction() {
return FormulaCompiler.get().getAllFormulaFunctions();
}
/**
* Returns a list of all registered (public) formula commands. Use {@link #getFunctionParameters(String)}
* to get a list of command parameters.
*
* @return commands, e.g. "MailSend"
*/
public static List getAllFormulaCommands() {
return FormulaCompiler.get().getAllFormulaCommands();
}
/**
* Scan through the function table or keyword table to find parameters of an @ function or an @ command.
*
* @param atFunctionName name returned by {@link #getAllFormulaFunction()} or {@link #getAllFormulaCommands()}, e.g. "@Left("
* @return function parameters, e.g. ["stringToSearch; numberOfChars)", "stringToSearch; subString)"] for the function "@Left("
*/
public static List getFunctionParameters(String atFunctionName) {
return FormulaCompiler.get().getFunctionParameters(atFunctionName);
}
/**
* Formula computation result
*/
public interface FormulaExecutionResult {
/**
* Returns the result
*
* @return result, e.g. List with String or {@link DominoDateTime}
*/
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy