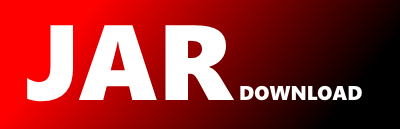
com.hcl.domino.data.Item Maven / Gradle / Ivy
/*
* ==========================================================================
* Copyright (C) 2019-2022 HCL America, Inc. ( http://www.hcl.com/ )
* All rights reserved.
* ==========================================================================
* Licensed under the Apache License, Version 2.0 (the "License"). You may
* not use this file except in compliance with the License. You may obtain a
* copy of the License at .
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
* ==========================================================================
*/
package com.hcl.domino.data;
import java.time.LocalDate;
import java.time.LocalTime;
import java.time.OffsetDateTime;
import java.time.temporal.TemporalAccessor;
import java.util.List;
import java.util.Optional;
import java.util.Set;
import com.hcl.domino.mime.MimeEntity;
import com.hcl.domino.misc.INumberEnum;
import com.hcl.domino.misc.NotesConstants;
import com.hcl.domino.richtext.RichTextRecordList;
import com.hcl.domino.richtext.records.RecordType;
import com.hcl.domino.richtext.records.RichTextRecord;
/**
* Carefull attention for date/time and the new Java Stuff and JNA TimeDate
*
* @author t.b.d
* @since 0.5.0
*/
public interface Item extends IAdaptable {
/**
* Enum for the field flags for an Item
*/
public enum ItemFlag implements INumberEnum {
SUMMARY(NotesConstants.ITEM_SUMMARY),
NAMES(NotesConstants.ITEM_NAMES),
READERS(NotesConstants.ITEM_READERS),
READWRITERS(NotesConstants.ITEM_READWRITERS),
PROTECTED(NotesConstants.ITEM_PROTECTED),
ENCRYPTED(0x0002),
SIGNED(NotesConstants.ITEM_SIGN),
/** @since 1.27.0 */
SEALED(NotesConstants.ITEM_SEAL),
/** @since 1.27.0 */
PLACEHOLDER(NotesConstants.ITEM_PLACEHOLDER),
/**
* Special flag to keep \n in string item values instead of replacing them with
* \0
* (e.g. required when writing $$FormScript in design elements)
*/
KEEPLINEBREAKS(0x8000000);
private final Integer m_val;
ItemFlag(final Integer val) {
this.m_val = val;
}
ItemFlag(final short val) {
this.m_val = Short.toUnsignedInt(val);
}
@Override
public long getLongValue() {
return this.m_val & 0xffff;
}
@Override
public Integer getValue() {
return this.m_val;
}
}
/**
* This function converts the input {@link ItemDataType#TYPE_RFC822_TEXT} item
* in an open document
* to its pre-V5 equivalent; i.e. to {@link ItemDataType#TYPE_TEXT},
* {@link ItemDataType#TYPE_TEXT_LIST},
* or {@link ItemDataType#TYPE_TIME}.
*
* For example, we convert the PostedDate {@link ItemDataType#TYPE_RFC822_TEXT}
* item to a
* {@link ItemDataType#TYPE_TIME} item.
*
* It does not update the Domino database; to update the database, call
* {@link Document#save()}.
*
* @return this item instance (for chaining)
*/
Item convertRFC822TextItem();
/**
* Copies this item to a new document, retaining the current name.
* TODO: If overwrite is false, does it create a duplicate item or abort?
*
* @param doc target document for the new item
* @param overwrite true to replace any existing item with the same name
*/
void copyToDocument(Document doc, boolean overwrite);
/**
* Copies this item to a new document, with a new Item name.
* TODO: If overwrite is false, does it create a duplicate item or abort?
*
* @param doc target document for the new item
* @param newItemName new Item name to save as
* @param overwrite true to replace any existing item with the same name
*/
void copyToDocument(Document doc, String newItemName, boolean overwrite);
/**
* Returns the item value converted to the specified data type.
*
* We currently support the following value types out of the box:
*
* - {@link String}
* - {@link Integer}
* - {@link Long}
* - {@link Double}
* - {@link DominoDateTime}
* - {@link LocalDate}
* - {@link LocalTime}
* - {@link OffsetDateTime}
* - {@link TemporalAccessor} (returned as {@link DominoDateTime})
*
*
* Additional value types are supported by implementing and registering
* {@link DocumentValueConverter} as Java services.
*
* @param type of return value
* @param valueType class of return value
* @param defaultValue default value to be returned of object property is not
* set
* @return return value
* @throws IllegalArgumentException if the specified value type is unsupported
*/
T get(Class valueType, T defaultValue);
/**
* Returns a list of item values converted to the specified data type.
*
* We currently support the following value types out of the box:
*
* - {@link String}
* - {@link Integer}
* - {@link Long}
* - {@link Double}
* - {@link DominoDateTime}
* - {@link LocalDate}
* - {@link LocalTime}
* - {@link OffsetDateTime}
* - {@link TemporalAccessor} (returned as {@link DominoDateTime})
*
*
* Additional value types are supported by implementing and registering
* {@link DocumentValueConverter} as Java services.
*
* @param type of return value
* @param valueType class of return value
* @param defaultValue default value to be returned of object property is not
* set
* @return value list
* @throws IllegalArgumentException if the specified value type is unsupported
*/
List getAsList(Class valueType, List defaultValue);
/**
* Retrieves the item value as text.
*
* This differs from calling {@link #get} with `String.class` as the type in
* that it uses an internal Domino routine to convert values to text.
*
*
* It also differs from {@link Document#getAsText(String, char)} in that
* it can be used on individual items with duplicate names within a
* document.
*
* @param separator a separator character to use when the item is multi-value
* @return the item value as a string
* @since 1.28.0
*/
String getAsText(char separator);
/**
* Gets all the field flags corresponding to the Item
*
* @return field flags applied, e.g. {SUMMARY,READERS}
*/
Set getFlags();
/**
* Retrieves the name of the file containing the item's content, if
* the item is a MIME part and stores its content in a separate
* file item.
*
* @return an {@link Optional} describing the file attachment name
* containing the item value if the item is a MIME part and
* stores its content in a separate file item, or an empty
* one otherwise
* @since 1.26.0
*/
Optional getMimeFileName();
/**
* Retrieves the item content as a MIME entity, if this is a MIME item.
*
* @return an {@link Optional} describing a {@link MimeEntity} for the item
* content, or
* an empty one if this is not a MIME item
*/
Optional getMimeEntity();
/**
* Gets the field name. Fields beginning "$" are, by convention, system fields
*
* @return the field name as a String
*/
String getName();
/**
* Extracts the key elements available in the Notes Client Document Properties
* window,
* so they can be made available in a serializable format
*
* @return a {@link DocumentProperties} object representing the document's meta
* properties
*/
DocumentProperties getProperties();
/**
* Incremental integer for the nth save this Item was last updated.
* Cross-reference with the $Revisions field for the date and time it was saved.
* Be aware that for very frequently updating documents, the integer will cycle
* back to 1
*
* @return integer corresponding to the nth time the document was saved
*/
int getSequenceNumber();
/**
* Gets the data type for a field, converting it to the more readable enum
*
* @return enum corresponding to the internal int value for the data type,
* or {@link ItemDataType#TYPE_INVALID_OR_UNKNOWN} if it is unknown
*/
ItemDataType getType();
/**
* Gets the data type for a field, as the internal int
*
* @return internal int value corresponding to the data type
*/
int getTypeValue();
/**
* Decodes the item value(s). The data is always returned as a list even though
* the list may contain only one element (e.g. for
* {@link ItemDataType#TYPE_TEXT}.
*
* The following data types are currently supported:
*
* - {@link ItemDataType#TYPE_TEXT} - List with String object
* - {@link ItemDataType#TYPE_TEXT_LIST} - List of String objects
* - {@link ItemDataType#TYPE_NUMBER} - List with Double object
* - {@link ItemDataType#TYPE_NUMBER_RANGE} - List of Double objects
* - {@link ItemDataType#TYPE_TIME} - List with Calendar object
* - {@link ItemDataType#TYPE_TIME_RANGE} - List of Calendar objects
* - {@link ItemDataType#TYPE_OBJECT} with the subtype Attachment (e.g. $File
* items) - List with {@link Attachment} object
* - {@link ItemDataType#TYPE_NOTEREF_LIST} - List with one UNID
* - {@link ItemDataType#TYPE_UNAVAILABLE} - returns an empty list
*
*
* @return a {@link List} of objects representing the item value
*/
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy